Objective To learn to code, compile and run a program containing selection structures Assignment Plan and code a C++ program utilizing selection structure. Write an interactive C++ program to determine the day of the week for any given date from 1900-2099. Following are the steps you should use: A. Input the date in 3 separate parts, month, day and 4-digit year. Error check to make sure the month is between 1 and 12, the day between 1 and 31, and the year between 1900-2099. If there is an error, identify the type of error and stop the program. B. If the data is good, divide the last two digits of the year by 4. Store the quotient (ignoring the remainder) in Total. For example, for 1983, divide 83 by 4 and store 20 in Total. C. Add the last 2 digits of the year to Total. D. Add the two digits of the day of the month to Total. E. Using the following table, find the "value of the month" and add it to Total. January = 1 April = 0 July = 0 October = 1 February = 4 May = 2 August = 3 November = 4 March = 4 June = 5 September = 6 December = 6 F. If the year is 2000 or later, add 6. If the year is 2000 exactly and the month is January or February, subtract 1. G. Then, determine if the year is a leap year. A leap year is divisible by 4 but not divisible by 100. If it is a leap year and the month is January or February, subtract 1. H. Find the remainder when the Total is divided by 7. I. Use the remainder to find the day: 0 = Saturday 3 = Tuesday 6 = Friday 2 = Monday | 1= Sunday 4 = Wednesday 5 = Thursday Input Your three initials, the month, day, and 4-digit year of the date. Output All user prompts. Your 3 initials. All the input fields. The day of the week of the date entered if the data is valid. Appropriate error message if the data is invalid. Be sure your output file contains user prompts and what was entered by the user in addition to the results of your program processing. Run Run with the following data: 3/13/2014 - Thursday 1/1/1996 – Monday 2/2/2000 – Wednesday 12/20/1981 - Sunday 3/17/1895 - X 4/10/2130 – X 14/8/1933 – X 10/33/1920 - X Turn In Turn in source code and program outout.
Objective To learn to code, compile and run a program containing selection structures Assignment Plan and code a C++ program utilizing selection structure. Write an interactive C++ program to determine the day of the week for any given date from 1900-2099. Following are the steps you should use: A. Input the date in 3 separate parts, month, day and 4-digit year. Error check to make sure the month is between 1 and 12, the day between 1 and 31, and the year between 1900-2099. If there is an error, identify the type of error and stop the program. B. If the data is good, divide the last two digits of the year by 4. Store the quotient (ignoring the remainder) in Total. For example, for 1983, divide 83 by 4 and store 20 in Total. C. Add the last 2 digits of the year to Total. D. Add the two digits of the day of the month to Total. E. Using the following table, find the "value of the month" and add it to Total. January = 1 April = 0 July = 0 October = 1 February = 4 May = 2 August = 3 November = 4 March = 4 June = 5 September = 6 December = 6 F. If the year is 2000 or later, add 6. If the year is 2000 exactly and the month is January or February, subtract 1. G. Then, determine if the year is a leap year. A leap year is divisible by 4 but not divisible by 100. If it is a leap year and the month is January or February, subtract 1. H. Find the remainder when the Total is divided by 7. I. Use the remainder to find the day: 0 = Saturday 3 = Tuesday 6 = Friday 2 = Monday | 1= Sunday 4 = Wednesday 5 = Thursday Input Your three initials, the month, day, and 4-digit year of the date. Output All user prompts. Your 3 initials. All the input fields. The day of the week of the date entered if the data is valid. Appropriate error message if the data is invalid. Be sure your output file contains user prompts and what was entered by the user in addition to the results of your program processing. Run Run with the following data: 3/13/2014 - Thursday 1/1/1996 – Monday 2/2/2000 – Wednesday 12/20/1981 - Sunday 3/17/1895 - X 4/10/2130 – X 14/8/1933 – X 10/33/1920 - X Turn In Turn in source code and program outout.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter3: Assignment, Formatting, And Interactive Input
Section3.2: Formatting Numbers For Program Output
Problem 10E
Related questions
Question
Please don't use array or function in your coding. Just use (if-else) and (switch case-break).
