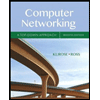
Is there still an array in the program? If so where is it?
//PSEUDOCODE
//Write a program that prompts the user to enter a full name consisting of three names
//first
//middle
//last
// into one String variable
//Then use methods of class String to:
//print the number of characters in the full name, excluding spaces
//print just the middle name and the number of characters in it
//print the three initials of the name
//print the last name in all lower case
//print the full name in the usual alphabetical format (Last, First Middle) and proper case
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter full name:");
String fullName = sc.nextLine();
System.out.println("\nNumber of Charatcers in your name " + fullName + "':" + fullName.replace("","").length());
String[] subNames = fullName.split(" ");
System.out.println("\nMiddle Name: " + subNames[1]);
System.out.println("Total characters: " + subNames[1].length());
System.out.println("Initials: " + subNames[0].charAt(0) + " " + subNames[1].charAt(0) + " " + subNames[2].charAt(0));
System.out.println("Last name is all lowercase: " + subNames[2].toLowerCase());
System.out.println("Full name: " + subNames[2] + ", " + subNames[0] + " " + subNames[1]);
sc.close();
}
}

Step by stepSolved in 2 steps with 1 images

Okay, in my assignment I am not allowed to use array in the program. How do I replace what I have without the array?
Okay, in my assignment I am not allowed to use array in the program. How do I replace what I have without the array?
- Rest of code in image / This is a bad programming style since it is using goto. // This is an spagetti code and not working.// Use function to display menu, and display game rules,// Use different color for text display.// fix it so it works any way you like./*HANDLE screen = GetStdHandle(STD_OUTPUT_HANDLE); // Write 16 lines in 16 different colors. for (int color = 0; color < 16; color++) { SetConsoleTextAttribute (screen, color); cout << " Hello World!" << endl; Sleep(400); // Pause between lines to watch them appear } // Restore the normal text color) SetConsoleTextAttribute(screen, 7);*/#include <iostream>#include <windows.h>using namespace std;int main(){ //textbackground(WHITE); //textcolor(RED); system("cls"); char ch, a[20], ch2; int num = 100, rnum, guess, count, ch1, c = 0; cout << "**********************************************************"<<endl; cout << "*…arrow_forwardComplete the Funnyville High School registration program where user is prompted for her full name and the program generates email id and temporary password. Sample run: generate_EmailID: This function takes two arguments: the user’s first name and last name and creates and returns the email id as a string by using these rules: The email id is all lower case. email id is of the form “last.first@fhs.edu”. e.g. For "John Doe" it will be "doe.john@fhs.edu". See sample runs above. generate_Password: This function takes two arguments: the user’s first name and last name and generates and returns a temporary password as a string by using these rules. Assume that user's first and last names have at least 2 letters. The temporary password starts with the first 2 letters of the first name, made lower case. followed by a number which is the sum of the lengths of the first and last name (For example this number will be 7 for "John Doe" since length of "John" is 4 and length of "Doe" is…arrow_forwardJAVA PPROGRAM ASAP Please create this program ASAP BECAUSE IT IS MY LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #2. Word Counter (page 608) Write a method that accepts a String object as an argument and returns the number of words it contains. For instance, if the argument is “Four score and seven years ago” the method should return the number 6. Demonstrate the method in a program that asks the user to input a string and then passes it to the method. The number of words in the string should be displayed on the screen. Test Case 1 Please enter a string or type QUIT to exit:\nHello World!ENTERThere are 2 words in that string.\nPlease enter a string or type QUIT to exit:\nI have a dream.ENTERThere are 4 words in that string.\nPlease enter a string or type QUIT to exit:\nJava is great.ENTERThere are 3 words in that string.\nPlease enter a string or type QUIT to exit:\nquitENTERarrow_forward
- JAVA PROGRAM ASAP Please CREATE A program ASAP BECAUSE IT IS LAB ASSIGNMENT so it passes all the test cases. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #16. Morse Code Converter Morse code is a code where each letter of the English alphabet, each digit, and various punctuation characters are represented by a series of dots and dashes. Write a program that asks the user to enter a string, and then converts that string to Morse code and prints on the screen. Use hyphens for dashes and periods for dots. The Morse code table is given in a text file morse.txt. When printing morse code, display eight codes on each line except the last line. Codes should be separated from each other with one space. There should be no extra spaces at the beginning and at the end of the output. Uppercase and lowercase letters are translated the same way. Morse.txt 0 -----1 .----2 ..---3 ...--4 ....-5 .....6 -....7 --...8 ---..9 ----.,…arrow_forwardVariables Primitive Write a java program that asks the user to enter 3 integers. Add the integers and display “The total is “ the result Reference Write a java program that asks the user for their first name and last name. Display “Your full name is “ the first name and last name Arrays One-dimensional Write a java program that creates an array of integers called numbers and contains five elements. Then use a for loop to print them out using println(). Two-dimensional submit your code for the total of rows and total of columns herearrow_forwardLearning Objectives: Python Programming Use loop over a stringReplace characters within a stringFunction (declaration and call)Write code in modular formInstructions Create a function only_chars that takes the line of text and returns the text line excluding the spaces, periods, exclamation points, or commas. Create a program that will read the input line, use the function, and then print the line, without spaces, periods, exclamation marks, or commas and their length. Ex: If the input is: Listen, Mr. Jones, calm down. the output is: ListenMrJonescalmdown21Do not forget if __name__ == "__main__" section!arrow_forward
- Python question Application: Python Fragment Formulation (Q1 – Q4) In this group of questions you are asked to produce short pieces of Python code. When you are asked to "write a Python expression" to complete a task, you can either give the expression in one line or break down the task into several lines. The last expression you write must represent the required task. Question 1 (Reduce parentheses) Give an equivalent version of this expression by removing as many redundant parentheses as possible, without expanding the brackets or simplifying. (x**(2**y))+(y*((z+x)**3)) Question 2 (Translate arithmetic concept into Python) You are given a list of numbers, named numbers, containing 3 integers. Write a python expression (one line) that evaluates to True if and only if the product of any two numbers in the given list is greater than the sum of all three numbers. Note: the product of two numbers, x and y is x*y. Question 3 (List/table access) You are given a table,…arrow_forward//write code that loops through the two variables returns an array ['2 is zwei', '3 is drei', '4 is vier', '5 is fünf', '6 is sechs']// example:// return: ['2 is zwei', '3 is drei', '4 is vier', '5 is fünf', '6 is sechs']// hint: use Array.forEach, Array.map or a for loopfunction germanNumbers(){ const cardinalNumbers = [2,3,4,5,6]; const germanNumbers = ['zwei', 'drei', 'vier', 'fünf', 'sechs']; } // write code that returns an array of ONLY prime numbers that are in the array numbers// example: // return [2, 3, 5, 7, 11, 13]// hint use: Array.filterfunction returnPrimeNumbers(){ const numbers = [1,2,3,4,5,6,7,8,9,10,11,12,13,14,15]; }arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
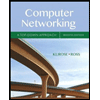
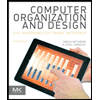
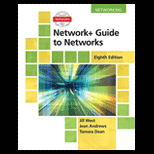
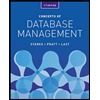
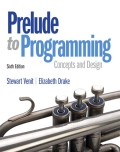
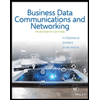