Java Programming: The program will figure out a number chosen by a user. Ask user to think of a number from 1 to 100. The program will make guesses and the user will tell the program to guess higher (h) or lower (l). Sample run of the program might look like below : Guess a number from 1 to 100. (here, the user is thinking of 81) Is it 50? (h/l/c): h Is it 75? (h/l/c): h Is it 88? (h/l/c): l Is it 81? (h/l/c): c Great! Do you want to play again? (y/n): y Guess a number from 1 to 100. (here the user is thinking 37) Is it 50? (h/l/c): l Is it 25? (h/l/c): h Is it 37? (h/l/c): c Great! Do you want to play again? (y/n): n our program should implement the binary search algorithm. Every time the program makes a guess it should guess the midpoint of the remaining possible values. Consider the first example above, in which the user has chosen the number 81: On the first guess, the possible values are 1 to 100. The midpoint is 50. The user responds by saying “higher” On the second guess the possible values are 51 to 100. The midpoint of 75. The user responds by saying “higher” On the third guess the possible values are 76 to 100. The midpoint is 88. The user responds by saying “lower” On the fourth guess the possible values are 76 to 87. The midpoint is 81. The user responds by saying “correct” The main function must look like this, call all functions in main: public static void main(String[] args) { boolean playAgain = true; while (playAgain) { playOneGame(); playAgain = shouldPlayAgain(); } } The playOneGame function should have a return type of void. It should implement a complete guessing game on the range of 1 to 100 inclusive. Both 1 and 100 should be valid guesses for the human user to make. The shouldPlayAgain function should have a boolean return type. It should prompt the user to determine if the user wants to play again, read in a character, then return true if the character is a ‘y’, and otherwise return false. In addition, you should implement the helper functions getUserResponseToGuess, and getMidpoint. They should be invoked inside your playOneGame function. getUserResponseToGuess. This function should prompt the user with the phrase “is it ? (h/l/c): “ with the value replacing the token . It should return a char. The char should be one of three possible values: ‘h’, ‘l’, or ‘c’. It should have the following signature: public static char getUserResponseToGuess(int guess) getMidpoint. This function should accept two integers, and it should return the midpoint of the two integers. If there are two values in the middle of the range then you should consistently choose the smaller of the two. It should have the following signature:
Java Programming:
The program will figure out a number chosen by a user. Ask user to think of a number from 1 to 100. The program will make guesses and the user will tell the program to guess higher (h) or lower (l).
Sample run of the program might look like below :
Guess a number from 1 to 100. (here, the user is thinking of 81)
Is it 50? (h/l/c): h
Is it 75? (h/l/c): h
Is it 88? (h/l/c): l
Is it 81? (h/l/c): c
Great! Do you want to play again? (y/n): y
Guess a number from 1 to 100. (here the user is thinking 37)
Is it 50? (h/l/c): l
Is it 25? (h/l/c): h
Is it 37? (h/l/c): c
Great! Do you want to play again? (y/n): n
our program should implement the binary search
On the first guess, the possible values are 1 to 100. The midpoint is 50.
The user responds by saying “higher”
On the second guess the possible values are 51 to 100. The midpoint of 75.
The user responds by saying “higher”
On the third guess the possible values are 76 to 100. The midpoint is 88.
The user responds by saying “lower”
On the fourth guess the possible values are 76 to 87. The midpoint is 81.
The user responds by saying “correct”
The main function must look like this, call all functions in main:
public static void main(String[] args) {
boolean playAgain = true;
while (playAgain) {
playOneGame();
playAgain = shouldPlayAgain();
}
}
The playOneGame function should have a return type of void. It should implement a complete guessing game on the range of 1 to 100 inclusive. Both 1 and 100 should be valid guesses for the human user to make.
The shouldPlayAgain function should have a boolean return type. It should prompt the user to determine if the user wants to play again, read in a character, then return true if the character is a ‘y’, and otherwise return false.
In addition, you should implement the helper functions getUserResponseToGuess, and getMidpoint. They should be invoked inside your playOneGame function.
getUserResponseToGuess. This function should prompt the user with the phrase “is it <guess>? (h/l/c): “ with the value replacing the token <guess>. It should return a char. The char should be one of three possible values: ‘h’, ‘l’, or ‘c’. It should have the following signature:
public static char getUserResponseToGuess(int guess)
getMidpoint. This function should accept two integers, and it should return the midpoint of the two integers. If there are two values in the middle of the range then you should consistently choose the smaller of the two. It should have the following signature:
public static int getMidpoint(int low, int high)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

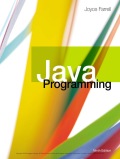
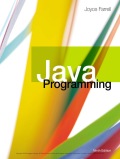