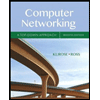
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
In python, please!
The code should contain __name__ == "__main__"
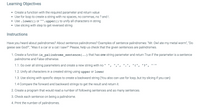
Transcribed Image Text:**Learning Objectives**
- Create a function with the required parameter and return value.
- Use for loop to create a string with no spaces, no commas, no ? and !.
- Use `.lower()` or `.upper()` to unify all characters in string.
- Use slicing with step to get reversed string.
**Instructions**
Have you heard about palindromes? About sentence palindromes? Examples of sentence palindromes: "Mr. Owl ate my metal worm", "Do geese see God?", "Was it a car or a cat I saw?" Please, help us check that the given sentences are palindromes.
1. Create a function `is_palindrome_sentence(..)` that has one string parameter and returns True if the parameter is a sentence palindrome and False otherwise.
1.1. Go over all string parameters and create a new string with no " ", ",", ".", "!", "?", "'"
1.2. Unify all characters in a created string using `upper` or `lower`.
1.3. Use slicing with specific steps to create a backward string (You also can use for loop, but try slicing if you can).
1.4. Compare the forward and backward strings to get the result and return it.
2. Create a program that would read a number of following sentences and as many sentences.
3. Check each sentence on being a palindrome.
4. Print the number of palindromes.
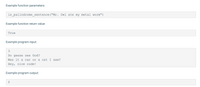
Transcribed Image Text:**Example function parameters:**
```plaintext
is_palindrome_sentence("Mr. Owl ate my metal worm")
```
**Example function return value:**
```plaintext
True
```
**Example program input:**
```plaintext
3
Do geese see God?
Was it a car or a cat I saw?
Hey, nice code!
```
**Example program output:**
```plaintext
2
```
**Explanation:**
This example demonstrates a function, `is_palindrome_sentence`, which checks if a given sentence is a palindrome. A palindrome is a word, phrase, or sequence that reads the same backward as forward, ignoring spaces, punctuation, and capitalization. The function takes a string as input and returns `True` if the sentence is a palindrome.
In the program input example, there are three sentences provided:
1. "Do geese see God?"
2. "Was it a car or a cat I saw?"
3. "Hey, nice code!"
The function evaluates these sentences, discovering that the first two are palindromes. Therefore, the program output is `2`, indicating that there are two palindrome sentences among the input.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- PYTHON question Question 1: Twenty-Twenty-Three Part 1: Come up with the most creative expression that evaluates to 2023, using only numbers and the [+, *, - ] operators. You should replace the underscores in return ______ with the expression that evaluates to 2023. def twenty_twenty_three(): """Come up with the most creative expression that evaluates to 2023,using only numbers and the +, *, and - operators. (no call expressions) >>> twenty_twenty_three() 2023 """ *** YOUR CODE HERE *** return _______ Part 2: Try to rewrite the same expression, this time entirely with call expressions (using function calls: add, mul, sub, etc… You can use from operators import add, mul, sub)arrow_forwardLAB ASSIGNMENTS IMPORTANT: you should complete the PDP thinking process for each program. Turn in items: 1) book_list.py: you can adapt your Chapter 4 book_list.py Lab program. The program summarizes costs of a book list. It uses all of our standard mipo_ex features. This program summarizes a book list. Enter the number of books that you need: In this version of the program you must use pylnputPlus functions to perform all the input validation and for the main() loop decision. Please enter a whole number: three Enter a number greater than 0: 3 Enter the name of book #1: The Mueller Report Enter cost of The Mueller Report, to the nearest dollar: Please enter a whole number: 18 Enter the name of book #2: Educated: A Memoir Adjust your program to allow book prices to include $ and cents. Restrict individual book prices to $100 maxium. Enter cost of Educated: A Memoir, to the nearest dollar: Please enter a whole number: 24 Enter the name of book #3: Becoming Enter cost of Becoming, to the…arrow_forwardIn Visual Studio using Console App (.NET Core) (4) In Visual Studio, create and test a console application that does the following: (1) Create a variable of the Integer data type, named marks; (2) Create a variable of the String data type, named grade; (3) Use the built-in function Console.ReadLine() to get the user's input for marks as follows (it is assumed that the user always inputs integers when testing this program): Console.WriteLine("Input the marks:") marks CType(Console.ReadLine(), Integer) (4) Convert marks into grade as follows using an If-Then-Elself statement: marks 90-100 85-89 80-84 77-79 73-76 70-72 67-69 63-66 60-62 50-59 0-49 grade A+ A A- B+ B B- C+ с C- D F (5) Display the value of grade in the console window using the following statement: Console.WriteLine("The grade is "& grade) Hint: The If-Then-Elself statement is a lengthy one and is similar to the following: If ((marks >=90) And (marks = 85) And (marks =0) And (marks<-49) Then grade "F" Elsearrow_forward
- 7 Select the value that is returned by the following expression. "abcd"[2:] Try to answer this question from your understanding and not typing the expression into the Python shell. It will help reinforce your understanding of basic Python expressions. Question 7 options: "ab" "bc" "cd" errorarrow_forwardTo remove the value of a variable but keeping the variable in PowerShell, we can use this cmdlet: Group of answer choices Delete-Value Clear-Variable Clear-Value Remove-Variablearrow_forwardAssume the following statement has been executed:number = 1234567.456Write a Python statement that displays the value referenced by the number variable formatted as1,234,567.5arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
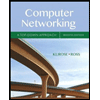
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
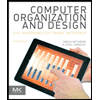
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
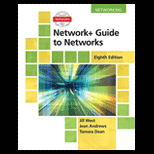
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
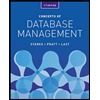
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
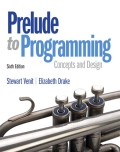
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
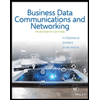
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY