Only in c++ please. Just need the solution code. Thank you. SavingsAccount is a class with two double* data members pointing to the amount saved and interest rate of the savings account, respectively. Two doubles are read from input to initialize mySavingsAccount. Write a copy constructor for SavingsAccount that creates a deep copy of mySavingsAccount. At the end of the copy constructor, output "Produced a new SavingsAccount object" and end with a newline. Ex: If the input is 78.00 1.00, then the output is: Produced a new SavingsAccount object Initial amount saved: $78.00 with 2.00 interest rate Produced a new SavingsAccount object After 1 month(s): $234.00 After 2 month(s): $702.00 After 3 month(s): $2106.00 After 4 month(s): $6318.00 After 5 month(s): $18954.00 Custom value interest rate $78.00 with 1.00 interest rate#include #include using namespace std; class SavingsAccount { public: SavingsAccount(double startingAmount = 0.0, double startingRate = 0.0); SavingsAccount(const SavingsAccount& acc); void SetAmount(double newAmount); void SetRate(double newRate); double GetAmount() const; double GetRate() const; void Print() const; private: double* amount; double* rate; }; SavingsAccount::SavingsAccount(double startingAmount, double startingRate) { amount = new double(startingAmount); rate = new double(startingRate); } /* Your code goes here */ void SavingsAccount::SetAmount(double newAmount) { *amount = newAmount; } void SavingsAccount::SetRate(double newRate) { *rate = newRate; } double SavingsAccount::GetAmount() const { return *amount; } double SavingsAccount::GetRate() const { return *rate; } void SavingsAccount::Print() const { cout << fixed << setprecision(2) << "$" << *amount << " with " << *rate << " interest rate" << endl; } void SimulateGrowth(SavingsAccount c, int months) { for (auto i = 1; i <= months; ++i) { c.SetAmount(c.GetAmount() * (c.GetRate() + 1.0)); cout << "After " << i << " month(s): " << "$" << c.GetAmount() << endl; } } int main() { double amount; double rate; cin >> amount; cin >> rate; SavingsAccount mySavingsAccount(amount, rate); SavingsAccount mySavingsAccountCopy = mySavingsAccount; mySavingsAccount.SetRate(rate + 1.0); cout << "Initial amount saved: "; mySavingsAccount.Print(); SimulateGrowth(mySavingsAccount, 5); cout << endl; cout << "Custom value interest rate" << endl; mySavingsAccountCopy.Print(); return 0; }
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Only in c++ please. Just need the solution code. Thank you.
SavingsAccount is a class with two double* data members pointing to the amount saved and interest rate of the savings account, respectively. Two doubles are read from input to initialize mySavingsAccount. Write a copy constructor for SavingsAccount that creates a deep copy of mySavingsAccount. At the end of the copy constructor, output "Produced a new SavingsAccount object" and end with a newline.
Ex: If the input is 78.00 1.00, then the output is:
Produced a new SavingsAccount object Initial amount saved: $78.00 with 2.00 interest rate Produced a new SavingsAccount object After 1 month(s): $234.00 After 2 month(s): $702.00 After 3 month(s): $2106.00 After 4 month(s): $6318.00 After 5 month(s): $18954.00 Custom value interest rate $78.00 with 1.00 interest rate#include <iostream> #include <iomanip> using namespace std; class SavingsAccount { public: SavingsAccount(double startingAmount = 0.0, double startingRate = 0.0); SavingsAccount(const SavingsAccount& acc); void SetAmount(double newAmount); void SetRate(double newRate); double GetAmount() const; double GetRate() const; void Print() const; private: double* amount; double* rate; }; SavingsAccount::SavingsAccount(double startingAmount, double startingRate) { amount = new double(startingAmount); rate = new double(startingRate); } /* Your code goes here */ void SavingsAccount::SetAmount(double newAmount) { *amount = newAmount; } void SavingsAccount::SetRate(double newRate) { *rate = newRate; } double SavingsAccount::GetAmount() const { return *amount; } double SavingsAccount::GetRate() const { return *rate; } void SavingsAccount::Print() const { cout << fixed << setprecision(2) << "$" << *amount << " with " << *rate << " interest rate" << endl; } void SimulateGrowth(SavingsAccount c, int months) { for (auto i = 1; i <= months; ++i) { c.SetAmount(c.GetAmount() * (c.GetRate() + 1.0)); cout << "After " << i << " month(s): " << "$" << c.GetAmount() << endl; } } int main() { double amount; double rate; cin >> amount; cin >> rate; SavingsAccount mySavingsAccount(amount, rate); SavingsAccount mySavingsAccountCopy = mySavingsAccount; mySavingsAccount.SetRate(rate + 1.0); cout << "Initial amount saved: "; mySavingsAccount.Print(); SimulateGrowth(mySavingsAccount, 5); cout << endl; cout << "Custom value interest rate" << endl; mySavingsAccountCopy.Print(); return 0; }

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

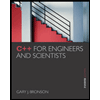
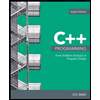
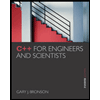
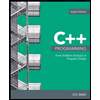