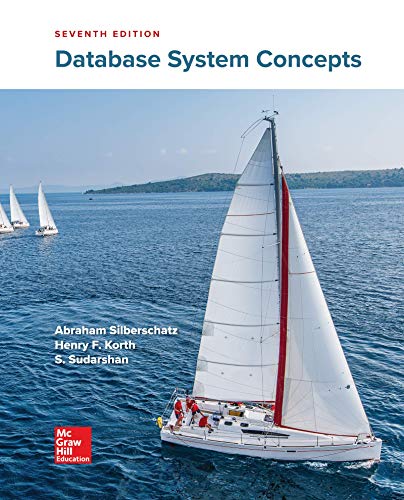
JAVA CODING QUESTION. Object-oriented and constructor chaining!!!
Build a "country" class. A country object will have 2 attributes its name and an array of the names of football stadiums in the country
You have to assume there is a football stadium class that consists of just one constructor that has only one input parameter, which is its name.
Add the following constructors to the country class:
1) Country(String country_name) //country has no football stadium
2)Country(String country_name, String football_stadium_name) //country has exactly 1 football stadium
3)Country(String country_name, football_stadium[] football_stadiums) //country has multiple football stadiums
YOU HAVE TO USE CONSTRUCTOR CHAINING!!
After coding answer the following question in words:
When making a country object what is the maximum amount of constructor methods that are called and what is the minimum amount that will be called. Write an example for both situations

Step by stepSolved in 2 steps

- Lab 2 – Designing a class This lab requires you to think about the steps that take place in a program by writing pseudocode. Read the following program prior to completing the lab. Design a class named Computer that holds the make, model, and amount of memory of a computer. Include methods to set the values for each data field, and include a method that displays all the values for each field. For the programming problem, create the pseudocode that defines the class and enter it below. Enter pseudocode herearrow_forwardAssignment 2 A Student class has the following attributes: String name; String streetAddress; int age; String phonNumber; String mobileNumber; Requirements: . Create the setters and getters . Create one default constructor • create one paramertized constructor • Create an object of the class using the default constructor . ● . Create another object of the class using the paramertized constructor Make sure to suply the values from the keyboard Print the information about each employee . Make sure to add comments to you code and that your code is indented corectly . Use the camelCase naming convension. . Use try and catch block. After you are doneo a samle run and tak a screenshot of it. zip the screenshot and the sourc code file and upload the zipped file to the Blackboard.arrow_forwardMake C# (Sharp): console project named MyPlayList. Create an Album class with 4 fields to keep track of title, artist, genre, and copies sold. Each field should have a get/set property. Your Album class should have a constructor to initialize the title (other 3 variables should default to null or 0). In addition, create a public method in the Album class to calculate amount sold for the album (copies sold * cost_of_album). You determine the cost of each album. Also create a method in the Album class to display the information. Print out of information should look something like this: The album Tattoo You by the Rolling Stones, a Rock group, made $25,000,000. In the main method, create 3 instances of the Album class with different albums, use setters to set values of the artist, genre, and copies sold. And then use getters to display information about each on the console.arrow_forward
- A field has access that is somewhere between public and private. static final Opackage Oprotectedarrow_forwardProblem Description and Given Info For this assignment you are given the following Java source code files: IStack.java (This file is complete – make no changes to this file) MyStack.java (You must complete this file) Main.java (You may use this file to write code to test your MyStack) You must complete the public class named MyStack.java with fields and methods as defined below. Your MyStack.java will implement the IStack interface that is provided in the IStack.java file. You must implement your MyStack class as either a linked list or an array list (refer to your MyArrayList and MyLinkedList work). Your MyStack must not be arbitrarily limited to any fixed size at run-time. UML UML CLass Diagram: MyStack Structure of the Fields While there are no required fields for your MyStack class, you will need to decide what fields to implement. This decision will be largely based on your choice to implement this MyStack as either an array list or a linked list. Structure of the Methods As…arrow_forwardclass Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name "Rubik's Cube" >>> your_widget.cost 6 >>> your_widget.is_cheap() True """ # Add your methods here if __name__ == '__main__': import doctest # Uncomment the line below if you prefer to test your examples with doctest # doctest.testmod()arrow_forward
