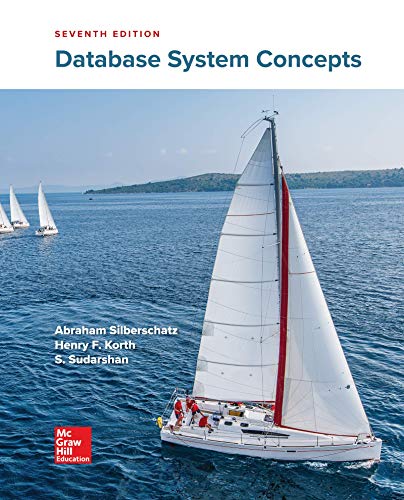
Microprocessor lab tasks -
Solve the problems in assembly language using emu8086
solution format
.MODEL SMALL
.STACK 100H
.DATA
; DEFINE YOUR VARIABLES HERE
.CODE
MAIN PROC
MOV AX, @DATA
MOV DS, AX
; YOUR CODE STARTS HERE
; YOUR CODE ENDS HERE
MOV AX, 4C00H
INT 21H
MAIN ENDP
END MAIN
Collapse
:white_tick:
1
Problems :
Task 01
a program that takes in 3 digits as input from the user and finds the
maximum Sample input:
1st input: 1
2nd input: 2
3rd input: 3
Sample Output:
3
Task 02
Take two digits as input from the user and multiply them. If the result is divisible by 2 and
3 both, print "Divisible". Otherwise, print "Not divisible"
Sample input:
1st input: 5
2nd input: 6
Result is 30
Sample Output:
Divisible
Sample input:
1st input: 5
2nd input: 2
Result is 10
Sample Output:
Not divisible

Step by stepSolved in 2 steps with 1 images

- Dynamic memory allocation is simplified here.arrow_forwardExplain the concept of variable lifetimes and how garbage collectors manage them in languages like Java and Python.arrow_forwardAssembly language 64-bit code The program will prompt the user for their name and then say hello to them using their name. For example, the interactive console of Repl.it might look like: > bash main.shEnter your name: *Camila *Hello, Camila> You will not have a library of routines to call upon for the printing and reading. You are manufacturing the complete setup and syscall. After you complete the reading, remember that .bss is for your variables that will receive data and .data is for fixed information, like prompts.arrow_forward
- ASSEMBLY Local variables are stored on the runtime stack, at a higher address than the stack pointer. True Falsearrow_forwardSelect common examples of when an assembly programmer would want to use the stack: to pass arguments to save return address for CALL local variables temporary save area for registers applications which have FIFO nature, such as customers waiting in a bank queuearrow_forward// main module Module main() // Local variables Declare Integer num1, num2, sum, answer // Get numbers Set num1 = random(1, 100) Set num2 = random(1, 100) Set sum = getSum(num1, num2) // Get user answer Call getAnswer(answer) // display result Call showResult(sum, answer) End Module // The getAnswer module gets user answer Module getAnswer(Integer Ref inputAnswer) Display “Enter sum of numbers: “ Input inputAnswer End Module // The showResult module tells if user answer is correct or not Module showResult (Integer sum, answer) If sum == answer Then Display "Correct answer – Good Work!" Else Display "Correct answer is: ", sum End If End Module // The getSum function adds 2 numbers. Function Integer getSum(Integer num1, num2) Declare Integer result Set result = num1 + num2 Return result flowchart for this pleasearrow_forward
- Coprocessors: What precisely are they?arrow_forwardA program becomes a process when it is an active entity loaded in main memory. max 0 ↓ 介 What region is used for variables local to a function? no option is correct data stack text heap sparse address spacearrow_forwardAs a program is started, it allocates memory (address space) to store its many components, such as its code, data, and data structures (stack, heap). Talk about the different applications of stacks and heaps, and how many of them a single process may have.arrow_forward
- Example: The Problem Input File Using C programming language write a program that simulates a variant of the Tiny Machine Architecture. In this implementation memory (RAM) is split into Instruction Memory (IM) and Data Memory (DM). Your code must implement the basic instruction set architecture (ISA) of the Tiny Machine Architecture: //IN 5 //OUT 7 //STORE O //IN 5 //OUT 7 //STORE 1 //LOAD O //SUB 1 55 67 30 55 67 1 LOAD 2- ADD 3> STORE 4> SUB 5> IN 6> OUT 7> END 8> JMP 9> SKIPZ 31 10 41 30 //STORE O 67 //OUT 7 11 /LOAD 1 //OUT 7 //END 67 70 Output Specifications Each piece of the architecture must be accurately represented in your code (Instruction Register, Program Counter, Memory Address Registers, Instruction Memory, Data Memory, Memory Data Registers, and Accumulator). Data Memory will be represented by an integer array. Your Program Counter will begin pointing to the first instruction of the program. Your simulator should provide output according to the input file. Along with…arrow_forwardWRITE THE FOLLOWING PROGRAM IN HIGH LEVEL ASSEMBLY LANGUAGE (HLA)arrow_forwardPLEASE DON'T USE STRUCTS Using C programming language write a program that simulates a variant of the Tiny Harvard Architecture. In this implementation memory (RAM) is split into Instruction Memory (IM) and Data Memory (DM). Your code must implement the basic instruction set architecture (ISA) of the TinyMachine Architecture: 1 -> LOAD 2->ADD 3->STORE 4 -> SUB 5 ->IN 6-> OUT 7-> END 8 -> JMP 9 -> SKIPZ Each piece of the architecture must be accurately represented in your code (Instruction Register, Program Counter, Instruction Memory (IM), MAR1, MDR-1(MAR-1 and MDR-1 are connected to the IM). Data Memory, MAR-2, MDR2 (MAR-2 and MDR-2 are connected to the DM), and Accumulator. Instruction Memory will be represented by an integer array and each instruction will use 2 elements of the array(one for OP and the other one for address) Data Memory will be represented by an integer array and each data value uses an element of the DM array. Your Program Counter will begin pointing to the first…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
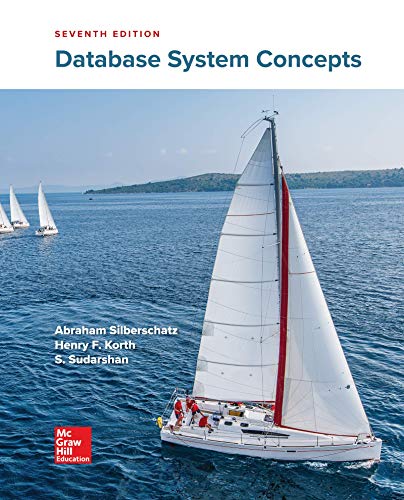
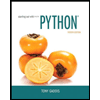
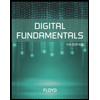
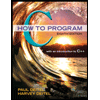
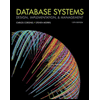
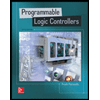