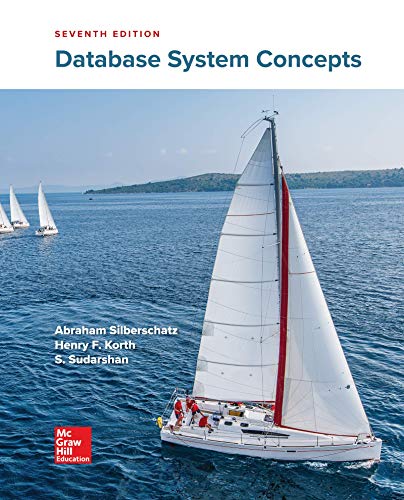
Concept explainers
JAVA Only
Design, implement and test a Java class that processes a series of triangles. The triangle specification will be identical to that in
The data for each triangle will be on a separate line of the input file, with the input data echoed and the results referred to by the line number (see example).
Some things to note:
- All input data lines must be processed.
- A new line with triangle data always starts with the ‘#’ marker.
- If an error is encountered, it must be handled and the program will continue.
- Extraneous data must be discarded. This is data that appears after the first three triangle sides are found, but before a new triangle marker is found.
- If the end of file (EOF) is found, a message should be printed out
- There shall be no unhandled file related exceptions and an end-of-file exception must be avoided.
On initialization, the program will prompt the user for both an input and an output file. If a non-existent input file is specified, the appropriate exception must be handled, resulting in an error message. For the exception case, re-prompt the user for the correct input file [Extra credit if you implement a JFileChooser type dialog box]. Once the I/O file is specified, the program will read in and process all the entries in the file. The output of the program will be written to the specified output file and echoed to the console.
The program will evaluate each line and determine the validity of the triangle. If valid, the program will output summary line for the triangle calculation (see Programming Assignment #2A). If invalid, the program will output a description of the error found in the entry. Your program must be robust and handle situations where all the triangle inputs are not proper and/or present.
Once all the entries are processed, a summary will be generated providing the following information:
- Pathname of the input file
- Number of lines processed
- Number of valid triangles
- Number of invalid triangles
You should be able to reuse much of your Programming Assignment #2A code for this project. However, strongly recommend that you rewrite your code and put this functionality in separate method(s). Likewise, any residual problems from #2A should be fixed in this version. The main features you will be adding are:
- Multiple methods
- Exception handing
- File I/O
- Dialog box
Sample file input:
# 3 4 5
# 5 5 5
# 5 5 7 XXX
# -3 10 10 15
# 0 0 0
# 3 4.1 5
# 3 x 4
# 5 4 3 6 YYY ZZZ
# 1 1 10
# 15 16 22
# 3 4
Corresponding file output:
1: 3, 4, 5: The triangle is a right triangle,
The area of this triangle is 6.00
2: 5, 5, 5: The triangle is equilateral,
The area of this triangle is 10.83
3: 5, 5, 7: The triangle is isosceles,
The area of this triangle is 12.50
Item discarded: XXX
Error - At least one of your sides is an invalid integer value
Item discarded: 15
Error - At least one of your sides is an invalid integer value
Error - At least one of your sides is not an integer
Error - At least one of your sides is not an integer
Error - Sides not in ascending order
Item discarded: 6
Item discarded: YYY
Item discarded: ZZZ
Error - Sides do not form a valid triangle
4: 15, 16, 22: This triangle is not distinctive,
The area of this triangle is 120.00
EOF found
File Path: E:\Java\Assign-05\pa5-test-01.txt
--------------- Summary Report ---------------
Total entries processed = 10
Number of valid entries = 4
Number of invalid entries = 6
Percentage of valid entries = 40.00%

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 8 images

- Write a C#program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string) ClassRegistration -…arrow_forwardYou have been hired at an open-air mine. You are to write a program to control a digger. For your task, you have been given a `map' of all the underground resources in the mine. This map comes as a file. The file has n rows. Each row has n space-separated integers. Each integer is between zero and one hundred (inclusive). The file should be understood to represent a grid, n wide by n deep. Each square in the grid can contain valuable resources. The value of these resources can go from zero (no resources) to 100 (max resources). The grid maps the resources just below the surface, and down to the lowest diggable depths. The digger starts at the surface (so, just on top of the topmost row in the grid)—at any horizontal position between 1 and n. The digger cannot dig directly downwards, but it can dig diagonally in either direction, left-down, or right-down. In its first time-step, it will dig onto the first row of the grid. In its second time-step, it'll hit the second row, and so on.…arrow_forwardwrite in c++Write a program that would allow the user to interact with a part of the IMDB movie database. Each movie has a unique ID, name, release date, and user rating. You're given a file containing this information (see movies.txt in "Additional files" section). The first 4 rows of this file correspond to the first movie, then after an empty line 4 rows contain information about the second movie and so forth. Format of these fields: ID is an integer Name is a string that can contain spaces Release date is a string in yyyy/mm/dd format Rating is a fractional number The number of movies is not provided and does not need to be computed. But the file can't contain more than 100 movies. Then, it should offer the user a menu with the following options: Display movies sorted by id Display movies sorted by release date, then rating Lookup a release date given a name Lookup a movie by id Quit the Program The program should perform the selected operation and then re-display the menu. For…arrow_forward
