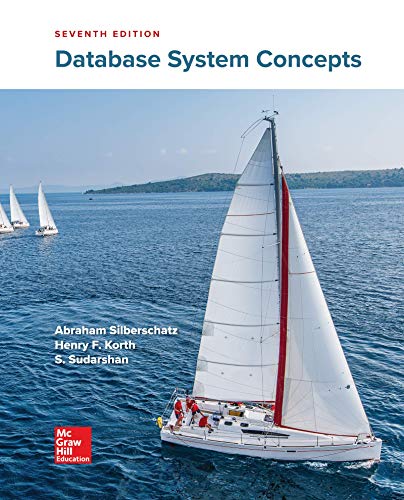
USING C++
PLEASE CREATE THREE FILES!
- IntCollection.h (or IntCollection.hpp): class definition
- IntCollection.cpp: class implementation
- main.cpp, including sample output and your answer to question 6 at the bottom. : main program to run the code
The following code creates an IntCollection object named ‘c’. It adds seven integers to ‘c’, then uses a for loop to display the seven integers:
int main() { IntCollection c; c.add(45); c.add(-210); c.add(77); c.add(2); c.add(-21); c.add(42); c.add(7); for (int i = 0; i < c.getSize(); i++) { cout << c.get(i) << endl; } }
For this assignment you will add a copy constructor, a destructor, and three overloaded operators to the IntCollection class. In the design diagram below, the black member functions represent code that has already been implemented. You will be implementing the green items. Each item that you will be adding to the class is described below the diagram.
private: int size // the number of ints currently stored in the int collection int capacity // total number of elements available in data array int* data // a pointer to the dynamically allocated data array void addCapacity() // private function to allocate more memory if necessary |
public: IntCollection() ~IntCollection() IntCollection(const IntCollection &c) void add(int value) int get(int index) int getSize() IntCollection& operator=(const IntCollection &c) bool operator==(const IntCollection &c) IntCollection& operator<<(int value) |
1. The Copy Constructor. The copy constructor should perform a deep copy of the argument object, i.e. it should construct an IntCollection with the same size and capacity as the argument, with its own complete copy of the argument's data array.
2. The Assignment operator (=). The assignment operator should also perform a deep copy of the argument object. It must return itself (or more efficiently, a reference to itself) in order to support multiple assignments on the same line, e.g. a = b = c. If you implement your assignment operator first it could be used in the copy constructor, but this is not a requirement.
3. The Is Equals operator (==). The "is equals" operator should return true if the argument object has the same size as the receiving object, and the values in both objects’ data arrays are identical.
4. The insertion operator (<<). The insertion operator should add the int parameter into the receiving IntCollection. The functionality is the same as the add() function, i.e. add ints to the collection. Note, however, that this function must return a reference to itself in order to support multiple insertions on the same line, e.g. c << 45 << -210. Unlike the assignment operator, this return must be done by reference, because each insertion actually modifies the IntCollection object, and insertion is done from left to right.
5. The destructor. Function add() calls addCapacity() to allocate memory when it needs more room. Nowhere in this program is the memory deallocated with delete [], which means we have a memory leak! Add a destructor which correctly handles this.
6. addCapacity. Note that addCapacity() is a private member function. What happens if you try to call it from outside the class, i.e. by adding the line below to main()?
c.addCapacity();

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- Music App 1. practise file I/O and exception handling; 2. practise String processing. Aim: Task: Create a Java application that transcripts a simple song score sheet. The program asks user for filename of a text file that contains a simple song score sheet in this format: Sample song score sheet 1, song1.txt: #simple song with assumed time signature = 4 d1mls202s 1mld2o2 d1rimlfls 301s1f1m1rld4 Lines beginning with a hash #' is a comment line. A song contains one or more lines of musical notes, d, r, m, f, s, l, tand also o meaning "Off" or rest. Each single character note is followed by a single digit integer in [1 – 4], denoting the duration of the note in number of beats. After transcription, the program outputs the melody on the screen as well as to another text file: Melody output 1, on screen AND in melody_song1.txt: #simple song with assumed time signature Do Me So- Off- So Me Do Re Me Fa| So-- Off = 4 Do- Off- | So Fa Me Re Do--- | The default time signature is 4 beats per…arrow_forwardLAB ASSIGNMENTS IMPORTANT: you need to complete the PDP thinking process for each program. The example program downloads, opens and reads a file, hosted at this address: https://www. w3.org/TR/PNG/iso_8859-1.txt web_scraping 1.py - write a program to download a .txt file from online, open and read the file, and then display the output. Note: you do NOT have to display the whole file or write to a .txt file (optional). You need to use a different .txt file. 120 The following are the graphical (non-control) characters defined by ISO 8859-1 (1987). Descriptions in words aren't all that helpful, but they're the best we can do in text. A graphics file illustrating the character set should be available from the same archive as this file. Step 1 - find a .txt file online to work with. One we have not used previously. Hex Description Hex Description 20 SPACE 21 EXCLAMATION MARK Al INVERTED EXCLAMATION MARK 22 QUOTATION MARK A2 CENT SIGN 23 NUMBER SIGN АЗ POUND SIGN 24 DOLLAR SIGN A4 CURRENCY…arrow_forward9b_act1. Please help me answer this in python programming.arrow_forward