Thanks
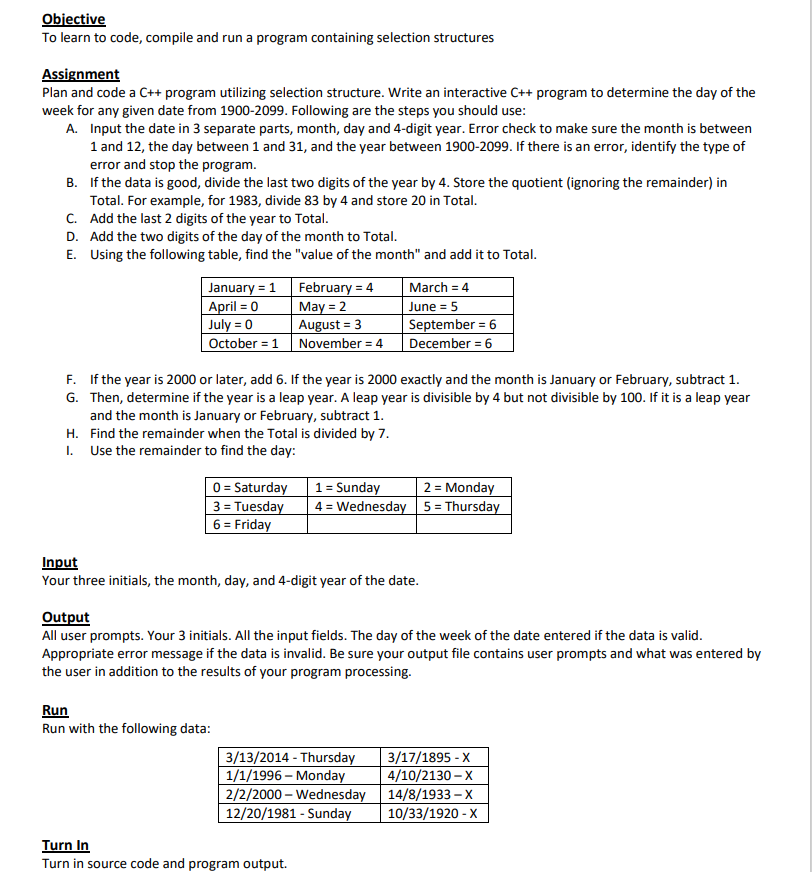
Transcribed Image Text:Objective
To learn to code, compile and run a program containing selection structures
Assignment
Plan and code a C++ program utilizing selection structure. Write an interactive C++ program to determine the day of the
week for any given date from 1900-2099. Following are the steps you should use:
A. Input the date in 3 separate parts, month, day and 4-digit year. Error check to make sure the month is between
1 and 12, the day between 1 and 31, and the year between 1900-2099. If there is an error, identify the type of
error and stop the program.
B. If the data is good, divide the last two digits of the year by 4. Store the quotient (ignoring the remainder) in
Total. For example, for 1983, divide 83 by 4 and store 20 in Total.
C. Add the last 2 digits of the year to Total.
D. Add the two digits of the day of the month to Total.
E. Using the following table, find the "value of the month" and add it to Total.
January = 1
April = 0
July = 0
October = 1 November = 4
February = 4
May = 2
August = 3
March = 4
June = 5
September = 6
December = 6
F. If the year is 2000 or later, add 6. If the year is 2000 exactly and the month is January or February, subtract 1.
G. Then, determine if the year is a leap year. A leap year is divisible by 4 but not divisible by 100. If it is a leap year
and the month is January or February, subtract 1.
H. Find the remainder when the Total is divided by 7.
I. Use the remainder to find the day:
0= Saturday
3 = Tuesday
1 = Sunday
4 = Wednesday 5 = Thursday
2 = Monday
%3D
6 = Friday
Input
Your three initials, the month, day, and 4-digit year of the date.
Output
All user prompts. Your 3 initials. All the input fields. The day of the week of the date entered if the data is valid.
Appropriate error message if the data is invalid. Be sure your output file contains user prompts and what was entered by
the user in addition to the results of your program processing.
Run
Run with the following data:
3/13/2014 - Thursday
1/1/1996 – Monday
2/2/2000 – Wednesday
12/20/1981 - Sunday
3/17/1895 - X
4/10/2130 – X
14/8/1933 – X
10/33/1920 - X
Turn In
Turn in source code and program output.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 10 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
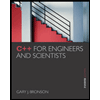
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
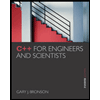
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr