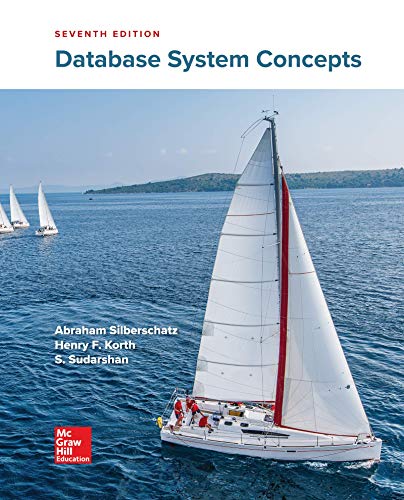
This is the code so far
#include <iostream> // for cin and cout
#include <iomanip> // for setw() and setfill()
using namespace std; // so that we don't need to preface every cin and cout with std::
void printSpaces(int n, int end)
{
for (int j = n; j > end; j--)
{
cout << " ";
}
}
void printFirstTwoBuildingSection(int n, int startSpacing)
{
int start = 2 * ((n / 2) - 1), end = 0;
for (int i = 0; i < n; i++)
{
printSpaces(startSpacing, 0);
cout << "|";
if (i < (n / 2))
{
printSpaces(i, 0);
cout << "\\";
printSpaces(start, 0);
cout << "/";
start -= (2);
printSpaces(i, 0);
cout << "|" << endl;
}
else
{
printSpaces(n - i - 1, 0);
cout << "/";
printSpaces(end, 0);
cout << "\\";
end += (2);
printSpaces(n - i - 1, 0);
cout << "|" << endl;
}
}
printSpaces(startSpacing, 0);
cout << "/";
for (int i = 0; i < n; i++)
{
cout << "-";
}
cout << "\\" << endl;
}
int main()
{
int menuOption = 0;
cout << "Choose from among the following options:\n"
<< "1. Exit the program\n"
<< "2. Display building\n"
<< "Your choice -> ";
cin >> menuOption;
cout << endl; // Leave a blank line after getting the user input for the menu option.
// See if exit was chosen
if (menuOption == 1)
{
exit(0);
}
// Menu 2
if (menuOption == 2)
{
int sections;
cout << "Number of building sections -> ";
cin >> sections;
int n = 1 - (sections - 2) + sections * 2;
int sizeOfBuild = 2;
cout << setw(n) << " /\\ " << endl;
cout << setw(n) << " || " << endl;
cout << setw(n) << " || " << endl;
cout << setw(n) << " -- " << endl;
cout << setw(n) << "|++|" << endl;
cout << setw(n) << "====" << endl;
for (int i = 1; i <= sections; i++, sizeOfBuild += 2)
{
printFirstTwoBuildingSection(sizeOfBuild, sections - i);
}
}
cout << endl;
return 0;
}
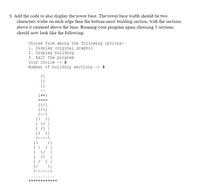

Step by stepSolved in 2 steps with 1 images

- #include <iostream>using namespace std;class st{private:int arr[100];int top;public:st(){top=-1;}void push(int ItEM) {top++;arr[top]=ItEM; }bool ise() {return top<0;} int pop(){int Pop;if (ise()) cout<<"Stack is emptye ";else{Pop=arr[top];top--;return Pop;}}int Top(){int TOP;if (ise()) cout<<" empty ";else {TOP=arr[top];return TOP;}}void screen(){ for (int i = 0; i <top+1 ; ++i) {cout<<arr[i];} }};int main() {st c;c.push(1);c.push(2);c.push(3);c.push(4);c.pop();c.push(5);c.screen();return 0;} alternative to this code??arrow_forwardPls help ASAP. Language is C#arrow_forwardIn c++ Please show me the Test output *You may not use any STL containers in your implementations Need help with the following functions: -LinkedList(const LinkedList<T>& other_list) Constructs a container with a copy of each of the elements in another, in the same order. -LinkedList& operator=(const LinkedList& other_list) Replaces the contents of this list with a copy of each element in another, in the same order. -void resize(std::size_t n) resizes the list so that it contains n elements. -void resize(std::size_t n, const T &fill_values) resizes the list so that it contains n elements -void remove(const T &val) Removes from the container all the elements that compare equal to val - bool operator == (const LinkedList &another) Compares this list with another for equality - bool operator != (const LinkedList &another) Compares this list with another for equality CODE #include <iostream> template <typename T> class LinkedList { struct…arrow_forward
