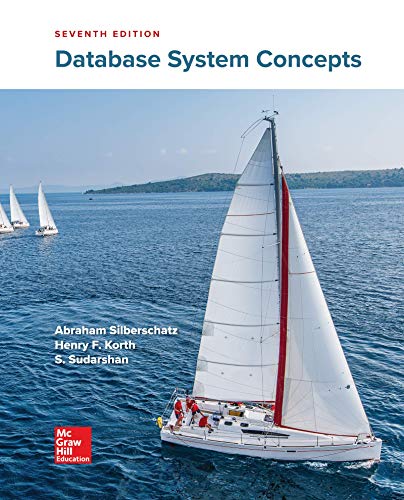
please answer this question using python 3.
Submit function:
reads the five inputs, calls the functions listed below as needed, and displays tax.
Inputs
# of jobs:
income :
# of children:
Is Married?:
Is Student? :
Outputs: tax
functions Required
1. count_regular_exemptions()
Calculates number of regular exemptions.
- Everyone starts with one exemption
- If no jobs, you get one more exemption
- If you have one job:
You get one more exemption if you are married, or if you are a single student.
- If you have more than one job:
You get one more exemption if your income is less than 1500.
2. count_child_exemptions()
Calculates number of child credit exemptions.
- If your income is less than $70,000 : You get 4 times the number of children as the number of exemptions. Example: income 60,000 with 3 children: 4 * 3 = 12 exemptions
- If your income is at or above $70,000 : You get one additional exemption for every 2 kids. That is: 0 exemptions for 1 child, 1 for 2 children, 1 for 3, 2 for 4 and 5 children, 3 for 6 and 7 children, etc.
3. compute_taxable_income()
Calculates the taxable income.
Each child exemption is worth $1000.
Each regular exemption is worth $2000.
exemption amount is the sum of child exemption amount and regular exemption amount, each computed using the counts and unit exemptions above.
Taxable income = Income - Exemption amount
4. compute_tax_rate
Determine tax rate based on taxable income.
Find the tax rate based on the table below.
Rate |
Single |
Married |
10% |
Up to,including, 38,000 |
Up to and including $78,000 |
15% |
Above 38,000 |
Above 78,000 |
tax is determined using taxable income and tax rate.
5. compute_deduction
Calculate deduction amount to be subtracted from tax.
Deduction amount starts at 0 dollars.
- if student and income < $80,000: $2000
- if student and income < $57,000: $3000
The income referred to above is the ‘income’ input by the user. Not taxable income.
tax is updated by subtracting any deduction amount from tax.
If tax < 0: set tax due to $0.00.
Output format: Your estimated taxes due are: $XXXX
Summary:
Show average tax for students, and the number of taxpayers. Use a function to compute the average.
Reset: Getting ready for a new series of inputs.
Additional features:
- revisit and optimize the if statements to be as efficient as possible
- Include counts of: married students, single students, and non-student taxpayers in summary
#main
quit = False
while not quit:
print('1.Submit 2.Summary 3.Reset 4.Exit')
choice = int(input('Enter choice: '))
if choice == 1:
submit()
elif choice == 2:
summary()
elif choice == 3:
reset()
elif choice == 4:
quit = True
else:
print('Invalid Choice!')

- Initialize the following variables:
- num_married_students = 0
- num_single_students = 0
- num_non_student_taxpayers = 0
- total_tax_students = 0
- total_tax_taxpayers = 0
- Define a function count_regular_exemptions that takes num_jobs, income, is_married, and is_student as input. a. Set exemptions to 1. b. If num_jobs is 0, increment exemptions by 1. c. If num_jobs is 1:
- If is_married or is_student is true, increment exemptions by 1.
- If income is less than 1500, increment exemptions by 1. d. If num_jobs is greater than 1 and income is less than 1500, increment exemptions by 1. e. Return the calculated exemptions.
- Define a function count_child_exemptions that takes income and num_children as input. a. If income is less than 70000, return 4 times the number of children. b. Otherwise, return the integer division of num_children by 2.
- Define a function compute_taxable_income that takes income and exemptions as input. a. Calculate the child_exemption_amount as 1000 times the child exemptions. b. Calculate the regular_exemption_amount as 2000 times the regular exemptions. c. Calculate the total_exemption_amount as the sum of child_exemption_amount and regular_exemption_amount. d. Return the difference between income and total_exemption_amount as the taxable income.
- Define a function compute_tax_rate that takes taxable_income and is_married as input. a. If is_married is true and taxable_income is less than or equal to 78000, return 0.10. b. If is_married is true and taxable_income is greater than 78000, return 0.15. c. If is_married is false and taxable_income is less than or equal to 38000, return 0.10. d. If is_married is false and taxable_income is greater than 38000, return 0.15.
- Define a function compute_deduction that takes is_student and income as input. a. Set deduction_amount to 0. b. If is_student is true and income is less than 80000, set deduction_amount to 2000. c. If is_student is true, income is less than 57000, and deduction_amount is already set to 2000, update deduction_amount to 3000. d. Return the deduction_amount.
