Paper Scissors Rock “Strategy” Purpose:To review class inheritance. Directions and Examples: Download the starter code from the dropbox where you got this assignment. This code contains a class called Player that randomly chooses paper, scissors or rock with equal probability. It also contains a class called PaperScissorsRock that creates two Player objects, has them play one million games, and counts the number of times Player 1 wins. Your task for the practice lab is to create a subclass of Player called StrategicPlayer. The StrategicPlayer class should have the following fields and methods: private double percentPaper – the likelihood (between 0 and 1) that the player will choose paper private double percentScissors – the likelihood (between 0 and 1) that the player will choose scissors private double percentRock – the likelihood (between 0 and 1) that the player will choose rock public StrategicPlayer(double paper, double scissors, double rock) – a constructor that initializes the object’s fields The class should also override the go method from the Player superclass to choose paper, scissors or rock in the desired percentages. Modify the driver program so that Player 1 is a StrategicPlayer instead of a regular Player and re-run the program. Hint: the outcome should be little changed from the original version no matter what percentages you use, because there is no strategy in Paper Scissors Rock
Paper Scissors Rock “Strategy”
Purpose:To review class inheritance.
Directions and Examples:
Download the starter code from the dropbox where you got this assignment. This code contains a class
called Player that randomly chooses paper, scissors or rock with equal probability. It also contains a class
called PaperScissorsRock that creates two Player objects, has them play one million games, and counts
the number of times Player 1 wins.
Your task for the practice lab is to create a subclass of Player called StrategicPlayer. The StrategicPlayer
class should have the following fields and methods:
private double percentPaper – the likelihood (between 0 and 1) that the player will choose paper
private double percentScissors – the likelihood (between 0 and 1) that the player will choose scissors
private double percentRock – the likelihood (between 0 and 1) that the player will choose rock
public StrategicPlayer(double paper, double scissors, double rock) – a constructor that initializes the
object’s fields
The class should also override the go method from the Player superclass to choose paper, scissors or
rock in the desired percentages.
Modify the driver program so that Player 1 is a StrategicPlayer instead of a regular Player and re-run the
program. Hint: the outcome should be little changed from the original version no matter what
percentages you use, because there is no strategy in Paper Scissors Rock
Given Code:

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

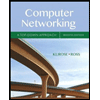
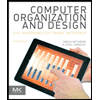
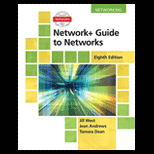
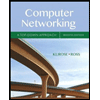
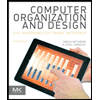
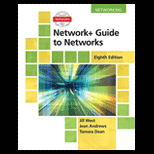
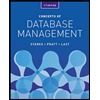
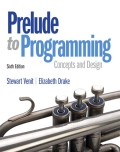
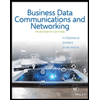