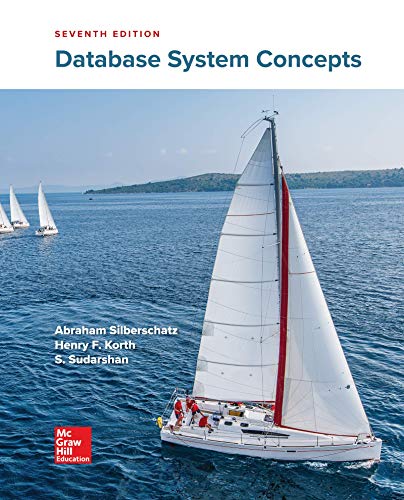
Concept explainers
make it so the python code becomes a dynamic bar chart using from matplotlib import animation. Make it so the bar moves.
This is the code I have so far for the python code
import random
import sys
import matplotlib.pyplot as plt
import pandas as pd
def roll_dice():
die1 = random.randrange(1, 7)
die2 = random.randrange(1, 7)
return (die1, die2)
def display_dice(dice):
die1, die2 = dice
print(f'Player rolled {die1} + {die2} = {sum(dice)}')
# List that stores number of wins on every roll
winList = []
# List that stores number of losses on every roll
lossList = []
# List that stores label indexes of horizontal bar plot
ylabel = []
# 1
# number of games of craps
n = int(input("Enter number of games: "))
# Iterating 13 times
# Because it is mentioned in the question that plot should have 13
# horizontal bars for wins, and 13 horizontal bars for losses.
for roll in range(13):
ylabel.append('Roll ' + str(roll + 1))
# variables that keep track of wins and losses on every roll
# for the given number of games of craps
wins = losses = 0
for i in range(n):
die_values = roll_dice()
display_dice(die_values)
sum_of_dice = sum(die_values)
if sum_of_dice in (7, 11):
game_status = 'WON'
wins += 1
elif sum_of_dice in (2, 3, 12):
game_status = 'LOST'
losses += 1
else:
game_status = 'CONTINUE'
my_point = sum_of_dice
print('Point is', my_point)
while game_status == 'CONTINUE':
die_values = roll_dice()
display_dice(die_values)
sum_of_dice = sum(die_values)
if sum_of_dice == my_point:
game_status = 'WON'
wins += 1
elif sum_of_dice == 7:
game_status = 'LOST'
losses += 1
if game_status == 'WON':
print('Player wins')
else:
print('Player loses')
winList.append(wins)
lossList.append(losses)
# 2
data = {'Wins': winList, 'Losses': lossList}
df = pd.DataFrame(data, columns=['Wins', 'Losses'], index=ylabel)
df.plot.barh()
plt.title('Game of Craps')
plt.ylabel('Roll')
plt.xlabel('Number of Games')
plt.show()

//Python code
import random
import sys
import matplotlib.pyplot as plt
import pandas as pd
from matplotlib import animation
def roll_dice():
die1 = random.randrange(1, 7)
die2 = random.randrange(1, 7)
return (die1, die2)
def display_dice(dice):
die1, die2 = dice
print(f'Player rolled {die1} + {die2} = {sum(dice)}')
# List that stores number of wins on every roll
winList = []
# List that stores number of losses on every roll
lossList = []
# List that stores label indexes of horizontal bar plot
ylabel = []
# 1
# number of games of craps
n = int(input("Enter number of games: "))
# Iterating 13 times
# Because it is mentioned in the question that plot should have 13
# horizontal bars for wins, and 13 horizontal bars for losses.
for roll in range(13):
ylabel.append('Roll ' + str(roll + 1))
# variables that keep track of wins and losses on every roll
# for the given number of games of craps
wins = losses = 0
for i in range(n):
die_values = roll_dice()
display_dice(die_values)
sum_of_dice = sum(die_values)
if sum_of_dice in (7, 11):
game_status = 'WON'
wins += 1
elif sum_of_dice in (2, 3, 12):
game_status = 'LOST'
losses += 1
Step by stepSolved in 4 steps

Is there a way that you can run the code? And paste the output. Because when ever I run it. It doesn't work.
when ever I run the code it says ax.barh(df.index, df['Wins'], color='blue')
NameError: name 'ax' is not defined. How do I fix it.
Is there a way that you can run the code? And paste the output. Because when ever I run it. It doesn't work.
when ever I run the code it says ax.barh(df.index, df['Wins'], color='blue')
NameError: name 'ax' is not defined. How do I fix it.
