Java, the user selects an item from the text files ranging 1-15, repeats the loop until 'q'. the program provides a list of what they ordered including discounts, then calculates the total bill and how much they saved. The bottom is my the code I'm working on
Java, the user selects an item from the text files ranging 1-15, repeats the loop until 'q'. the program provides a list of what they ordered including discounts, then calculates the total bill and how much they saved.
The bottom is my the code I'm working on
class BillProcessor {
public static void prepareBill(LinkedHashMap<String, Integer> purchases, LinkedHashMap<String,Double>items,
LinkedHashMap<String,String> sales, ArrayList<String> itemNames){
double totalCost, totalDiscount, actualCost, discount; //note actualCost is the price of a single item
totalCost = 0.00;
totalDiscount = 0.00;
actualCost = 0.00;
discount = 0.00;
for(Map.Entry<String,Integer>set: purchases.entrySet()) {
if (sales.get(set.getKey()) != null) {
if (sales.get(set.getKey()).equals("bogo") == true) {
actualCost = set.getValue() * items.get(set.getKey());
totalCost += actualCost;
System.out.println("Item Name : " + set.getKey() + "\tItem Quantity(bogo) : " + (set.getValue() * 2
+ "\tItem Cost : " + actualCost));
} else {
discount = Double.valueOf(sales.get(set.getKey()));
actualCost = set.getValue() * (items.get(set.getKey()) - discount);
totalCost = totalCost + actualCost;
totalDiscount = totalDiscount + discount;
System.out.println("Item Name : " + set.getKey() + "\tItem Quantity(sale) : " + set.getValue()
+ "\tItem Cost : " + actualCost);
}
} else {
actualCost = set.getValue() * items.get(set.getKey());
totalCost += actualCost;
System.out.println("Item Name : " + set.getKey() + "\tItem Quantity : " + set.getValue()
+ "\tItem Cost : " + actualCost);
}
}
System.out.printf("Your total bill is $%.2f.\n", totalCost);
System.out.printf("You saved $%.2f by shopping with us today.", totalDiscount);
}
}
public class GarciaGroceryStore {
private static LinkedHashMap<String, Double> items;
private static LinkedHashMap<String, String> sales;
private static LinkedHashMap<String, Integer> purchases;
private static ArrayList<String>itemNames;
public static LinkedHashMap<String,Double> readItemsFromFile(String fname){
LinkedHashMap<String, Double> result = new LinkedHashMap<String, Double>();
itemNames = new ArrayList<String>();
try {
File file = new File(fname);
FileReader fr = new FileReader(file); //open the file
BufferedReader br = new BufferedReader(fr);
String line;
while((line = br.readLine()) != null) {
String item[] = line.split(" ");
