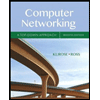
h25.cpp
-----------------------------------------------------------
#include <string>
#include <iostream>
using namespace std;
#include "h25.h"
// Add your code here
PLEASE WRITE THE CODE HERE
//////////////////////// STUDENT TESTING //////////////////////////
int run()
{
cout << "Add your own tests here" << endl;
return 0;
}
h25.h
-----------------------------------------------------------------
#ifndef H25_H_
#define H25_H_
/**
* Reverses the C-style string pointed to by s.
*
* @param s a pointer to the character in a C-style string.
*/
void reverse(char * s);
/**
* Finds the first occurrence of str2 which appears in str1.
* Returns a pointer to the first occurrence of str2 in str1.
* If no match is found, then a null pointer is returned.
* If str2 points to a string of zero length, then the argument str1 is returned.
*
* @param str1 C-string to search through.
* @param str2 C-string to search for.
* @return
*/
const char * findStr(const char *str1, const char *str2);
// DO NOT CHANGE THESE LINES
#define strcmp static_assert(false, "strcmp not allowed");
#define strstr static_assert(false, "strstr not allowed");
#define strlen(s) static_assert(false, "strlen not allowed");
#define strcat(d, s) static_assert(false, "strcat not allowed");
#define strcpy(d, s) static_assert(false, "strcpy not allowed");
#define string static_assert(false, "string not allowed");
#endif
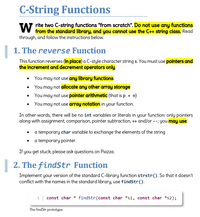
![The function:
• finds the first occurrence of the entire string s2 inside the string s1
• returns a pointer to the first occurrence.
• If no match is found, then return the C++ nullptr.
• If s2 points to a string of zero length, then return s1.
Here are some examples:
findstr("cowtown", "ow"); // returns &s1[1]
findstr("cowtown", "own"); // returns &s1[4]
3 findstr("cowtown", "two"); // returns nullptr
Using the findStr function.
You may use pointer notation or array notation. However, do not use any functions
from the standard library. That includes strlen() or the C++ string class.](https://content.bartleby.com/qna-images/question/79920f54-6dd5-4f69-b3b3-91d2ebf645a4/8f2a7dfa-b47c-4fb2-9726-39d2fe054639/8yc1m4_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

- #include <string>#include <iostream>#include <stdlib.h> #include <stdio.h>#include <iomanip> using namespace std; //################################################################################################# class Dice{ private: int value; public: //Randomly assigns a value between from 1 to 6 to the dice. void roll(){ value = rand() % 6 + 1; } int reveal() {return value;} void setValue(int value) {this->value = value;} }; //################################################################################################# const int HAND_SIZE = 5; class Hand { public: Dice dices[HAND_SIZE]; //Display the value of the five dice void show(){ for(int i = 0; i < HAND_SIZE; i++){ cout << dices[i].reveal() << " "; } cout << endl; } void play(){ for(int i = 0; i < HAND_SIZE; i++){ dices[i].roll(); } } Dice* getDice(int diceNum){…arrow_forward# ===================== Provided Helper Functions ===================== def transform_string(s: str) -> str: """Return a new string based on s in which all letters have been converted to uppercase and punctuation characters have been stripped from both ends. Inner punctuation is left untouched. >>> transform_string('Birthday!!!') 'BIRTHDAY' >>> transform_string('"Quoted?"') 'QUOTED' >>> transform_string('To be? Or not to be?') 'TO BE? OR NOT TO BE' """ punctuation = """!"'`@$%^&_-+={}|\\/,;:.-?)([]<>*#\n\t\r""" result = s.upper().strip(punctuation) return result def is_vowel_phoneme(phoneme: str) -> bool: """Return True if and only if phoneme is a vowel phoneme. That is, whether phoneme ends in a 0, 1, or 2. Precondition: len(phoneme) > 0 and phoneme.isupper() >>> is_vowel_phoneme('AE0') True >>> is_vowel_phoneme('DH') False >>>…arrow_forward# file name: w07_markus.py## Complete the following steps:# (1) Complete the following function according to its docstring.# (2) Save your file after you make changes, and then run the file by# clicking on the green Run button in Wing101. This will let you call your# modified function in the Python shell. An asterisk * on the Wing101# w07_markus.py tab indicates that modifications have NOT been saved.