It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed) #include "node.h" #include using namespace std; class BSTree { node* root; int size; node* create_node(int num, node* parent) { node* n = (node*) malloc( sizeof(node) ); n->element = num; n->parent = parent; n->right = NULL; n->left = NULL; return n; } bool search(node* curr, int num) { if (curr == NULL) { return false; } if (num == curr->element) { return true; } if (num < curr->element) { return search(curr->left, num); } return search(curr->right, num); } node* search_node(node* curr, int num) { if (num == curr->element) { return curr; } if (num < curr->element) { if (curr->left != NULL) { return search_node(curr->left, num); } return curr; } if (curr->right != NULL) { return search_node(curr->right, num); } return curr; } node* findNewRoot(node* curr) { if(curr->left == NULL) { return curr; } return findNewRoot(curr->left); } public: BSTree() { root = NULL; size = 0; } bool remove(int num) { bool isPresent = search(num); if(isPresent){ bool rem = false; int numOfChild; node* realRoot = search_node(root,num); if(realRoot->left == NULL && realRoot->right == NULL) { numOfChild = 0; } else if((realRoot->left != NULL && realRoot->right == NULL) || (realRoot->left == NULL && realRoot->right != NULL) ) { numOfChild = 1; } else if(realRoot->left != NULL && realRoot->right != NULL) { numOfChild = 2; } if(numOfChild == 0) { bool leadRoot = false; if(realRoot == root) { leadRoot = true; } if(leadRoot) { free(realRoot); size--; BSTree(); rem = true; return rem; } if(realRoot->right == NULL && realRoot->left == NULL) { bool toRight; if(realRoot->element > realRoot->parent->element) { toRight = true; } else { toRight = false; } if(toRight) { realRoot->parent->right = NULL; } else { realRoot->parent->left = NULL; } rem = true; free(realRoot); size--; return rem; } } if(numOfChild == 1) { bool leadRoot = false; if(realRoot == root) { leadRoot = true; } if(realRoot->right != NULL && realRoot->left == NULL) { bool toRight; if(leadRoot) { root = realRoot->right; root->parent = NULL; rem = true; free(realRoot); return rem; } if(realRoot->element > realRoot->parent->element) { toRight = true; } else { toRight = false; } if(toRight) { realRoot->parent->right = realRoot->right; realRoot->right->parent = realRoot->parent; } else { realRoot->parent->left = realRoot->right; realRoot->right->parent = realRoot->parent; } rem = true; free(realRoot); size--; return rem; } if(realRoot->left != NULL && realRoot->right == NULL) { bool toRight; if(leadRoot) { root = realRoot->left; root->parent = NULL; rem = true; free(realRoot); return rem; } if(realRoot->element > realRoot->parent->element) { toRight = true; } else { toRight = false; } if(toRight) { realRoot->parent->right = realRoot->left; realRoot->left->parent = realRoot->parent; } else { realRoot->parent->left = realRoot->left; realRoot->left->parent = realRoot->parent; } rem = true; free(realRoot); size--; return rem; } } if(numOfChild == 2) { node* temp; temp = findNewRoot(realRoot->right); if(realRoot->right->element == temp->element) { bool uniqueRight = false; if(temp->right != NULL) { uniqueRight = true; } if(uniqueRight) { realRoot->right = temp->right; temp->right->parent = temp->parent; } else { realRoot->right = NULL; } rem = true; realRoot->element = temp->element; free(temp); size--; return rem; } bool hasRight = false; if(temp->right != NULL) { hasRight = true; } if(hasRight) { temp->parent->left = temp->right; temp->right->parent = temp->parent; } else { temp->parent->left = NULL; } rem = true; realRoot->element = temp->element; free(temp); size--; } return rem; } return isPresent; } bool isEmpty() { // TODO isEmpty return size == 0; } void print_preorder(node* curr) { // TODO preorder traversal cout << curr->element << " "; if(curr->left != NULL) { print_preorder(curr->left); } if(curr->right != NULL) { print_preorder(curr->right); } } void print_postorder(node* curr) { // TODO postorder traversal if(curr->left != NULL) { print_postorder(curr->left); } if(curr->right != NULL) { print_postorder(curr->right); } cout << curr->element << " "; }
It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed) #include "node.h" #include using namespace std; class BSTree { node* root; int size; node* create_node(int num, node* parent) { node* n = (node*) malloc( sizeof(node) ); n->element = num; n->parent = parent; n->right = NULL; n->left = NULL; return n; } bool search(node* curr, int num) { if (curr == NULL) { return false; } if (num == curr->element) { return true; } if (num < curr->element) { return search(curr->left, num); } return search(curr->right, num); } node* search_node(node* curr, int num) { if (num == curr->element) { return curr; } if (num < curr->element) { if (curr->left != NULL) { return search_node(curr->left, num); } return curr; } if (curr->right != NULL) { return search_node(curr->right, num); } return curr; } node* findNewRoot(node* curr) { if(curr->left == NULL) { return curr; } return findNewRoot(curr->left); } public: BSTree() { root = NULL; size = 0; } bool remove(int num) { bool isPresent = search(num); if(isPresent){ bool rem = false; int numOfChild; node* realRoot = search_node(root,num); if(realRoot->left == NULL && realRoot->right == NULL) { numOfChild = 0; } else if((realRoot->left != NULL && realRoot->right == NULL) || (realRoot->left == NULL && realRoot->right != NULL) ) { numOfChild = 1; } else if(realRoot->left != NULL && realRoot->right != NULL) { numOfChild = 2; } if(numOfChild == 0) { bool leadRoot = false; if(realRoot == root) { leadRoot = true; } if(leadRoot) { free(realRoot); size--; BSTree(); rem = true; return rem; } if(realRoot->right == NULL && realRoot->left == NULL) { bool toRight; if(realRoot->element > realRoot->parent->element) { toRight = true; } else { toRight = false; } if(toRight) { realRoot->parent->right = NULL; } else { realRoot->parent->left = NULL; } rem = true; free(realRoot); size--; return rem; } } if(numOfChild == 1) { bool leadRoot = false; if(realRoot == root) { leadRoot = true; } if(realRoot->right != NULL && realRoot->left == NULL) { bool toRight; if(leadRoot) { root = realRoot->right; root->parent = NULL; rem = true; free(realRoot); return rem; } if(realRoot->element > realRoot->parent->element) { toRight = true; } else { toRight = false; } if(toRight) { realRoot->parent->right = realRoot->right; realRoot->right->parent = realRoot->parent; } else { realRoot->parent->left = realRoot->right; realRoot->right->parent = realRoot->parent; } rem = true; free(realRoot); size--; return rem; } if(realRoot->left != NULL && realRoot->right == NULL) { bool toRight; if(leadRoot) { root = realRoot->left; root->parent = NULL; rem = true; free(realRoot); return rem; } if(realRoot->element > realRoot->parent->element) { toRight = true; } else { toRight = false; } if(toRight) { realRoot->parent->right = realRoot->left; realRoot->left->parent = realRoot->parent; } else { realRoot->parent->left = realRoot->left; realRoot->left->parent = realRoot->parent; } rem = true; free(realRoot); size--; return rem; } } if(numOfChild == 2) { node* temp; temp = findNewRoot(realRoot->right); if(realRoot->right->element == temp->element) { bool uniqueRight = false; if(temp->right != NULL) { uniqueRight = true; } if(uniqueRight) { realRoot->right = temp->right; temp->right->parent = temp->parent; } else { realRoot->right = NULL; } rem = true; realRoot->element = temp->element; free(temp); size--; return rem; } bool hasRight = false; if(temp->right != NULL) { hasRight = true; } if(hasRight) { temp->parent->left = temp->right; temp->right->parent = temp->parent; } else { temp->parent->left = NULL; } rem = true; realRoot->element = temp->element; free(temp); size--; } return rem; } return isPresent; } bool isEmpty() { // TODO isEmpty return size == 0; } void print_preorder(node* curr) { // TODO preorder traversal cout << curr->element << " "; if(curr->left != NULL) { print_preorder(curr->left); } if(curr->right != NULL) { print_preorder(curr->right); } } void print_postorder(node* curr) { // TODO postorder traversal if(curr->left != NULL) { print_postorder(curr->left); } if(curr->right != NULL) { print_postorder(curr->right); } cout << curr->element << " "; }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
C++ PROGRAMMING
Binary Search Trees
SEE ATTACHED PHOTO FOR THE PROBLEM INSTRUCTIONS
It doesn't have to be long, as long as you explain what the important parts of the code do. (The code is already implemented and correct, only the explanation needed)
#include "node.h"
#include <iostream>
using namespace std;
class BSTree {
node* root;
int size;
node* create_node(int num, node* parent) {
node* n = (node*) malloc( sizeof(node) );
n->element = num;
n->parent = parent;
n->right = NULL;
n->left = NULL;
return n;
}
bool search(node* curr, int num) {
if (curr == NULL) {
return false;
}
if (num == curr->element) {
return true;
}
if (num < curr->element) {
return search(curr->left, num);
}
return search(curr->right, num);
}
node* search_node(node* curr, int num) {
if (num == curr->element) {
return curr;
}
if (num < curr->element) {
if (curr->left != NULL) {
return search_node(curr->left, num);
}
return curr;
}
if (curr->right != NULL) {
return search_node(curr->right, num);
}
return curr;
}
node* findNewRoot(node* curr) {
if(curr->left == NULL)
{
return curr;
}
return findNewRoot(curr->left);
}
public:
BSTree() {
root = NULL;
size = 0;
}
bool isPresent = search(num);
if(isPresent){
bool rem = false;
int numOfChild;
node* realRoot = search_node(root,num);
if(realRoot->left == NULL && realRoot->right == NULL)
{
numOfChild = 0;
}
else if((realRoot->left != NULL && realRoot->right == NULL) || (realRoot->left == NULL && realRoot->right != NULL) )
{
numOfChild = 1;
}
else if(realRoot->left != NULL && realRoot->right != NULL)
{
numOfChild = 2;
}
if(numOfChild == 0)
{
bool leadRoot = false;
if(realRoot == root)
{
leadRoot = true;
}
if(leadRoot)
{
free(realRoot);
size--;
BSTree();
rem = true;
return rem;
}
if(realRoot->right == NULL && realRoot->left == NULL) {
bool toRight;
if(realRoot->element > realRoot->parent->element)
{
toRight = true;
}
else
{
toRight = false;
}
if(toRight)
{
realRoot->parent->right = NULL;
}
else
{
realRoot->parent->left = NULL;
}
rem = true;
free(realRoot);
size--;
return rem;
}
}
if(numOfChild == 1) {
bool leadRoot = false;
if(realRoot == root)
{
leadRoot = true;
}
if(realRoot->right != NULL && realRoot->left == NULL) {
bool toRight;
if(leadRoot)
{
root = realRoot->right;
root->parent = NULL;
rem = true;
free(realRoot);
return rem;
}
if(realRoot->element > realRoot->parent->element)
{
toRight = true;
}
else
{
toRight = false;
}
if(toRight)
{
realRoot->parent->right = realRoot->right;
realRoot->right->parent = realRoot->parent;
}
else
{
realRoot->parent->left = realRoot->right;
realRoot->right->parent = realRoot->parent;
}
rem = true;
free(realRoot);
size--;
return rem;
}
if(realRoot->left != NULL && realRoot->right == NULL) {
bool toRight;
if(leadRoot)
{
root = realRoot->left;
root->parent = NULL;
rem = true;
free(realRoot);
return rem;
}
