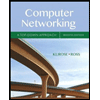
main()
Write a python program that displays simple rithmetic problems and asks users for the answer. the program has two functions:
mathQuiz(n)
Chooses two different rnadom int between 1 and n (inclusive)
randomly picks one of the following four arithmetic operators: +, -, *, %
Computes correct asnwer for chosen numbers and operator, but does not display it.
Displays the arithmetic problem using the two nmubers and the operators.
Waits for the user to enter an answer.
If user's answer is correct, print "Correct!" and return True
If user's answer is wrong, print "Wrong! Correct answer is:" with the correct answer and return False
main()
Intializes counters for score (number correct) and number of tries
Calls mathQuiz() with 20 to use for number
USe boolean value returned by mathQuiz() to see if user was right or wrong
Updaates user's score and number of attempts appropriately.
Asks user if they want to play again (y or n)
Validate user's response. Ask again if what user entered is not y or n
If user entered y, loops back for another math problems
If user entered n, "Your score: N correct in M attempts." and quit the program

Step by stepSolved in 4 steps with 2 images

- In C Languagearrow_forward***IN PSUEDOCODE ONLY - not a real programming language!!*** Question:A prime number is a number that is only evenly divisible by itself and 1. For example, the number 5 is prime because it can only be evenly divided by 1 and 5. The number 6, however, is not prime because it can be divided evenly by 1, 2, 3, and 6. Design a Boolean function called isPrime, that accepts an integer as an argument and returns True if the argument is a prime number, or False otherwise. Use the function in a program that prompts the user to enter a number and then displays a message indicating whether the number is prime. The following modules should be written: - getNumber, that accepts a Ref to an integer, prompts the user to enter a number, and accepts that input - isPrime, that accepts an integer as an argument and returns True if the argument is a prime number, or False otherwise - showPrime, that accepts an integer as an argument , calls isPrime, and displays a message indicating whether the…arrow_forwardPython Programming Only (PLEASE INCLUDE INPUT VALIDATION IF NEEDED) Write a function named maximum that accepts two integer values as arguments and returns the value that is the greater of the two. For example, if two numbers are one big and one small the function should return the bigger number. Use the function in a program that prompts the user to enter two integer values. The program should display the value that is the greater of the two.arrow_forward
- PART I: Functions – Math Test Write the Flowchart and Python code for the following programming problem based on the pseudocode below. Write a program that will allow a student to enter their name and then ask them to solve 10 mathematical equations. The program should display two random numbers that are to be added, such as: 247 + 129 The program should allow the student to enter the answer. The program should then display whether the answer was right or wrong, and accumulate the correct values. After the 10 questions are asked, calculate the average correct. Then display the student name, the number correct, and the average correct in both decimal and percentage format. In addition to any system functions you may use, you might consider the following functions: A function that allows the student to enter their name. A function that gets two random numbers, anywhere from 1 to 500. A function that displays the equation and asks the user to enter their answer. A function that…arrow_forwardWrite a program in MIPS assembly language to convert an ASCII number string containing positive and negative integer decimal strings, to an integer. Your program should expect register $aO to hold the address of a null-terminated string containing some combination of the digits 0 through 9. Your program should compute the integer value equivalent to this string of digits, then place the number in register $v0. If a non-digit character appears anywhere in the string, your program should stop with the value-1 in register $v0. For example, if register $a0 points to a sequence of three bytes 50ten,52ten,0ten(null-terminated string "24"). then when the program stops, register $v0 should contain the value 24tenarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
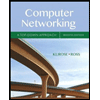
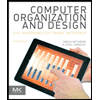
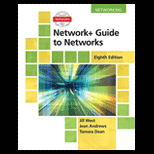
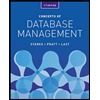
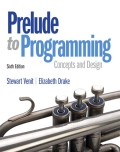
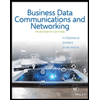