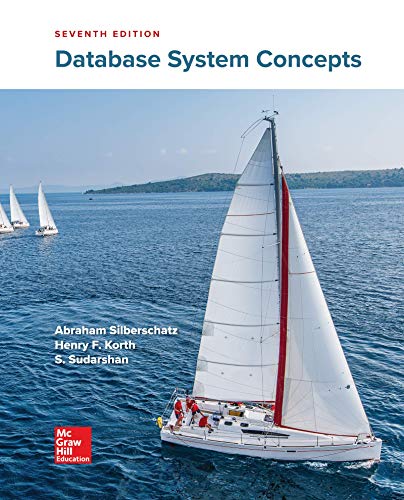
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
(Java)
DO PART 2! PART 2 IS BASED OFF PART 1!!!
PART 1:
- Open up a new Java project called Activity6 and create a class called Person
- Inside of the class, define three attributes that a person has as variables of the class
- Name
- Age
- Gender
- Next, define two actions that can be performed as methods of the class:
- The method is named greeting
- It takes no parameters
- It prints out the message "Hi, my name is " + name + "!"
- It returns nothing
- The method is named addYear
- It takes in no parameters
- It adds one year to the person's age
- It returns nothing
- Then, create a new Java file called PersonTest.java.
- Copy and paste the below test code into PersonTest.java:
/**
* @author
* CIS 36B, Activity 6.1
*/
public class PersonTest {
public static void main(String[] args) {
Person wen = new Person();
wen.name = "Wen";
wen.age = 19;
wen.gender = "male";
wen.greeting();
wen.addYear();
System.out.println("I just turned " + wen.age + " years old.");
}
}
- Now, run the program and verify that you get the following output:
Hi, my name is Wen!
I just turned 20 years old.
Part 2:
- Open up your Person class from the last activity.
- Add a second method to this class. Here are the specifications for this method:
- The method is named greeting
- It takes in one String parameter for another person's name
- It prints out the message "Hi, " + otherName + "!"
- It returns nothing.
- Add a final method to this class.
- The method is named printPerson
- It prints a Person's name, age and gender to the console, in the format
Name: <name>
Age: <age>
Gender: <gender>
- It returns nothing
- Copy and paste the new test file below into PersonTest.java (you can erase the old contents of the file)
- Add the missing 4 lines of code.
- Then run your code to verify you have the correct output (shown below):
* @author
* CIS 36B, Activity 6.2
*/
import java.util.Scanner;
import java.util.ArrayList;
public class PersonTest {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
Person wen = new Person();
wen.name = "Wen";
wen.age = 19;
wen.gender = "male";
wen.greeting();
System.out.print("\nWhat's your name: ");
String name = input.nextLine();
//call greeting method passing in name parameter
System.out.print("\nHow old are you: ");
int age = input.nextInt();
System.out.print("What is your gender: ");
String gender = input.next();
//make a new Person here and assign that Person the name, age and gender read from console
ArrayList<Person> pair = new ArrayList<Person>(2);
pair.add(wen);
//add second person to ArrayList here
System.out.println("\nPair
for (int i = 0; i < pair.size(); i++) {
//add a line of code to call printPerson on each person in ArrayList
}
}
}
- Now, run the program and verify that you get the new output (given the below input):
What's your name: Maria
Hi, Maria!
How old are you: 20
What is your gender: female
Pair Programming Partners:
Name: Wen
Age: 19
Gender: male
Name: Maria
Age: 20
Gender: female
- When you are getting the correct output, submit your Person.java *and* PersonTest.java
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- create different java methods in making the code and have at least 2 or more classesarrow_forward1) create a class Cat... Create class Cat Add properties What characteristics do they have?Name, age, color, type (domestic / feral), etc. What color(s) might they have? White, cream, fawn, Cinnamon, Chocolate, Red, Lilac, Blue, Black,Lavender, etc. Add methods eat(), play(), etc. Constructors? A class provides a special type of methods, known as constructors, which areinvoked to construct objects from the class Create 2 different constructor with different initialization parameters How to get the number of cats? Add a static variable and a static method Test Cat class by creating 2 Cat instances 2) create an Abstract class AbstractCat with the sameproperties and methods as Cat... Create class AbstractCat. How to secure data? To protect it from direct access and manipulation from outside of the classwe need to make our instance data fields private. To let clients still manipulate data under our control we can provide thempublic getters and setters. create getters, setters,…arrow_forwardNeed it in the java language, doesn't have to be perfect. If you can do the whole program that would be best but if not an outline would suffice so I can get a good idea of how to finish the program. Thanks!arrow_forward
- 4. Using arrays in Java statements (comparison, equations, instantiating/creating...) 5. Declaring variables and constants 6. Method Headers 7. Parameter lists 8. Classes and Objects 9. Constructors 10. Evaluation Math Expressions 11. Java Standard Class Library (packages) 12. Using Classes - Random, DecimalFormat, Math, Keyboard, NumberFormat, String 13. Writing Math equations 14. The return statement and return types for methods 15. Converting binary numbers 16. Data types and literal valuesarrow_forwardWrite a java code for the following. Create a class called student Define a constructor to initialize student details (name, branch, rollnumber) Define a method NumberOfStudents method to count number of students registered Create a class called Main Define main method Create four student objects with respect to constructor call NumberOfStudents method for each created object print the number of students registeredarrow_forwardwrite a class book, which has 3 attributes: title, price, quantity. Write the following methods, (8 points) a. setter/getter methods for each of the 3 attributes. b. __str__ method to display the complete information for the book, including title, price and quantity c. Method sell_copies(), passing the number of copies sold, which will reducethe value of quantity of the book. write the code in python please and thank youarrow_forward
