Part II: Understand Pointers/Dynamic Memory To-do 2: Write 3 different functions in C++ to create memory on the heap without causing a memory leak. Hint: You will need to assign the address to a pointer that was created outside the function. Remember, you can return an address or change what a pointer points to (the contents of a pointer) in a function by passing the pointer by reference or by passing the address of the pointer. Additional questions: What if you want to change the contents of what the pointer points to? Make a function that will set the contents of the space on the heap. Do you still need to pass by reference or the address of the pointer? Why or why not? How will you delete the memory off the heap? Try doing it outside a function, and inside a function. Make sure your delete function is setting your pointer back to NULL, since it is not supposed to be pointing anywhere anymore. You can check to see if you have any memory leaks using valgrind. svalgrind program_exe Part IlII: Implementing 1-D arrays in assignment 4 To-do 3: Create a C-style string that will hold a sentence/paragraph inputted by the user. • You can make a static array for this, but make sure your getline has the appropriate length. • Use cin.getline (array_name, num_chars_to_read) for C-style strings. • What do you think may happen if you enter more characters than specified by num_chars_to_read in cin.getline ()? The code below may help this problem. if (cin.fail(0) { cin.ignore (256,'\n'); //get rid of everything leftover cin.clear (); //reset the failbit for the next cin To-do 4: Create an array of C++ strings for any N number of words that user wants to search for and read those words from the user. • Ask the user how many words they want to search for. • Create an array of that many words • Read each word from the user. Remember, you would use getline for C++ strings. getline (cin, word array_name [i]); You need to have a main function that asks for a sentence/paragraph and for the N words. After getting inputs from the user, print out both arrays to check if they are working properly. Remember to check memory leaks as well.
Part II: Understand Pointers/Dynamic Memory To-do 2: Write 3 different functions in C++ to create memory on the heap without causing a memory leak. Hint: You will need to assign the address to a pointer that was created outside the function. Remember, you can return an address or change what a pointer points to (the contents of a pointer) in a function by passing the pointer by reference or by passing the address of the pointer. Additional questions: What if you want to change the contents of what the pointer points to? Make a function that will set the contents of the space on the heap. Do you still need to pass by reference or the address of the pointer? Why or why not? How will you delete the memory off the heap? Try doing it outside a function, and inside a function. Make sure your delete function is setting your pointer back to NULL, since it is not supposed to be pointing anywhere anymore. You can check to see if you have any memory leaks using valgrind. svalgrind program_exe Part IlII: Implementing 1-D arrays in assignment 4 To-do 3: Create a C-style string that will hold a sentence/paragraph inputted by the user. • You can make a static array for this, but make sure your getline has the appropriate length. • Use cin.getline (array_name, num_chars_to_read) for C-style strings. • What do you think may happen if you enter more characters than specified by num_chars_to_read in cin.getline ()? The code below may help this problem. if (cin.fail(0) { cin.ignore (256,'\n'); //get rid of everything leftover cin.clear (); //reset the failbit for the next cin To-do 4: Create an array of C++ strings for any N number of words that user wants to search for and read those words from the user. • Ask the user how many words they want to search for. • Create an array of that many words • Read each word from the user. Remember, you would use getline for C++ strings. getline (cin, word array_name [i]); You need to have a main function that asks for a sentence/paragraph and for the N words. After getting inputs from the user, print out both arrays to check if they are working properly. Remember to check memory leaks as well.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter12: Points, Classes, Virtual Functions And Abstract Classes
Section: Chapter Questions
Problem 1TF
Related questions
Question
![Part II: Understand Pointers/Dynamic Memory
To-do 2:
Write 3 different functions in C++ to create memory on the heap without causing a memory
leak.
Hint: You will need to assign the address to a pointer that was created outside the function. Remember,
you can return an address or change what a pointer points to (the contents of a pointer) in a function by
passing the pointer by reference or by passing the address of the pointer.
Additional questions:
What if you want to change the contents of what the pointer points to? Make a function that will set
the contents of the space on the heap. Do you still need to pass by reference or the address of the
pointer? Why or why not?
How will you delete the memory off the heap? Try doing it outside a function, and inside a function.
Make sure your delete function is setting your pointer back to NULL, since it is not supposed to be
pointing anywhere anymore.
You can check to see if you have any memory leaks using valgrind.
Svalgrind program_exe
Part IlI: Implementing 1-D arrays in assignment 4
To-do 3:
Create a C-style string that will hold a sentence/paragraph inputted by the user.
You can make a static array for this, but make sure your getline has the appropriate length.
Use cin.getline (array_name, num_chars_to_read) for C-style strings.
What do you think may happen if you enter more characters than specified by
num_chars_to_readin cin.getline ()? The code below may help this problem.
if (cin.fai1()) {
cin.ignore (256,'\n'); //get rid of everything leftover
cin.clear ();
//reset the failbit for the next cin
}
To-do 4:
Create an array of C++ strings for any N number of words that user wants to search for
and read those words from the user.
Ask the user how many words they want to search for.
Create an array of that many words
Read each word from the user. Remember, you would use getline for C++ strings.
getline (cin, word_array_name[i]);
You need to have a main function that asks for a sentence/paragraph and for the N words. After getting
inputs from the user, print out both arrays to check if they are working properly. Remember to check
memory leaks as well.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8759b09c-a99c-4616-917a-1eb84cf5071d%2F3bbe75a1-5da9-4bf0-95b0-a318cb060cc4%2Fyado48_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Part II: Understand Pointers/Dynamic Memory
To-do 2:
Write 3 different functions in C++ to create memory on the heap without causing a memory
leak.
Hint: You will need to assign the address to a pointer that was created outside the function. Remember,
you can return an address or change what a pointer points to (the contents of a pointer) in a function by
passing the pointer by reference or by passing the address of the pointer.
Additional questions:
What if you want to change the contents of what the pointer points to? Make a function that will set
the contents of the space on the heap. Do you still need to pass by reference or the address of the
pointer? Why or why not?
How will you delete the memory off the heap? Try doing it outside a function, and inside a function.
Make sure your delete function is setting your pointer back to NULL, since it is not supposed to be
pointing anywhere anymore.
You can check to see if you have any memory leaks using valgrind.
Svalgrind program_exe
Part IlI: Implementing 1-D arrays in assignment 4
To-do 3:
Create a C-style string that will hold a sentence/paragraph inputted by the user.
You can make a static array for this, but make sure your getline has the appropriate length.
Use cin.getline (array_name, num_chars_to_read) for C-style strings.
What do you think may happen if you enter more characters than specified by
num_chars_to_readin cin.getline ()? The code below may help this problem.
if (cin.fai1()) {
cin.ignore (256,'\n'); //get rid of everything leftover
cin.clear ();
//reset the failbit for the next cin
}
To-do 4:
Create an array of C++ strings for any N number of words that user wants to search for
and read those words from the user.
Ask the user how many words they want to search for.
Create an array of that many words
Read each word from the user. Remember, you would use getline for C++ strings.
getline (cin, word_array_name[i]);
You need to have a main function that asks for a sentence/paragraph and for the N words. After getting
inputs from the user, print out both arrays to check if they are working properly. Remember to check
memory leaks as well.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
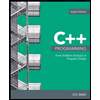
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
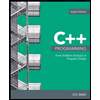
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning