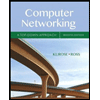
Many computer applications involve searching through a set of data and sorting the data. A number of effi cient searching and sorting algorithms have been devised in order to reduce the runtime of these tedious tasks. In this problem we will consider how best to parallelize these tasks.
Consider the following binary search
BinarySearch(A[0..N−1], X) {
low = 0
high = N −1
while (low <= high) {
mid = (low + high) / 2
if (A[mid] >X)
high = mid −1
else if (A[mid] <X)
low = mid + 1
else
return mid // found
}
return −1 // not found
}
Assume that you have Y cores on a multi-core processor to run BinarySearch. Assuming that Y is much smaller than N, express the speedup factor you might expect to obtain for values of Y and N. Plot these on a graph.
Next, assume that Y is equal to N. How would this affect your conclusions in your previous answer? If you were tasked with obtaining the best speedup factor possible (i.e., strong scaling), explain how you might change this code to obtain it.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- Coding in Python, we have the function to perform this task, V col_Hdeg whichinputs the adjacency matrix A of the graph and initializes the array vert_color s tohold vertex colors. It then calculates the degree of each vertex and stores it in thearray degrees.The while loop iterates until all vertices are colored and at each iteration, thevertex v with the highest degree is colored. The array temp is used to find the currentcolors of the neighbors of vertex v and an unused smallest color is determined aftersorting this array.arrow_forwardThe algorithm below searches for the maximum in an input array A. Assume A is a random sequence containing n distinguishable real numbers, what is the probability for line #4 to be executed exactly once? (The phrase "random sequence" here means the n numbers can appear in any order with equal chance.) mymax(A, n) { 1: max = A[1]; 2: for i = 2:n 3: if A[i] > max 4: max = A[i); 5: end; 6: end; 7: return max; }arrow_forwardCan someone help me with this algorithm? No coding neededarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
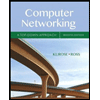
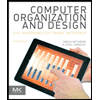
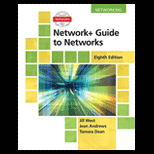
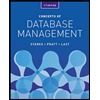
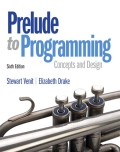
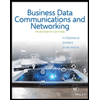