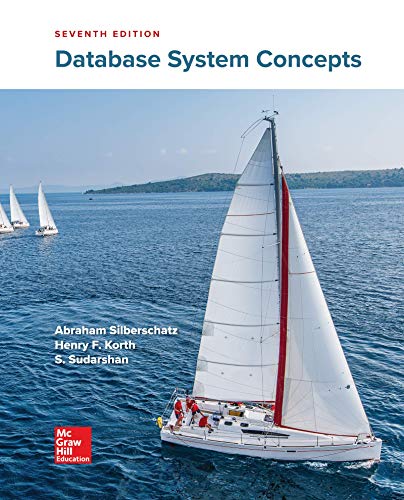
Modify the code on part-1.php, as indicated below.
a. Create the additional properties for the class Customer:
i. address
ii. telephone
b. Set an appropriate value for each of the newly created property, address and telephone,
in the customer object
c. Set an appropriate value for forename and surname in the customer object.
d. Display the customer’s forename and surname before their email. Both forename and
surname should be on the same line
e. Display the customer’s address on its own line, after their email. Capture your output
with a screenshot
f. Use the withdraw method to withdraw $100 for an object of the class, Account
g. Update your code to display the new balance. Capture your output with a screenshot
------
<?php
class Customer//This comment is used to indicate that the a class called 'Customer' is being created
{
public string $forename;
public string $surname;
public string $email;
public string $password;
}
class Account //This a class named, Account
{
public int $number;
public string $type;
public float $balance;
}
public function withdraw(float $amount): float
{
$this->balance -= $amount;
return $this->balance;
}
$customer = new Customer();//An instance of 'customer' class is created using the 'new' operator
$account = new Account(); //same is done with the 'Account' class
$customer->email = 'ivy@eg.link'; //Here, the email property of the customer object is given a value
$account->balance = 1000.00;// The account property of the customer object is given a value
?>
<?php include 'includes/header.php';//This includes the file name header.php ?>
<p>Email: <?= $customer->email ?></p>
<p>Balance: $<?= $account->balance ?></p>
<?php include 'includes/footer.php';//This includes the file name footer.php ?>

Step by stepSolved in 3 steps with 2 images

- JAVA Code Write a complete UML class diagram for the class described below. Use a line of minus signs to separate sections. Don't worry about drawing a box or centering any text. Do include all proper UML notation. Your need to create an instantiable class that represents a asteroid heading towards Earth. This class should be named Asteroid. It will contain three fields: a string named name and a mass and velocity, measured in kg and m/s respectively, each of which can accept numbers with decimal points. An observatory wants to know how much kinetic energy each asteroid will have as it passes or hits the earth. The class needs one constructor that sets the values of all the fields. The class needs the following public methods: Getters and setters for all fields. A method called getKineticEnergy that calculates and returns the kinetic energy of the asteroid. (Kinetic energy is calculated as 0.5 x mass x velocity2.) Standard methods: toString, equals, and hashCode where equals and…arrow_forwardCreate a java class called product with three data elements: name, price peer unit and quantity . Accessors and Mutators methods and at least three Constructors. In Main Java application call the product class to create at least three objects each with different constructor ,calculate the cost of each product object and the total price and print out the data about the product objects.arrow_forwardFor the Point2D data type, create three static comparators: one that compares points based on their x, y, and distance from the centre, and the other two that compare points based on their respective coordinates. For the Point2D data type, create two non-static comparators: one that compares objects based on how far they are from a given point, and the other that compares objects based on how far they are from a given point polarly.arrow_forward
- 1. Create a class called Triangle that models triangles using three points (the vertices) for the x's and three points for the y's. The vertices will be of type double. It will have two constructors: one by default and one that creates a triangle from its three vertices. The class will also include a method to calculate the area and another to calculate the perimeter of the triangle. Attributes are of type public. Write here the class diagram and class code in Javaarrow_forwardConsider an online holiday booking system. The users can register to book a holiday package. Currently, the design model includes the classes: User, Package and Family_Package. Which of the following is correct about a Package object in the object-oriented software design? Select one: O a. A Package object's attributes should always be made public and directly accessible. O b. A Package object contains a set of attributes only. O c. A Family Package object contains a set of methods only. O d. A Package object is one instance of its class.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
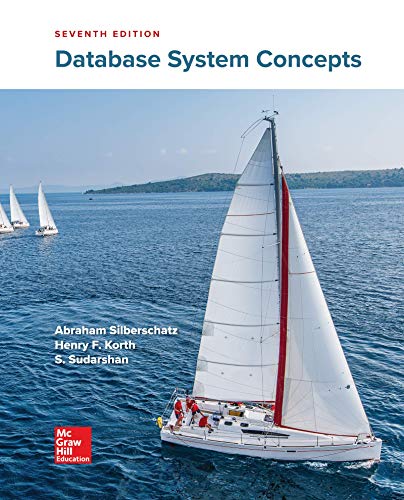
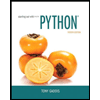
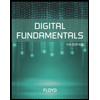
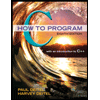
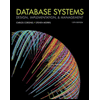
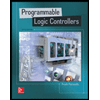