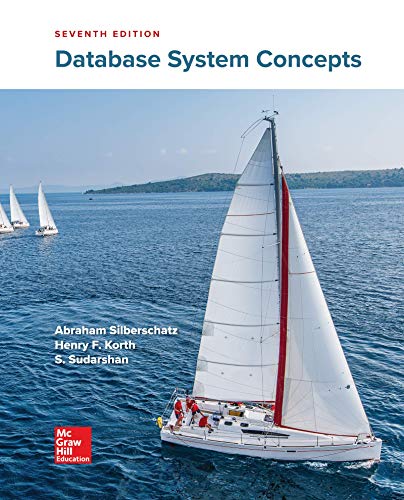
Concept explainers
Java
Given a 2 dimensional array, write a method to return the average of each row.
The result must be returned as a one dimensional array. The array may be ragged.
For example:
int[][] my2DArray = {
{2,5,5},
{2,6,8,4},
{6,5,2,3}
}
The result should be:
int[] myArrayAverage = {
4, //Average of {2,5,5}
5, //Average of {2,6,8,4}
4 //Average of {6,5,2,3}
}
Note: You only need to write down the code inside the myMethod() code block
public class CST1201Test3
{
public static void main(String[] args)
{
int[][] my2DArray = {
{2,5,5},
{2,6,8,4},
{6,5,2,3}
};
int[] myArrayAverage = myMethod(my2DArray));
}
static int[] myMethod(int[][] numbers)
{
// your answer
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- JAVA complete a method that swaps the first and second half of an array of integers. For example, if the array contains the values 1 4 9 16 25 36then after calling the method, it should contain the values16 25 36 1 4 9If the array contains an odd number of elements, leave the middle element in place. For example, 1 4 9 16 25 36 45becomes25 36 45 16 1 4 9arrow_forwardJAVA programming language i need to create an "addFront" method in the bottom of the code that adds an element at the front. [-1, -3, -5] A.add(-9) ===> [-9, -1, -3, -5]. Its all the way in the bottom, please help me. public class ourArray { //define an array of integers private int[] Ar; //Ar is a reference //declare private int capacity = 100; private int size = 0; private int increment = 10; //default constructor public ourArray() { Ar = new int[100]; capacity = 100; size = 0; increment = 10; } //Constructor that accepts the capacity (C). It creates an array of C integersm, sets capacity to C // Increment to 10 public ourArray(int C) { Ar = new int [C]; size = 0; capacity = C; increment = 10; } //Constructor that accepts two integers (c, i) c for capacity and i for increment public ourArray (int C, int I) { Ar = new int[C]; size = 0; capacity = C; increment = I; } //setter for Increment public void setincrement(int I) { increment = I; } //getter for size, capacity…arrow_forwardin java programming answer the following: Given an array called myArr (created as ArrayList<T>) and contains the {1,2,3,4,5,6,7}. (a) what would be the contents of this array after myArr.add(10). b) write the content of myArr after myArr.add(4, 11);arrow_forward
- In Java: Write a method that multiplies all elements in the array by 3 and returns it to the main method.arrow_forwardMethod Details: public static java.lang.String getArrayString(int[] array, char separator) Return a string where each array entry (except the last one) is followed by the specified separator. An empty string will be return if the array has no elements. Parameters: array - separator - Returns: stringThrows:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forwardjava Create a static method that: is called appendPosSum returns an ArrayList of Integers takes one parameter: an ArrayList of Integers This method should: Create a new ArrayList of Integers Add only the positive Integers to the new ArrayList Sum the positive Integers in the new ArrayList and add the Sum as the last element For example, if the incoming ArrayList contains the Integers (4,-6,3,-8,0,4,3), the ArrayList that gets returned should be (4,3,4,3,14), with 14 being the sum of (4,3,4,3). The original ArrayList should remain unchanged. public static void main(String[] args) { Scanner in = new Scanner(System.in); int size = in.nextInt(); ArrayList<Integer> list = new ArrayList<>(); for(int i=0; i < size; i++) { list.add(in.nextInt()); } System.out.println(appendPosSum(list));arrow_forward
- Java - Sort an Arrayarrow_forwardA method named getUserStringsAndPopulate Array(). This method should keep prompting the user to enter as many strings as the user wants. The user should be able to stop entering strings by entering EXIT. This method should take the strings entered by the user and populate a one-dimensional array of strings with the user input. The string EXIT should NOT be added to the array because it is a special flag that will tell the method to stop prompting the user to enter more strings. This method should return the one-dimensional array of strings populated with user input and should not accept any arguments. 2.b) A method named searchArray() that would accept a one-dimensional array of strings and search the array for the string Winter. The search logic should NOT be case sensitive. If a match is found, this method should print the string in the array followed by a message like " --- Found a match!". Otherwise, the method should not print anything. This method should NOT return anything.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
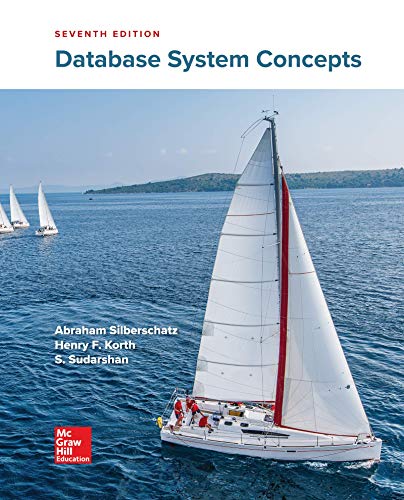
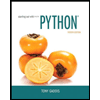
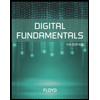
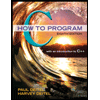
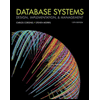
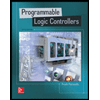