# (3) Test your function in the Wing101 shell by evaluating the examples from# the docstring and confirming that the correct result is displayed.# (4) Test your function using different function arguments.# (5) When you are convinced that your function is correct, submit your# modified file to MarkUs. You can find instructions on submitting a file# to MarkUs in Week *2* Perform -> Accessing Part 2 of the# Week 2 Perform (For Credit) on PCRS.# (6) Verify you have submitted the right file to MarkUs by downloading it# and checking that the…arrow_forward
- C++ OOP You are creating an Employee Record App. Following information is required to store.o name. A string that holds the employee’s name.o idNumber. An int variable that holds the employee’s ID number.o department. A string that holds the name of the department where the employeeworks.o position. A string that holds the employee’s job title.o CovidStatus: Either the employee has been covid Positive or not.arrow_forwardIn Python, you can use ______ to create Strings. single quotes (') double quotes (") three single quotes (''') All of the above A and B only None of the abovearrow_forward56 bool LoginMenu::authenticate() { bool valid = false; 57 58 SONHORANNKRARRETERL 59 60 61 62 63 64 65 66 67 68 69 } 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 } bool LoginMenu::doLogin() { int attempt = 0; do { } for (int i = 0; i > username; cout > password; bool LoginMenu::create() { login.setUsername (username); login.setPassword (password); // TODO Lab 3 - set login DateTime cout << endl; if (authenticate()) { return true; } } while (++attempt < 3); return false; 95 bool LoginMenu::remove() { 96 97 98 99 == cout << "Todo create" << endl; return true; bool LoginMenu::reset() { cout << "Todo Remove" << endl; return true; 100 101 102 103 } 104 105 bool LoginMenu::do Logout() { 106 107 108 109 110 111 cout << "Todo Reset" << endl; return true; cout << "Todo Logout" << endl; int mo = 0, d = 0, yr = 0, hr = 0, min = 0, sec = 0; getCurrent Time (mo, d, yr, hr, min, sec); // TODO set logoutDateTime return true;arrow_forward
- C# Programming, Chapter 12 Question 6. Create a project naned BreezyViewApartments that contains a Form for an apartment rental service. Allow the user to choose a number of bedrooms, number of baths, and view--street or lake. After the user makes selections, display the monthly rent, which is calculated using a base price of $450, $550, or $700 per month for a one-, two-, or three-bedroom apartment, respectively. $75 is added to the base price for more than one bath, and $50 additional is added to the price for a lake view. Use the Controls that you think are best for each function. Label items appropriately, and the fonts and colors to achieve an attractive design. Any help of input, output and comments through visual studio 2022 and Windows form would be helpful.arrow_forward#include <xc.h> void ____(void); void main (void) { TRISDbits.TRISD0 = ____;TRISDbits.TRISD1 = 0;TRISDbits.TRISD2 = ____;TRISDbits.TRISD3 = 0; ____ = 0; PORTDbits.RD0 = ____; PORTDbits.RD1 = 0; if(____ == 0)fail(); PORTDbits.RD0 = ____; PORTDbits.RD1 = 1; if(PORTDbits.RD2 == 0)fail(); ____ = 1; PORTDbits.RD1 = 0; if(____ == 0)____; PORTDbits.RD0 = ____; PORTDbits.RD1 = ____; if(PORTDbits.RD2 == ____)fail(); while(1) { PORTDbits.RD3 = 1; PORTDbits.RD4 = 0; } } void fail(void) { while(1) { PORTDbits.RD3 = ____; PORTDbits.RD4 = ____; } }arrow_forwardDesign the pseudo code that calculates and displays a person's body mass index(bmi) based upon their weight and height. The calculation uses the formula: bmi = (weight/ (height*height)) psedocode must have proper internal documentation, using block and or line comments. Declare all variables before using them. Pseudo code must illustrate calculations.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
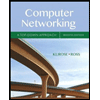
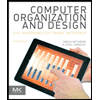
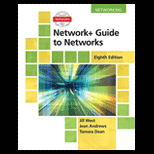
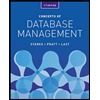
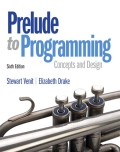
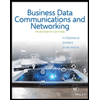