- In c++ Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, th backwards End each loop with a newline. Ex If courseGrades (7, 9, 11, 10), print: 7 9 11 10 10 11 9 7 Hint Use two for loops. Second loop starts with i=NUM VALS-1. (Notes) Note: These activities may test code with different test values. This activity will perform two tests, both with a 4-element array (int courseGrades(4) See 'How to Use zyBooks Also note: If the submitted code tries to access an invalid array element, such as courseGrades[9] for a 4-element array, the test may generate strange results. Or the test may crash and report "Program end never reached", in which case the system doesn't print the test case that caused the reported message 32344345730rity? 2 using namespace std; 3 4 int main() { 5 6 7 8 9 10 11 12 13 14 const int NUM VALS-4; int courseGrades [NUM VALS]; int i; for (i = 0; i > courseGrades[1]; } y Your solution goes here Yarrow_forwardFind error #include<bits/stdc++.h> using namespace std; class Solution{ public: bool isPossible(vector<int>ank,int n,int mid) { int count=0; for(int i=0;i<rank.size()) { int val= (-1 + sqrt(1+(8*mid)/rank[i]))/2; count+=val; } return count>=n; } int findMinTime(int N, vector<int>&A, int L){ int low=*min_element(A.end()),high=1000000; int ans=high; while(low<=high) { int mid=low+(high)/2; if(isPossible(A,mid)) { ans=mid; high=mid; } else low=mid; } return ans; } }; int main() { int t; cin>>t; while(t--) { int l; cin >> l; vector<int>arr(l); for(int i = 0; i < l; i++){ cin >> arr[i]; } Solution ob; int ans = ob.findMin(n, *arr, l); cout…arrow_forwardThis is What i have so far. #include <iostream> // for cin and cout#include <iomanip> // for setw() and setfill()using namespace std; // so that we don't need to preface every cin and cout with std:: void printFirstTwoBuildingSection(int n, int startSpacing){int start = n / 2, end = 0;if (n <= 2){start = 0;}for (int i = 0; i < n; i++){for (int j = 0; j < startSpacing; j++){cout << " ";}cout << "|";if (i < (n / 2)){for (int k = 0; k < i; k++){cout << " ";}cout << "\\";for (int k = 0; k < start; k++){cout << " ";}cout << "/";start -= (n / 2);for (int k = 0; k < i; k++){cout << " ";}cout << "|" << endl;}else{for (int k = n - i - 1; k > 0; k--){cout << " ";}cout << "/";for (int k = 0; k < end; k++){cout << " ";}cout << "\\";end += (n / 2);for (int k = n - i - 1; k > 0; k--){cout << " ";}cout << "|" << endl;}}for (int j = 0; j < startSpacing;…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
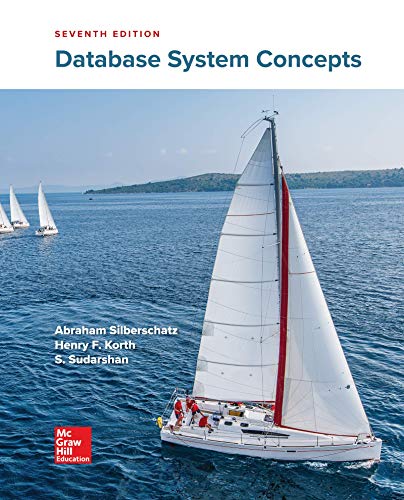
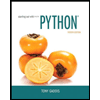
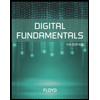
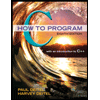
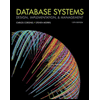
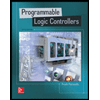