- Portfolio Instructions: You are working for a financial advisor who creates portfolios of financial securities for his clients. A portfolio is a conglomeration of various financial assets, such as stocks and bonds, that together create a balanced collection of investments. When the financial advisor makes a purchase of securities on behalf of a client, a single transaction can include multiple shares of stock or multiple bonds. It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date. The characteristics of stocks and bonds in a portfolio are shown below: Stocks: Bonds: Purchase date (Date) Purchase date (Date) Purchase price (double)…arrow_forwardCar Class Project The car classwill havethe following attributes: •year: an integer that holds the car's model year •model: a string that holds the make of the car •make: a string that holds the model of the car •speed: an integer that holds the car's current speed Your class should contain thefollowing: A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class. •A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string. •To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly. •An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed(). •A modifier method called accelerate() which adds 5 to the speed variable…arrow_forwardTrue or False ___(1) An abstract class can have fields. ___(2) You can create an object from an abstract class _ _(3) An abstract class can have both abstract methods and methods that have method bodyarrow_forward
- Describe the class's private and public components.arrow_forwardJAVA Programming Problem 3 - Book A Book has such properties as title, author, and numberOfPages. A Volume will have properties such as volumeName, numberOfBooks, and an array of book objects (Book [ ]). You are required to develop the Book and Volume classes, then write an application (DemoVolume) to test your classes. The directions below will give assistance. Create a class called Book with the following properties using appropriate data types: Title, Author, numberOfPages, Create a second class called Volume with the following properties using appropriate data types: volumeName, numberOfBooks and Book [ ]. The Book [ ] contains an array of book objects. Directions Create a class called Book with the following properties using appropriate data types: Title, Author, numberOfPages, The only methods necessary in the Book class, for this exercise, are the constructor and a toString(). Create a second class called Volume with the following properties using appropriate data types:…arrow_forwardRemaining Time: 35 minutes, 18 seconds, ¥ Question Completion Status: A class named Account is defined as the following. class Account } private: int id; double balance; public: //A constructor without parameter that creates a default account with id 0, balance 0 Account): /A constructor with the parameter that setting the id and balance Account(int, double), int getId); / return the ID double getBalance() //return the balance void withdraw(double amount) // the amount will be withdrawn void setlID(int), //set the ID void setBalance (double) //set the balance }: Assume that we will create a separate file of implementation of the function definition for the questions a) -b) a) Write a construction function definition with two parameters (ID and balance). b) Write a function definition of the function declaration (void withdraw(double amount):). c) Creates an Account object with an ID (1122) and balance ($20000). Then, write source codes to withdraw $2 MacBooarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
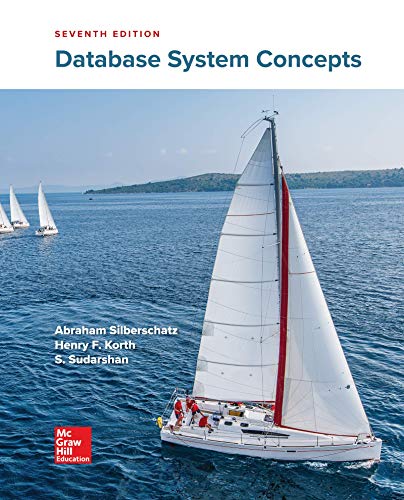
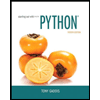
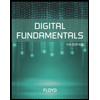
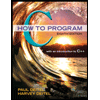
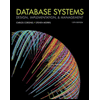
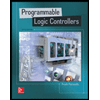