Modify the remove method in the UnorderedList class as follows. When the item is found in the UnorderedList, remove the item from the linked list and return а. True. b. When the item is NOT found in the UnorderedList, return False. Modify the UnorderedList class as follows. a. Implement the index(self,item) method. i. When the item is found, this method should return the position of the item in the UnorderedList. Note: the item at the head of the list is at position zero, the next item is at position one, and so on. ii. When the item is NOT found, this method should return -1. b. Implement the insert(pos,item) method. i. When pos is not valid, return False. Note: the pos value is invalid if it is less than zero or greater than size(). ii. Otherwise, insert the item at the pos position so the item just inserted is at this index position after the insert has completed. Note: the item at the head of the list is at position zero, the next item is at position one, and so on. In your source code file, add test code to test your modifications to the UnorderedList class. a. Think about the test cases you need to implement for each modification you made. Be sure to apply separation of concerns and design for reuse when developing vour test code. b.
Modify the remove method in the UnorderedList class as follows. When the item is found in the UnorderedList, remove the item from the linked list and return а. True. b. When the item is NOT found in the UnorderedList, return False. Modify the UnorderedList class as follows. a. Implement the index(self,item) method. i. When the item is found, this method should return the position of the item in the UnorderedList. Note: the item at the head of the list is at position zero, the next item is at position one, and so on. ii. When the item is NOT found, this method should return -1. b. Implement the insert(pos,item) method. i. When pos is not valid, return False. Note: the pos value is invalid if it is less than zero or greater than size(). ii. Otherwise, insert the item at the pos position so the item just inserted is at this index position after the insert has completed. Note: the item at the head of the list is at position zero, the next item is at position one, and so on. In your source code file, add test code to test your modifications to the UnorderedList class. a. Think about the test cases you need to implement for each modification you made. Be sure to apply separation of concerns and design for reuse when developing vour test code. b.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help with the code. Can anyone can help me write the code.
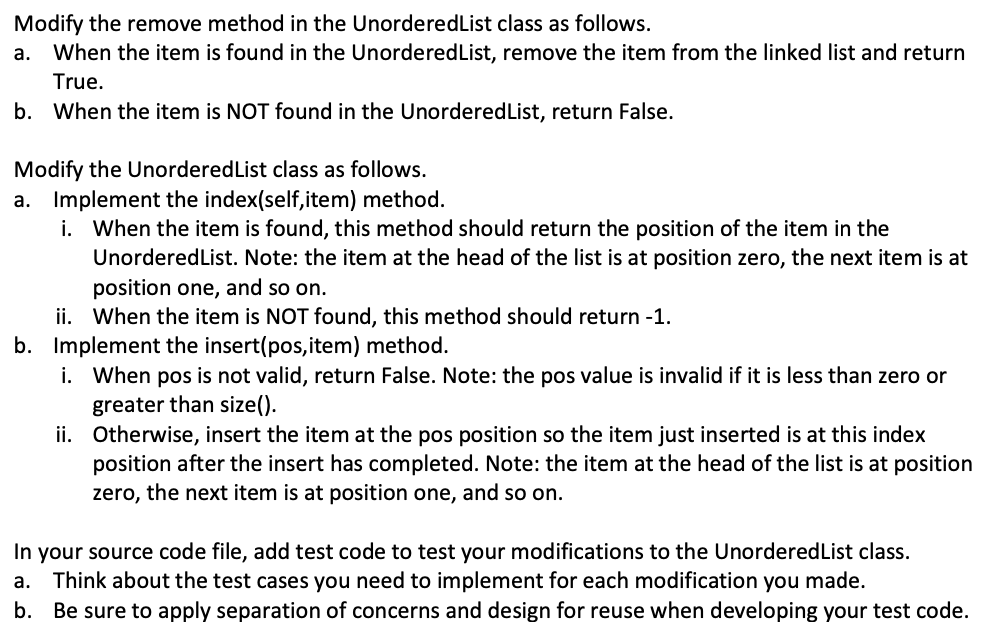
Transcribed Image Text:Modify the remove method in the UnorderedList class as follows.
When the item is found in the UnorderedList, remove the item from the linked list and return
а.
True.
b. When the item is NOT found in the UnorderedList, return False.
Modify the UnorderedList class as follows.
Implement the index(self,item) method.
i. When the item is found, this method should return the position of the item in the
UnorderedList. Note: the item at the head of the list is at position zero, the next item is at
position one, and so on.
ii. When the item is NOT found, this method should return -1.
b. Implement the insert(pos, item) method.
i. When pos is not valid, return False. Note: the pos value is invalid if it is less than zero or
а.
greater than size().
ii. Otherwise, insert the item at the pos position so the item just inserted is at this index
position after th
zero, the next item is at position one, and so on.
nsert has completed. Note: the item at the head of the list is at position
In your source code file, add test code to test your modifications to the UnorderedList class.
Think about the test cases you need to implement for each modification you made.
Be sure to apply separation of concerns and design for reuse when developing your test code.
а.
b.