if(realRoot->element > realRoot->parent->element)
{
toRight = true;
}
else
{
toRight = false;
}
if(toRight)
{
realRoot->parent->right = realRoot->left;
realRoot->left->parent = realRoot->parent;
}
else
{
realRoot->parent->left = realRoot->left;
realRoot->left->parent = realRoot->parent;
}
rem = true;
free(realRoot);
size--;
return rem;
}
}
if(numOfChild == 2) {
node* temp;
temp = findNewRoot(realRoot->right);
if(realRoot->right->element == temp->element)
{
bool uniqueRight = false;
if(temp->right != NULL)
{
uniqueRight = true;
}
if(uniqueRight)
{
realRoot->right = temp->right;
temp->right->parent = temp->parent;
}
else
{
realRoot->right = NULL;
}
rem = true;
realRoot->element = temp->element;
free(temp);
size--;
return rem;
}
bool hasRight = false;
if(temp->right != NULL)
{
hasRight = true;
}
if(hasRight)
{
temp->parent->left = temp->right;
temp->right->parent = temp->parent;
}
else
{
temp->parent->left = NULL;
}
rem = true;
realRoot->element = temp->element;
free(temp);
size--;
}
return rem;
}
return isPresent;
}
bool isEmpty() {
// TODO isEmpty
return size == 0;
}
void print_preorder(node* curr) {
// TODO preorder traversal
cout << curr->element << " ";
if(curr->left != NULL)
{
print_preorder(curr->left);
}
if(curr->right != NULL)
{
print_preorder(curr->right);
}
}
void print_postorder(node* curr) {
// TODO postorder traversal
if(curr->left != NULL)
{
print_postorder(curr->left);
}
if(curr->right != NULL)
{
print_postorder(curr->right);
}
cout << curr->element << " ";
}
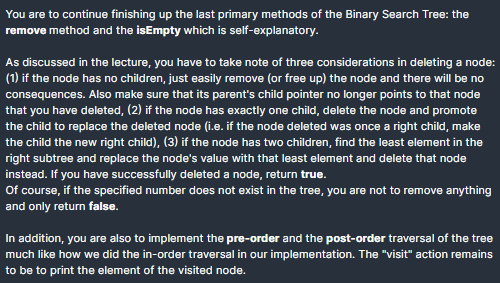
Transcribed Image Text:You are to continue finishing up the last primary methods of the Binary Search Tree: the
remove method and the isEmpty which is self-explanatory.
As discussed in the lecture, you have to take note of three considerations in deleting a node:
(1) if the node has no children, just easily remove (or free up) the node and there will be no
consequences. Also make sure that its parent's child pointer no longer points to that node
that you have deleted, (2) if the node has exactly one child, delete the node and promote
the child to replace the deleted node (i.e. if the node deleted was once a right child, make
the child the new right child), (3) if the node has two children, find the least element in the
right subtree and replace the node's value with that least element and delete that node
instead. If you have successfully deleted a node, return true.
Of course, if the specified number does not exist in the tree, you are not to remove anything
and only return false.
In addition, you are also to implement the pre-order and the post-order traversal of the tree
much like how we did the in-order traversal in our implementation. The "visit" action remains
to be to print the element of the visited node.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Recommended textbooks for you
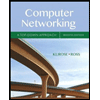
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
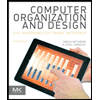
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
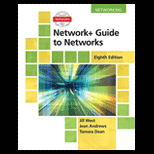
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
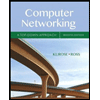
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
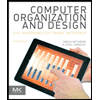
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
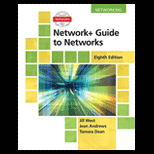
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
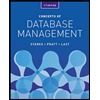
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
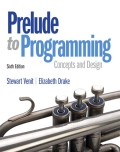
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
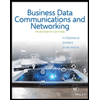
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY