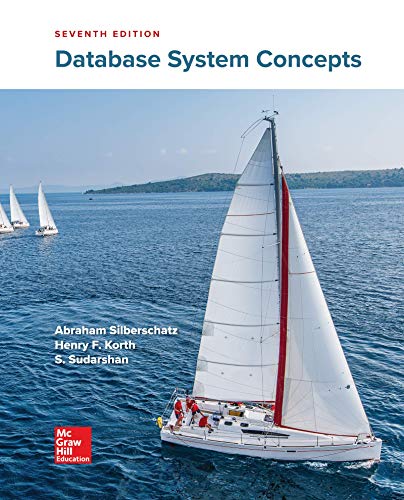
Concept explainers
Instructions:
IMPORTANT: This is a continuation of the previous part of the project and assumes that you are starting with code that fulfills all requirements from that part of the project.
Modify the your code from the previous part of the project to make it modular.
In addition to the main method, your code must include the following static methods:
Method 1 - displayTitle
A method that creates a String object in memory to hold the text “
Method 2 – getResolutionString
A method that accepts an integer value (1, 2, 3, or 4) that denotes the monitor resolution. The method should return the appropriate String representation of the monitor resolution. For example, if the method is passed an integer value of 1, it should return a String with a value of “1280 x 720”. (See Step 4 of Project 1)
Method 3 – getMultiplierValue
A method that accepts an integer value (1, 2, 3, or 4) that denotes the monitor resolution and returns the appropriate “multiplier” value. (See Step 5 of Project 1)
Method 4 - calculatePerformanceScore
A method that accepts the GPU clock speed, CPU clock speed, the number of cores, and the multiplier value, and calculates and returns the performance score. (See Step 6 of Project 1)
Method 5 – getRecommendedQuality
A method that accepts a performance score as an argument and returns the recommended graphics quality. (See Step 7 of Project 1)
Method 6 - displayInformation
A void method that prints out the information for a single computer. The output should look exactly the same as the output for Project 2. The method should accept only the arguments necessary to print out the information. (See Step 9 of Project 1)
Each of the above methods should match the naming and parameter requirements exactly. Do not rename the method, or add/remove arguments to the method.
At the end of the output, the program should display the highest and lowest performance score.
The code that you submit should be thoroughly documented with comments where appropriate (this includes documentation for each method).
Sample Input and Output (user input is in bold) - The output of your program should match the formatting and spacing exactly as shown
How many computers are being processed? 3
Computer Hardware Graphics Quality Recommendation Tool
Please enter the clock speed (in Megahertz) of your graphics card: 1000
Please enter the clock speed (in Megahertz) of your processor: 3000
Please enter the number of cores of your processor: 4
What is the resolution of your monitor?
1. 1280 x 720
2. 1920 x 1080
3. 2560 x 1440
4. 3840 x 2160
Please select from the options above: 1
GPU Clock Speed: 1000 MHz
CPU Clock Speed: 3000 MHz
Number of cores: 4
Monitor Resolution: 1280 x 720
Performance Score: 17,000.000
Recommended Graphics Quality: High
Please enter the clock speed (in Megahertz) of your graphics card: 1250
Please enter the clock speed (in Megahertz) of your processor: 3200
Please enter the number of cores of your processor: 4
What is the resolution of your monitor?
1. 1280 x 720
2. 1920 x 1080
3. 2560 x 1440
4. 3840 x 2160
Please select from the options above: 3
GPU Clock Speed: 1250 MHz
CPU Clock Speed: 3200 MHz
Number of cores: 4
Monitor Resolution: 2560 x 1440
Performance Score: 10,477.500
Recommended Graphics Quality: Unable to Play
Please enter the clock speed (in Megahertz) of your graphics card: 1800
Please enter the clock speed (in Megahertz) of your processor: 4300
Please enter the number of cores of your processor: 4
What is the resolution of your monitor?
1. 1280 x 720
2. 1920 x 1080
3. 2560 x 1440
4. 3840 x 2160
Please select from the options above: 2
GPU Clock Speed: 1800 MHz
CPU Clock Speed: 4300 MHz
Number of cores: 4
Monitor Resolution: 1920 x 1080
Performance Score: 19,650.000
Recommended Graphics Quality: Ultra
The highest performance score was: 19,650.000
The lowest performance score was: 10,477.500

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Methods that start with an underscore are considered internal methods, that is they are not there for the user to call from outside the class. True Falsearrow_forward1- A variable of class type (i.e., object) always require the same amount of memory to store its values? False True 2- A class that contains no methods (other than constructors) that change any of the data in its object is called as: Mutable class Immutable class Anonymous class Class invariantarrow_forwardGUI calculator in python - The user enters two integers into the text fields. - When the Add button is pressed, the sum of values in the text fields are shown after the Equals: as a label. - The Clear button clears the values in the text fields and the result of the previous calculation. The cleared values can be blank or zero. - The Quit button closes the GUI window.arrow_forward
- Draw Design Layout References Mailings Review View Help Create a Java program and name your file: FIRSTNAME. java (for example, lohn.java). Work on the following: Create three interfaces with the names "InterfaceOne," "IrnterfaceTwo," and "Interfacelhree" In the first interface, declare a method (signature only) with a name "updateGear()." In the second interface, dedare a method (signature only) with a name "accelerate()." In the third interface, dedare a method (signature only) with a name "pusherake()." Create two classes Car and Truck that implement these three interfaces at one time. Define a new method "currentSpeed()" in both the classes to find the current speed after the brake. • Define all the three methods inside each class. The data to these methods will be provided during the object creation. Invoke the two objects with a name c1 of class Car and t1 of class Truck. • After creating the objects, call all the three methods defined above in both the classes. Pass any of the…arrow_forwardJava Programarrow_forwardMethods with an empty parameter list and do not return a value: [Questions 1-8 required] You invoke a method by its name followed by a pair of brackets and the usual semi-colon Write a method called DisplayPersonalInfo(). This method will display your name, school, program and your favorite course. Call the DisplayPersonalInfo() method from your program Main() method Write a method called CalculateTuition(). This method will prompt the user for the number of courses that she is currently taking and then calculate and display the tuition cost. (cost = number of course * 569.99). Call the CalculateTuition() method two times from the same Main() method as in question 1. Write a method call CalculateAreaOfCircle(). This method will prompt the user for the radius of a circle and then calculate and display the area.[A = πr2].Call the CalculateAreaOfCircle() method twice from the same Main() method as in question 1. Use Math.Pi for the value of π Write a method call…arrow_forward
- Instructions: 1. Late submission is not accepted. 2. Variables name: 10 points off if you do not follow this step. Choose meaningful and descriptive names. No x, y . etc. ... Use lowercase. If the name consists of several words, concatenate all in one, use lowercase for the first word, and capitalize the first letter of each subsequent word in the name. For example, the variables radius and area, and the method computeArea. 3. File type: only JAVA file is allowed. Name it as Assignment2. Java 4. Link to online compiler is not allowed. 5. Please add the appropriate comments for each step you add. In the top of the program, add your name, time of starting writing the code and title of the assignment. Extra information would be great. 6. The submission can be in two separate java files or one java file that has one main function, has the run of two programs. Both answers of two programs should be visible not in the comments. 7. Run your program many times, if it is has syntax errors, you…arrow_forwardIN PYTHON PLEASE, THANK YOU!!!arrow_forwardJava Program - GUI Number Guessing Look at the code, notice that the actionPerformed method is not complete. It just contains code that will print to the console when buttons are pressed. Make the following modifications to the code. When the Higher button is pressed invoke this.guesser.higher(), and then put the new guess into the this.guessField When the Lower button is pressed invoke this.guesser.lower() and then put the new guess into the this.guessField When the Reset button is pressed, invoke this.guesser.reset() and then put the new guess into the ghis.guessField When the Correct button is pressed, exit the app using System.exit(0). Wrap the invocation of lower() and higher() in try catch blocks that catch NumberGuesserIllegalStateExceptions. Show a JOptionPane that alerts the user that you are onto their schemes. Change the guessing algorithm from random-guess to binary search. You can do this by changing the object created for the guesser to a plain old NumberGuesser. It…arrow_forward
- A method CAN Not be invoked using a variable that is initialized to null. True Falsearrow_forwardCharacteristics of a method: • contains code that performs task or set of tasks • allows code method coded once, called many times • facilitates the design, implementation, operation and maintenance of programsarrow_forwardPythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
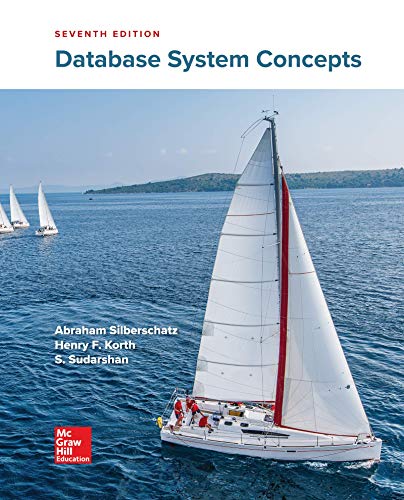
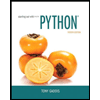
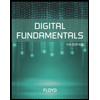
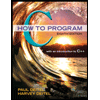
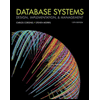
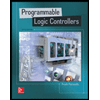