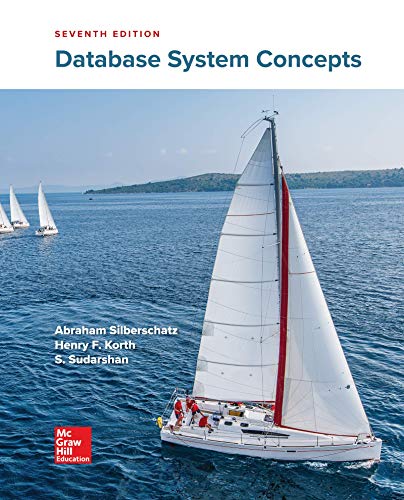
Concept explainers
/ This method takes as parameters a reference to the head of a linked list, a
// position specified by n, and a key. It returns the number of occurrences
// of the key in the linked list beginning at the n-th node. If n = 0, it means
// you should search in the entire linked list. If n = 1, then you should skip
// the first node in the list.
public int numOccurrencesRec(LNode node, int n, int key)
{
// TODO: implement this method
return 0; // replace this statement with your own return
}
}
LNode Below:
public class LNode
{
// instance variables
private int m_info;
private LNode m_link;
// constructor
public LNode(int info)
{
m_info = info;
m_link = null;
}
// member methods
public void setLink(LNode link)
{
m_link = link;
}
public LNode getLink()
{
return m_link;
}
public int getInfo()
{
return m_info;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- DONT use use any of the list methods (append, pop etc.). class mysimplequeue(): def __init__(self, m = 10): self.maxsize = m self.array = [None]*m self.FRONT = self.REAR = -1 def enqueue(self, item): # Inserts item at the rear of a non full queue and returns True. If unable to insert the item returns false ####################################################################### # Remove the pass statement and write your code ####################################################################### pass def dequeue(self): # Removes the item from the front of the queue and returns it. For unsuccessful dequeue return False ####################################################################### # Remove the pass statement and write your code ####################################################################### pass def peek(self): # Returns the…arrow_forwardA consecutive sequence a list of numbers that are organized in increasing order with the next eleme. one bigger than the current. Write a non-recursive method "lengthConsec", which takes an IntNode myList as the parameter and returns the length of the consecutive sequence in myList. To simplify the implementation, you can assume that there is no more than one consecutive sequence in the list. For example, in the following linked list, the consecutive sequence begins at node "5" and ends at node "7", so lengthConsec (myList) should return 3 in this case. myList 8 13 4 public class IntNode { 12 private int m_data; private IntNode m_link; Consecutive sequence 6 7 28arrow_forwardJava Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Second image is ItemNodearrow_forward
- JAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardGiven the interface of the Linked-List struct Node{ int data; Node* next = nullptr; }; class LinkedList{ private: Node* head; Node* tail; public: display_at(int pos) const; ... }; Write a definition for a method display_at. The method takes as a parameter integer that indicates the node's position which data you need to display. Example: list: 5 -> 8 -> 3 -> 10 display_at(1); // will display 5 display_at(4); // will display 10 void LinkedList::display_at(int pos) const{ // your code will go here }arrow_forwardJAVA plese Implement the indexOf method in the LinkedIntegerList class public int indexOf(int value); /** * Returns whether the given value exists in the list. * @param value - value to be searched. * @return true if specified value is present in the list, false otherwise. */ } public static void main(String[] args) { // TODO Auto-generated method stub SimpleIntegerListADT myList = null; System.out.println(myList); for(int i=2; i<8; i+=2) { myList.add(i); } System.out.println(myList.indexOf(44));arrow_forward
- Add more methods to the doubly linked list class then test them• search(e) // Return one node with 3 values (stuID, stuName, stuScore) which matches agiven key e (studentID).• addAfter(e, stuID, stuName, stuScore) //Add a new node with 3 values (stuID,stuName, stuScore) after the node with the key e (studentID).• removeAt(e) //Remove a node which matches a given key e (studentID)• count() //Return a number of nodes of list.• update(stuID, stuName, stuScore) //Update the values of one node first code below package DlinkedList;public class DLinkedList<A,B,C> { private Node<A,B,C> header; private Node<A,B,C> trailer; private int size; public DLinkedList() { header = new Node<>(null, null, null); trailer = new Node<>(null, null, null); header.setNext(trailer); trailer.setPrev(header); } public int getSize() { return size; } public boolean isEmpty() { return size==0; } public A…arrow_forwardSuppose that you have a singly linked list with five nodes and with head reference. Then the statement head = head.next will remove the first node of the linked list? a) true b) falsearrow_forwardWhich best describes this axiom: aList.getLength ( ) = ( aList.remove ( i ) ) . getLength ( ) + 1 You cannot remove an item from from existing list that is empty Removing an item from an existing list decreases its length by one Removing an item from an existing list increases the list size by one None of thesearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
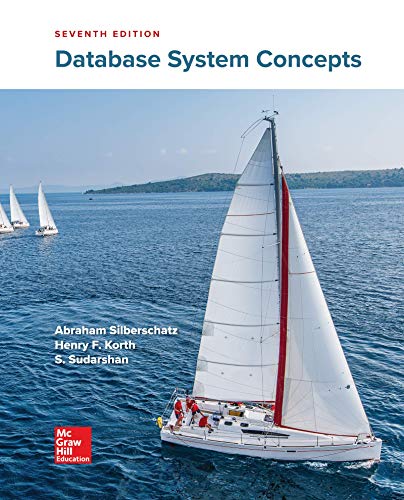
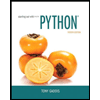
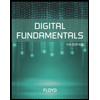
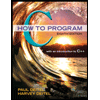
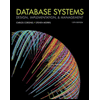
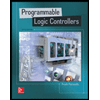