- C++ Visual Studio 2019 Write a program that asks the user for the name of a file. The program should display the contents of the file on the screen. Each line of screen output should be preceded with a line number, followed by a colon. The line numbering should start at 1. Here is an example: George Rolland 127 Academy Street Brasstown, NC 28706 If the file's contents won't fit on a single screen, the program should display 24 lines of output at a time, and then pause. Each time the program pauses, it should wait for the user to strike a key before the next 24 lines are displayed. Note: Save text below as the file to use and name it forChap12.txt file for this assignment. The line numbers should NOT be reset for each screen. Text: No one is unaware of the name of that famous English shipowner, Cunard. In 1840 this shrewd industrialist founded a postal service between Liverpool and Halifax, featuring three wooden ships with 400-horsepower paddle wheels and a burden of 1,162 metric…arrow_forwardData models and data structures should not be confused with one another.arrow_forwardJAVA PPROGRAM ASAP Hypergrade does not like this program because it says 2 out of 7 passed and take out the extra \n from the program. Please Modify and change this program ASAP BECAUSE IT IS HOMEWORK ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Also, for test cases 1-4 it wants only to input Please enter the file name or type QUIT to exit: then input the file and display the Total number of words. For test cases 5 and 7 it wants to only to input Please enter the file name or type QUIT to exit then input input5.txt and then display File: input5.txt does not exist.\n then display Please enter the file name again or type QUIT to exit:\n then type input1.txt to display the total number of words or type quit to exit the program. For test case 6 it wants only to Please enter the file name again or type QUIT to exit:\n then type quit to exit the porgram. import java.io.File;import java.io.FileNotFoundException;import…arrow_forward
- Word Puzzle GameIn this assignment is only for individual. You are going to decode the scrambled word into correct orderin this game. One round of play is as follows:1. Computer reads a text file named words.txt2. Computer randomly picks one word in the file and randomly scrambles characters in the wordmany times to hide the word3. Computer displays the scrambled word to user with indexes and gives the user options to1. Swap two letters in word based on index given2. Solve the puzzle directly3. Quit the game4. If a player chooses to swap letters, the computer reads two indexes and swaps the letters. Thegame resume to step 3 with the newly guessed word. If the resulted word after swapping is thesecret word, the game is over.5. If a user chooses to solve directly, computer prompts the user to enter the guessed word. If it’scorrect, the game is over, otherwise goes to step 3.6. After game is over, display how many times the player has tried to solve the puzzle.The following is sample…arrow_forwardC++ programming!!!! Abstract You are given a file containing many words. Read in all of the words from the file and insert each word into a searchable ADT. Give the user a looping menu allowing them to search for words in the word list. Searches must be be found in O(1) or constant time. Your code must time the search operations, and display the time to the user. For this assignment, you may use the provided word list or another word list with more than 150,000 words. Include this file with your submission. You must design and implement the following classes: Word: This class holds a single word as a string. Basic getters and setters are required. HashTable: This class represents the hash table for storing many words. Main: This is the driver class. The driver: Create a Hash Table Read in all of the words from a text file into the Hash Table Provide a looping menu for the user to perform searches. Time all searches and display the execution time to the user For each…arrow_forwardRange for loop should be used even the block code needs to access index. Group of answer choices True Falsearrow_forward
- JAVA PROGRAM Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage double_input1.txt double_input2.txt Test Case 1 Please enter the file name: \ndouble_input1.txtENTERTotal: -5,748.583\nAverage: -57.486\n Test Case 2 Please enter the file name: \ndouble_input2.txtENTERTotal: 112,546.485\nAverage: 56.273\n Test Case 3 Please enter the file name: \ndouble_input3.txtENTERFile 'double_input3.txt' does not exist.\nPlease enter the file name again: \ndouble_input1.txtENTERTotal: -5,748.583\nAverage: -57.486\narrow_forward*Coding language is Python Write a program that opens the productsales.txt file and reads the sales into a list. The program should output the following information, in this order: The total of all sales in the list The average of all sales in the list The lowest sale in the list The highest sale in the list NOTE: Your program should include at least one user-defined function, in addition to the main function. 4147 1594 2235 8433 10000 129 5555 7030 9764 7465 1111 4444 8954 2243 2895 1436 4978 5486 1436 9846 4789 8456 2497 2280 6375arrow_forwardJAVA PROGRAM ASAP Please CREATE A program ASAP BECAUSE IT IS HOMEWORK ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #14. Word Separator (modified *** Read carefully ***) Write a program that prompts the user to enter a file name, then opens the file in text mode and reads it line by line. While entering the file name, the program should allow the user to type quit to exit the program. If the file with a given name does not exist, then display a message and allow the user to re-enter the file name. The file can contain one or more sentences. The program should accept as input each sentence in which all the words are run together, but the first character of each word is uppercase. Convert sentences to strings in which the words are separated by spaces and only the first word starts with an uppercase letter. For example, the string StopAndSmellTheRoses.” would be converted to “Stop and smell the roses.”…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
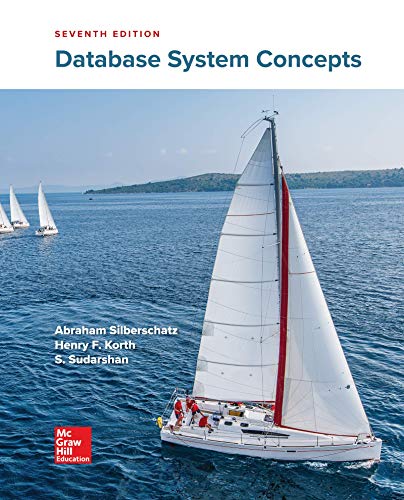
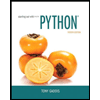
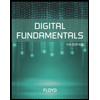
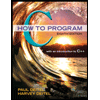
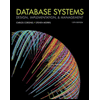
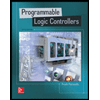