- java programming I have a Java program with a restaurant/store menu. Can you please edit my program when displaying the physical receipt? I would like the physical receipt to export as text file, not print the console's order receipt. import java.util.*; public class Restaurant2 { publicstaticvoidmain(String[] args) { // Define menu items and pricesString[] menuItems= {"Apple", "Orange", "Pear", "Banana", "Kiwi", "Strawberry", "Grape", "Watermelon", "Cantaloupe", "Mango"};double[] menuPrices= {1.99, 2.99, 3.99, 4.99, 5.99, 6.99, 7.99, 8.99, 9.99, 10.99};StringusersName; // The user's name, as entered by the user.ArrayList<String> arr = new ArrayList<>(); // Define scanner objectScanner input=new Scanner(System.in); // Welcome messageSystem.out.println("Welcome to AppleStoreRecreation, what is your name:");usersName = input.nextLine(); System.out.println("Hello, "+ usersName +", my name is Patrick nice to meet you!, May I Take Your Order?"); // Display menu items and…arrow_forwardJava programming 1. Write the code to print out an individual customer’s information. Your code must read the customer from a file, populate the object, and display the data from the object. Customer customer = new Customer (); 2. Write the signature line for this method 3, Write the line of code to read a line from the file Assume the object has been populated with the proper values. Write the line of code that will print one of the properties in the customer object. Be sure to include a textual description, e.g. “Customer Name: “arrow_forwardWrite a program in C++ that prints a sorted phone list from a database of names and phone numbers. The data is contained in two files named "phoneNames.txt" and "phoneNums.txt". The files may contain up to 2000 names and phone numbers. The files have one name or one phone number per line. To save paper, only print the first 50 lines of the output. Note: The first phone number in the phone number file corresponds to the first name in the name file. The second phone number in the phone number file corresponds to the second name in the name file. etc. You will find the test files in the Asn Five.ziparrow_forward
- You are going to write a program (In Python) called BankApp to simulate a banking application.The information needed for this project are stored in a text file. Those are:usernames, passwords, and balances.Your program should read username, passwords, and balances for each customer, andstore them into three lists.userName (string), passWord(string), balances(float)The txt file with information is provided as UserInformtion.txtExample: This will demonstrate if file only contains information of 3 customers. Youcould add more users into the file.userName passWord Balance========================Mike sorat1237# 350Jane para432@4 400Steve asora8731% 500 When a user runs your program, it should ask for the username and passwordfirst. Check if the user matches a customer in the bank with the informationprovided. Remember username and password should be case sensitive.After asking for the user name, and password display a menu with the…arrow_forwardChallenge 3: Encryption.java and Decryption.java Write an Encryption class that encrypts the contents of a binary file. Your encryption program should work like a filter, reading the contents of one file, modifying the data into a code, and then writing the coded content out to a second file. The second file will be a version of the first file, but written in a secret code. Use a simple encryption technique, such as reading one character at a time, and add 10 to the character code of each character before it is written to the second file. Come up with your own encryption method. Also write a Decryption class that reads the file produced by the Encryption program. The decryption file will use the method that you used and restore the data to its original state, and write it to a new file.arrow_forwardWrite a program in C++ that reads a list of enrollments from a file and prints a class roster for the teacher. The program would use files, functions, and classes.Here's what the output should look like: $ ./enroll.out Welcome to the Roster Generator Program!!! Please enter the name of your names file: bob.dat I'm sorry, I could not open 'bob.dat'. Please enter another name: csc442 File 'csc442' opened successfully! Name | Major | Phone # | Town ----------------+--------------------+---------------+------------------- Jason James | Computer Science | 555/555-1122 | Westfalia, HI 665 Faruk Ahmed | Medeaval French Po | 332-4546 | Slimsville, PQ 22 Sean Ramirez | Underwater Basket | 1-444/411-441 | Fritter, HO 44331 Happy Gilmore | Yellow Tail Migrat | | Simonton, SZ 1124 Total Enrollment: 4 Thank you for using the RGP!! Endeavor to have a gyroscopic day! $arrow_forward
- in c++ codearrow_forwardArithmetic Exception Arman has a shop where he provides stuff on rent. The whole payment system is computerised. A customer has to enter the item name, item type, cost, and the number of days they have taken the item for rent. The system then tells them the cost per day. But there is a problem with the machine that if the number of days entered is not an integer value then the system would crash. To handle this, Arman decided to upgrade the software and use exception handling in case the customer entered any invalid input it should display " Number of days x is invalid".Write a C++ program to find the item cost per day with the cost for n days given. The arithmetic exception has to be thrown if the n is less than or equal to zero.Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.The class Item has the following private data members.…arrow_forwardHello, I’m a beginner java student!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
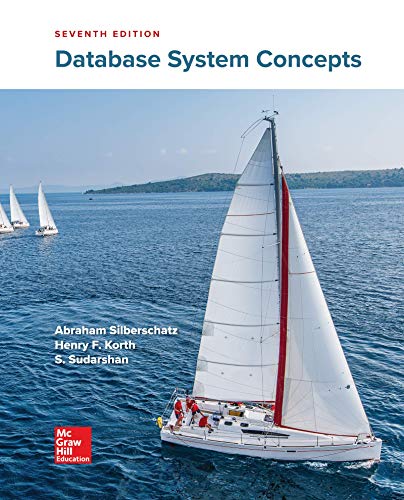
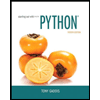
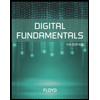
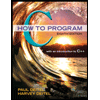
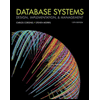
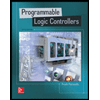