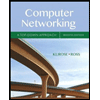
read carefully please
Without using the java collections interface (i.e. do not import java.util.List, LinkedList, etc. )
I need to write a java program that inserts a new String element (String newItem) into a linked list after another specified item (String itemToInsertAfter).
For example if items "A", "B", "C" and "D" are in a linked list in that order and the below method is called, insertAfter("E", "C"), then "E" would be inserted after "C", making the final list to be "A", "B", "C", "E" and "D" with no nulls or blank elements or any elements missing or anything. It should work for all lenghths of linkedlists of Strings.
I am not sure how to do this simple problem, can you help?
public Boolean insertAfter(String newItem, String itemToInsertAfter)
{
// returns true if done successfully, else returns false if itemToInsertAfter cannot be found or some other error
}

Step by stepSolved in 2 steps

- in python pleasearrow_forwardUsing java, write an easier version of a linked list with only a couple of the normal linked list functions and the ability to generate and utilize a list of ints. The data type of the connection to the following node can be just Node, and the data element can be just an int. You will need a reference like (Java) or either a reference to the first node, as well as one to the last node. (Answer the following questions) 1) Create a method or function that accepts an integer, constructs a node with that integer as its data value, and then includes the node to the end of the list. If the new node is the first one, this function will also need to update the reference to the first node. This function will need to update the reference to the final node. Consider how to insert the new node following the previous last node, and keep in mind that the next reference for the list's last node should be null. 2) Create a different method or function that iteratively explores the list, printing…arrow_forwardCreate a DeleteDuplicatesclass which consists of a deleteDuplicates method which takes the nums list as its parameter. Implement the deleteDuplicates method: Check if the input list is null or empty, and return an empty list if so. Initialize a pointer i to keep track of the unique elements. Use a for loop to Iterate through the list using another pointer j which starts at j=1 and goes until j=nums.size(); If the current element at j is not equal to the previous element at i using !nums.get(i).equals(nums.get(j), increment i and set the current element at j to the new position at i using nums.set(i, nums.get(j)). After the for loop ends, return the sublist from the beginning to i + 1, as this will contain all unique elements. Call the deleteDuplicates method with the sample list as argument: Call the deleteDuplicates method and pass the nums list as argument Store the result in a variable result Print the result to the console. Input: [1, 1, 2, 3, 3,…arrow_forward
- A loop that iteratively processes a given list is called a for construct. So long as there are things to process, it will keep running. To what extent is this statement accurate or false?arrow_forwardCreate a program that removes duplicate integer values from a list. For example if a list has these valuesList = [5, 7, 8, 10, 8, 5, 12, 15, 12]. After applying the removal function, you should have List = [5, 7, 8,10, 12, 15]. In your case you will get the numbers of list from the user.arrow_forwardYou are going to implement a program that creates an unsorted list by using a linked list implemented by yourself. NOT allowed to use LinkedList class or any other classes that offers list functions. It is REQUIRED to use an ItemType class and a NodeType struct to solve this homework. The “data.txt” file has three lines of data 100, 110, 120, 130, 140, 150, 160 100, 130, 160 1@0, 2@3, 3@END You need to 1. create an empty unsorted list 2. add the numbers from the first line to list using putItem() function. Then print all the current keys to command line in one line using printAll(). 3. delete the numbers given by the second line in the list by using deleteItem() function. Then print all the current keys to command line in one line using printAll().. 4. putItem () the numbers in the third line of the data file to the corresponding location in the list. For example, 1@0 means adding number 1 at position 0 of the list. Then print all the current keys to command line in one…arrow_forward
- Please answer the question in the screenshot. The language used is Java.arrow_forwardWrite a line (or lines) of code that uses a list that has been previously defined, named word_list, along with a string value entered by the user beforehand, named find, to print out the percent of the occurrence of that word in the list. As an example, if your word_list looked like this: ['the', 'word', 'I', 'am', 'looking', 'for', 'is', 'called', 'my', 'word'] And find was the string 'word' The Example Output would look like this: 20.00% of the list is word Otherwise, if your word_list looked like this: ['another', 'word', 'that', 'is', 'being', 'found', 'is', 'terracotta'] And find was the string 'looking' That Example Output would look like this: 0.00% of the list is lookingarrow_forwardPlease answer the question in the screenshot. The language used here is in Java.arrow_forward
- The for construction is a loops that iteratively processes a given list. Consequently, it works so long even though there are objects to process. What about this claim: true or false?arrow_forwardin Jave create a method work() with the follow instructions: If the work() method is passed a non-null then it must return a new List that contains some or all of the elements in data. The elements of the new List must be aliases for (not copies of) the objects in the List it is passed. If there are no sign attributes (i.e., the array is null or has 0 elements) then the work() method must return an empty List. If any of the conditions represented by the sign attribute holds for a particular element that that element must be included in the result (i.e., the conditions must be combined using a logical OR). The elements in the returned List must be in the same order they appeared in the original List (though not all elements must be in the result). public List<T> work(List<T> g){arrow_forwardHas to be done in Java. Import ArrayList.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
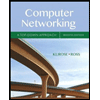
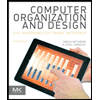
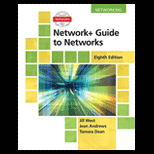
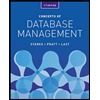
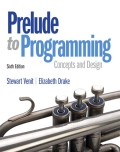
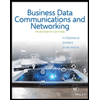