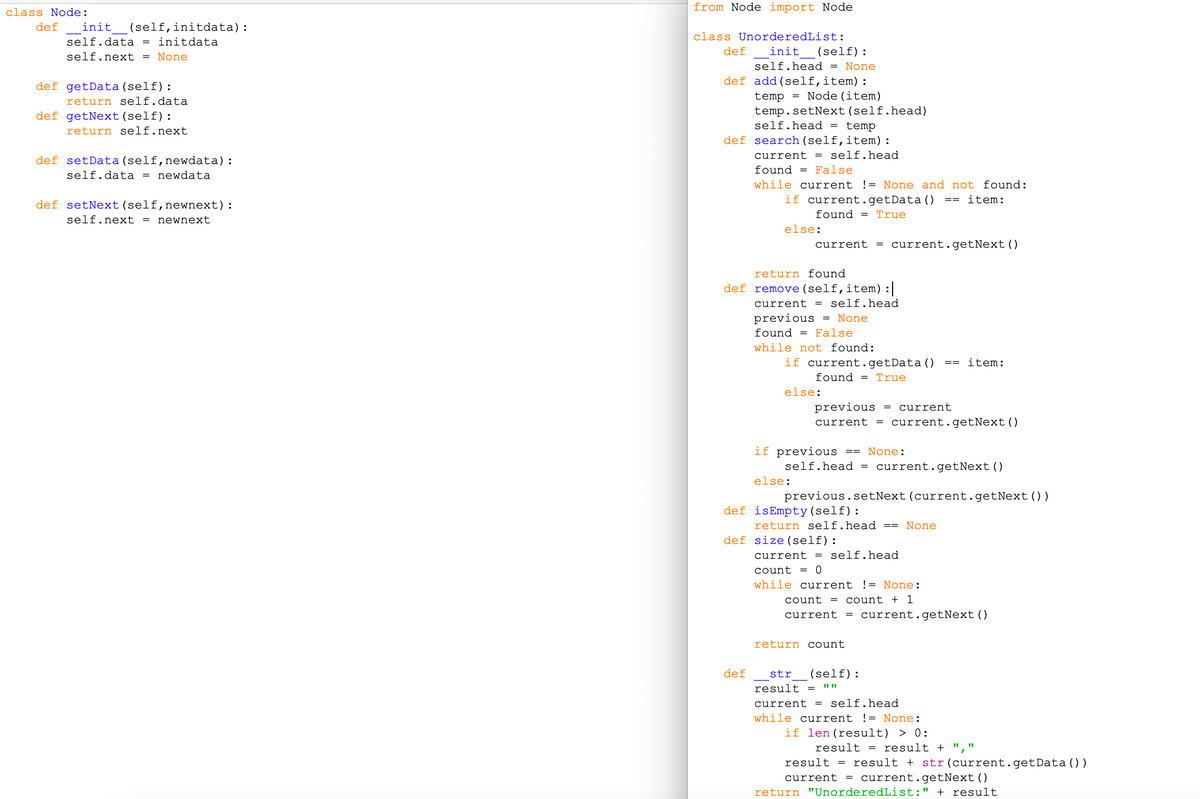
Transcribed Image Text:from Node import Node
class Node:
def
init
(self,initdata):
class UnorderedList:
self.data = initdata
def
init
(self):
self.next = None
self.head = None
def getData(self):
return self.data
def add(self,item):
= Node (item)
temp
temp.setNext(self.head)
self.head = temp
def getNext (self):
return self.next
def search (self,item):
current = self.head
def setData (self,newdata):
found =
False
self.data = newdata
while current != None and not found:
def setNext(self,newnext):
if current.getData ()
item:
found =
True
self.next = newnext
else:
current
= current.getNext()
return found
def remove (self,item) :
current = self.head
previous = None
found = False
while not found:
if current.getData()
item:
found = True
else:
previous
= current
current
= current.getNext()
if previous
self.head = current.getNext()
None:
==
else:
previous.setNext(current.getNext ())
def isEmpty(self):
return self.head == None
def size(self):
current = self.head
count
while current != None:
count =
count + 1
current
= current.getNext ()
return count
def
str
(self):
result =
current = self.head
while current != None:
if len(result) > 0:
result =
result + ",'
result = result + str(current.getData () )
current = current.getNext()
return "UnorderedList:" + result
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
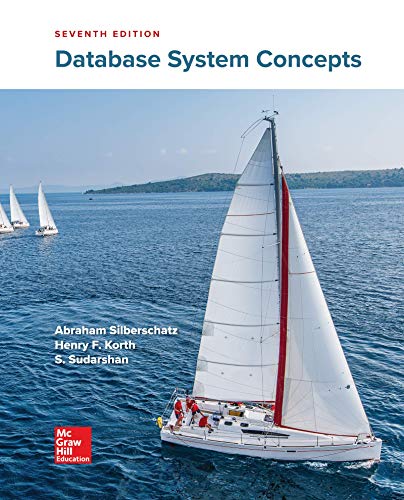
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
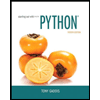
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
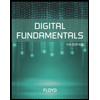
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
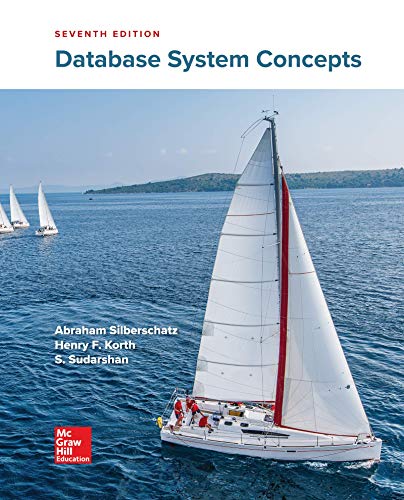
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
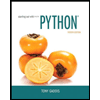
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
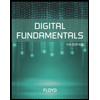
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
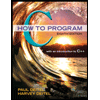
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
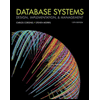
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
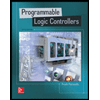
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education