result.put(item[0], Double.valueOf(item[1]));
itemNames.add(item[0]);
}
fr.close();
Collections.sort(itemNames);
return result;
} catch (IOException e){
e.printStackTrace();
}
return result;
}
public static LinkedHashMap<String, String> readSalesFromFile(String fname){
LinkedHashMap<String, String> result = new LinkedHashMap<String,String>();
try {
File file = new File(fname);
FileReader fr = new FileReader(file); // reads the file
BufferedReader br = new BufferedReader(fr);
String line;
while ((line = br.readLine()) != null) {
String item[] = line.split(" ");
result.put(item[0], item[1]);
}
fr.close();
return result;
} catch (IOException e) {
e.printStackTrace();
}
return result;
}
public static void printBanner() {
System.out.println("****************************************************************");
System.out.println("* CHARLIE'S PANTRY *");
System.out.println("****************************************************************");
System.out.println();
System.out.println("Welcome to your friendly neighborhood Charlie's Pantry. We sell\r\n"
+ "only the highest quality groceries and freshest produce around.\r\n"
+ "We have many great specials this week. The more you buy, the\r\n"
+ "more you save!\r\n"
+ "");
}
public static void showTheMenu() {
System.out.println("What would you like to buy?\n");
System.out.println("## Item Name Reg. Sale");
System.out.println("-------------------------------------------------------\n");
for (String item: itemNames) {
System.out.printf("%-10s %-10.2f %-10.2f", item, sales.get(item));
}
System.out.println("-------------------------------------------------------");
}
//The main
public static void main(String[] args) {
String choice;
char quit = 'q';
double totalCost, totalDiscount;
Scanner sc = new Scanner(System.in);
purchases = new LinkedHashMap<String, Integer>();
items = readItemsFromFile("items.txt");
sales = readSalesFromFile("sales.txt");
//present to the user the table
printBanner();
showTheMenu();
do {
System.out.print("");
//ask them to choose
System.out.println("Enter the number of your choice, or q to check out: ");
choice = (sc.nextLine()).charAt(0);
} while (quit != 'q');
sc.close();
BillProcessor.prepareBill(purchases, items, sales, itemNames);
System.out.println("\nThank you for your business. Come back soon!");
}
}
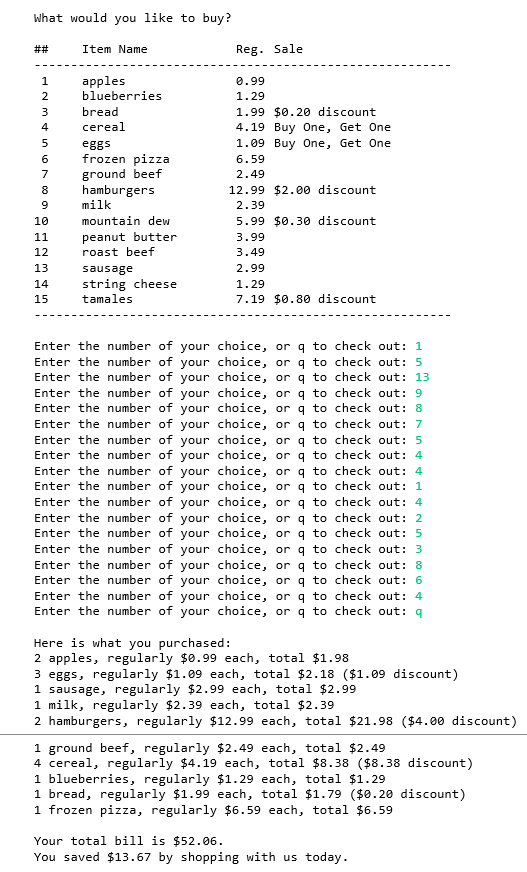
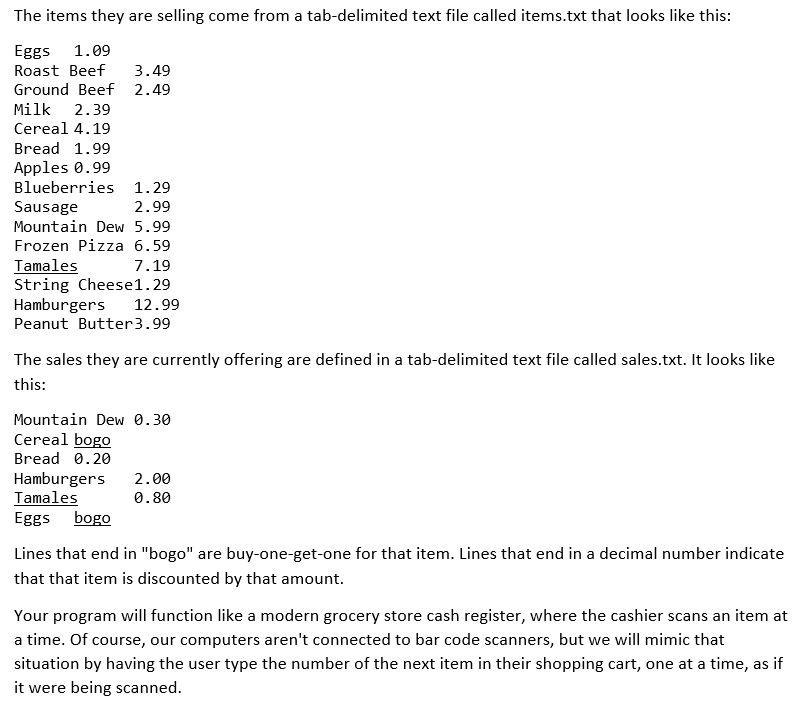

Step by step
Solved in 2 steps with 1 images

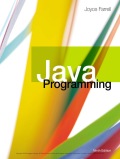
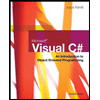
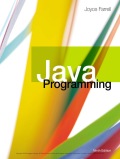
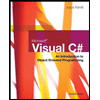