- 1. The main function creates 5 pixels with random red, green, and blue values. Complete the id_color function to return “red" if the red value is greater than the green and blue, "green" if the green value is greater than the red and blue, and “blue" if the blue value is greater than the red and green. If there is no clear maximum value (for example, if the red and green values are the same) return None. Do not change the main function. Save & Run 10/22/2020, 3:44:29 PM - 4 of 4 Show in CodeLens 1 import image 2 import random 4 def id_color(p): '''Returns the dominant color of pixel p''' pass #Your code here to determine the dominant color and return a string identif #Hint: get the red, green & blue values and use an if statement to determine wh 7 8. 9. 10 def main(): '''Controls the program' for i in range(5): 11 12 #Loop 5 timesarrow_forward1. In each iteration of a loop, generate a random number, and use it to determine some attribute of what is drawn in that iteration. For example, draw one shape multiple times in random locations and/or at random sizes OR 2. write a triply nested for loop to add even more complexity to your drawing than with a doubly nested looparrow_forwardPython code Upload a picture of your code(please don’t type it in here).arrow_forward
- #create a duplicate of the image for y in range (image.getHeight()-1): for x in range (1, image.getWidth () -1): #Row major traversal of each pixel #display in the image oldPixel=image.getPixel(x,y) #Get the pixel present at current place leftPixel=image.getPixel(x-1,y) #Get left pixel. bottomPixel=image.getPixel (x, y+1) #Get bottom pixel oldLum-average (oldPixel) #calcule luminance of current pixel leftLum-average (leftPixel) #calculate luminance of left pixel bottomLum=average (bottomPixel) #calculate luminance of right pixel if abs (oldLum-leftLum) >threshold or\ abs (oldLum-bottomLum) >threshold: new.setPixel (x,y, sharpen (old, degree)) return new #3main function def main (): #Input image image=Image ("testImage.gif") image.draw () #Call the method, edge detection out=edge_detection (image, 80,10) out.draw () if main () name main ": ==arrow_forwardDO NOT COPY FROM OTHER WEBSITES Code with comments and output screenshot is must for an Upvote. Thank you!!!arrow_forwardThe progam below was created by your experts and suppose create a pattern using nested loops. Your initial needs to appear at least eight times in the pattern and pattern doesn't matter. and it was good created but i received comment saying: - this is not using nested loops. Can someone please fix it the code below by using NESTED LOOPS? import turtle # Setting background color screen = turtle.Screen()screen.bgcolor("skyblue") #drawing letter 'M' in different colors turtle.penup()turtle.pensize(9) turtle.goto(-300,50)turtle.right(90)turtle.forward(300) for i in range (1,4):turtle.color("dark blue")turtle.pendown()turtle.backward(60)turtle.left(45)turtle.forward(40)turtle.left(90)turtle.forward(40)turtle.right(135)turtle.forward(60)turtle.backward(60) for i in range (1,4):turtle.color("red")turtle.pendown()turtle.backward(60)turtle.left(45)turtle.forward(40)turtle.left(90)turtle.forward(40)turtle.right(135)turtle.forward(60)turtle.backward(60) for i in range…arrow_forward
- programming in an object-oriented style using Java Use the composite pattern to model your scene. It may be an image, a simulator, a movie, a gaming, or something else entirely.arrow_forwardUse Python Codehow to filter dataframes using python . For example I have a student grade csv table, first, I need to read the csv using pandas library then I want to visualize my student scores (using matplotlib, seaborn, etc) but I only want to visualize those whose scores are 60 and above. so how do i do the code in python.Exampe of Student grades table (csv) : Student name Score Jojo 90 Lili 65 Bibi 50 Sarah 40 Rajah 100 Albert 80 Cherry 75 Jamie 85 So , based on the table above, what doesn't need to be visualized is Bibi and Sarah . Hope you can help me :)arrow_forwardFinal help needed for Python Turtle, does my code have everything required, and if not can you help make the changes needed. 1. An additional option to generate another island if the user does not like the one they have (loops until they are satisfied) and have the GUIs bigger.2. Does my code have two or more functions (I dont know if the drawing only counts as one)?3. Does my code have atleast one list, dictionary, or set (if not reorganize/add one)?4. Need help exporting the finished turtle image into a file on desktop (yes/no option input by user) that can be accessed, or png (In ###'s I saved the broken code that i dont know how to fix) My code: import turtleimport randomimport tkinter as tkfrom tkinter import simpledialog### OPTIONAL IMAGE CONVERTER, REMOVE ###'S TO USE###from PIL import Image# Colors:ocean = "#000066"sand = "#ffff66"grass = "#00cc00"lake = "#000066"mountain_color = "#808080" mountain_positions = []def draw_irregular_circle(radius, line_color, fill_color,…arrow_forward
- Create a vector x which ranges in values from -180 to 180 in steps of 10. Then let y = x. These values are in degrees. Find a variable z = cosd(x)’*sind(y); Use surf to plot the surface plot, and label using commands xlabel, ylabel, and zlabel. Add a title.arrow_forwardpython we have a package, Shapes, that contains the modules square.py, rectangle.py, and circle.py. choose correct statement to import rectangle.py? import Shapesimport rectangle import Shapes.rectangle import rectangle.py import Shapes.rectangle.pyarrow_forwardIn python, write a function own_filter where it can flip an image vertically or set an image to a red color.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
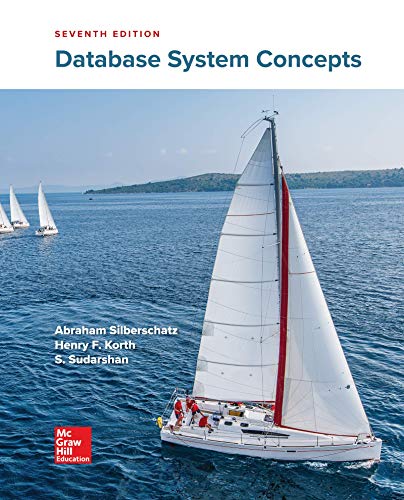
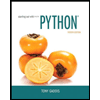
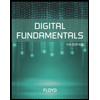
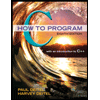
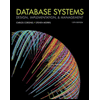
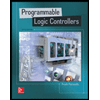