- Use Python for this question: Develop a class Volume that stores the volume for a stereo that has a value between 0 and 11. Usage of the class is listed below the problem descriptions. Throughout the class you must guarantee that: The numeric value of the Volume is set to a number between 0 and 11. Any attempt to set the value to greater than 11 will set it to 11 instead, any attempt to set a negative value will instead set it to 0. This applies to the following methods below: __init__, set, up, down You must write the following methods: __init__ - constructor. Construct a Volume that is set to a given numeric value, or, if no number given, defaults the value to 0. (Subject to 0 <= vol <=11 constraint above.) __repr__ - converts Volume to a str for display, see runs below. set – sets the volume to the specified argument (Subject to 0 <= vol <=11 constraint above.) get – returns the numeric value of the Volume up – given a numeric amount, increases the Volume by…arrow_forwardAnswer with True or False for the following. Explain your answers.-It is legal to write a method in a class which overloads another method declared in the same.-It is legal to write a method in a superclass which overrides a method declared in a sub-class.arrow_forwardJAVAarrow_forward
- Write a simple cash register class. (Use Java) Each cash register should keep track of the number of items checked out and the total cash in the register. We are only concerned with the number of items to check out and their prices. No need to handle item names, SKUs, taxes, etc... It should have the following methods (use static when appropriate): checkout( ) Takes two forms. If there is only one parameter that is the cash received and there is only one item checked out. If it has two parameters, the first parameter should have the number of items checked out and the second parameter is the price per item. cashout( ) Reset the number of items and total cash to zero for a cash register getItems( ) Returns the number of items in the cash register getTotalCash( ) Returns the total cash in the cash register getAveragePricePerItem( ) Returns the average price per item checked out by the cash register getRegisterCount( ) Returns the number of cash registers created getAllItems(…arrow_forwardJAVA. THIS IS A PROGRAMMING ASSIGNMENT NOT A WRITING ASSIGNMENT IF YOU ANSWERED BEFORE DO NOT ANSWER AGAIN PLEASE DO NOT PASTE A PAPER Design a class to store the following information about computer scientists⦁ Name⦁ Research area(s)⦁ Major contribution A scientist may have more than one area of research.⦁ Implement the usual setters and getters methods ⦁ Implement a method that takes a research area as an input and return thenames of scientists associated with that area ⦁ Implement a method that takes a word as an input and return the names ofscientists whose contribution mention that word Next, perform desktop research to find at least five prominent black computerscientists, with their research area(s) and major contribution, and store this data intoobjects of the class you designed. You can just hardcode this data. No user input needed.Define JUnit tests to check your research area and contribution search methods.arrow_forwardcan someone help me? The questions are: 1. Add an addExerciseAdd method that adds an exercise to a private variable exercises in Exercise You don't need to create a getter or setter for this private variable. 2. Modify the constructors in Exercise so that you can create two types of workouts (let one constructor use the other; each variable can only have a value in one constructor): a training schedule with exercises, but without a trainer. a training schedule with exercises and with a trainer. import java.util.ArrayList;class Exerciseplan {private String customer;private String trainer;Exerciseplan(String customer) {this.customer = customer;}Exerciseplan(String customer, String trainer) {this.customer = customer;this.trainer = trainer;}private void printExercise(String name, String muscleGroup, Integer numberOfSets, Integer repetition, Integer restTime) {System.out.println("Oefening voor " + muscleGroup + ":" +" herhaal " + numberOfSets + " keer " +"(rust tussendoor " + restTime +…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
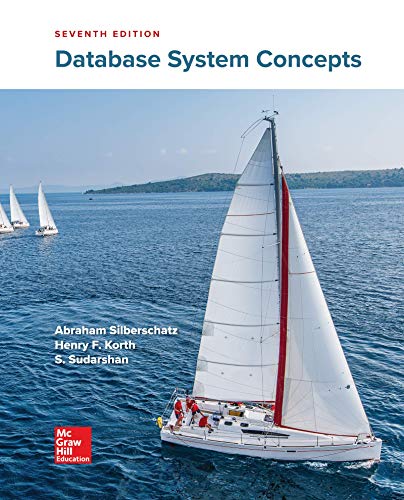
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
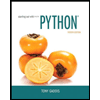
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
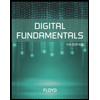
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
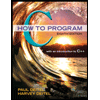
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
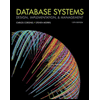
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
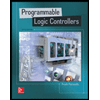
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education