- Define a function submit that takes no input. a. Within submit: i. Prompt the user for input: num_jobs, income, num_children, is_married, and is_student. ii. Calculate exemptions using count_regular_exemptions and count_child_exemptions functions. iii. Calculate taxable_income using compute_taxable_income function. iv. Calculate tax_rate using compute_tax_rate function. v. Calculate tax as taxable_income multiplied by tax_rate. vi. Calculate deduction using compute_deduction function. vii. Subtract deduction from tax and ensure it is non-negative. viii. Display the estimated taxes due. ix. Update counts and total tax for the summary.
- Define a function summary that takes no input. a. Within summary: i. Calculate the average tax for students and display it. ii. Display the number of taxpayers - married students, single students, and non-student taxpayers, and the total count.
- Define a function reset that takes no input. a. Within reset: i. Reset all count variables to 0.
Implement the main program using a while loop: a. Display a menu with options for submission, summary, reset, and exit. b. Prompt the user for a choice. c. Based on the user's choice, call the appropriate function: submit, summary, reset, or exit the program. d. Handle invalid choices by displaying an error message.
End the algorithm.
Step by stepSolved in 3 steps with 1 images

- Payhon programing. Please see attachment. Thanksarrow_forwardIn python write a program that: Function argumentsFor this exercise, you are asked to code three (3) functions that do nothing (execute the pass statement), but accept different combinations of positional arguments, named arguments (mandatory or not) and star or double star arguments, with potentially default values. Your duties must be called respectively: function1,function2,and function3.For function1, it must accept three (3) positional arguments of which two (2) are mandatory and one (1) has a zero (integer) default value. You are free to name these arguments as you see fit. For function2, it must accept one (1) mandatory positional argument and two (2) mandatory arguments named kw1 and kw2 with default values of None and '', respectively. For function3, it must accept an arbitrary number of positional values or named values.arrow_forward3. Consider a function that will test whether a person is qualified to finance a luxury car. They are qualified if their annual income is greater than $100,000 and the sales price is less than $1,000,000. Income and sales price are parameters of the function. The function returns a message to the program stating whether the person is qualified or not. Complete this function table Purpose a. Name Parameters Result finance() b. Write the function: c. Write a Python program that inputs income and sales price from the user. Using a while loop, the program validates the income is greater than zero. The program invokes the finance() function with this print statement: "print (finance (income, salesPrice))" d. Test your while loop by seeing what happens when an invalid income is input and then a valid income. Then run your program once with too low an income to finance but okay sales price, once with an okay income and okay sales price, and once with an okay income but too high a sales price.arrow_forward
- Define the following functions: find_base_area() has two parameters as a prism's base length and base width. The function returns the area of the prism's base. The area of the base is calculated by: find_vol() has three parameters as a prism's base length, base width, and height. The function returns the prism's volume, and uses the find_base_area() function to calculate the prism's base area. The volume is calculated by: Ex: If the input is: 4.0 2.0 3.0 then the output is: Prism base length: 4.0 Prism base width: 2.0 Prism height: 3.0 Base area: 8.0 Volume: 24.0arrow_forward. The electric company charges according to the following rate schedule: 9 cents per kilowatt-hour (kwh) for the first 300 kwh 8 cents per kwh for the next 300 kwh (up to 600 kwh) 6 cents per kwh for the next 400 kwh (up to 1,000 kwh) 5 cents per kwh for all electricity used over 1,000 kwh Write a function to compute the total charge for each customer. Write a main function to call the charge calculation function using the following data: Customer Number Kilowatt-hours Used 123 725 205 115 464 600 596 327 … … The program should print a three column chart listing the customer number, the kilowatt hours used, and the charge for each customer. The program should also compute and print the number of customers, the total kilowatt hours used, and the total charges.arrow_forwardWhich statement of the following is the most appropriate? Group of answer choices One good method for specifying what a function is supposed to do is to provide a precondition and postcondition for the function. One good method for specifying what a function is supposed to do is to provide a precondition and postcondition for the function. These form a contract between the programmer who uses the function and the programmer who writes the function. Using the assert function to check preconditions can significantly reduce debugging time, and the assertion-checking can later be turned off if program speed is a consideration. One good method for specifying what a function is supposed to do is to provide a precondition and postcondition for the function. These form a contract between the programmer who uses the function and the programmer who writes the function. Using the assert function to check preconditions can significantly reduce debugging time, and the…arrow_forward
- Problem 2: Simple Interest The formula for computing the future value of an amount that is increasing due to accumulating simple interest is F=P(1+rxi) Where F is the future value, P is the present value, is the annual interest rate (decimal value not percent), and is the total time period in years. Write a function named simpleInterest that accepts values for the three variables P, and and assings them to the output variable futureValue Function Code to call your function > Save C Reset function [futureValue] =simpleInterest (presentValue, interest RatePercent, time Years) %Enter commands to perform the computation and assign the results 3 %to the output variable defined in the function command in line 1. 1 [futureValue] =simpleInterest (10000,5.25,5) My Solutions > MATLAB Documentation C Reset Run Functionarrow_forward2. Safir Market wants you to create a simple python program for calculating Total cost and change for their customers. The program should start by reading price, articles and Money. Then you need to do the following: • Create a void function that will display "Safir Market" • Create a function with return value and with parameters that will compute Total cost as product of articles and price • Create a function that will accept the computed Total cost as a parameter and will compute the change. If the Total cost is more than or equal to Money, the balance is to be calculated as Money - Total cost, otherwise print the message 'Money not enough' • Display the output as shown below, Sample output Enter the price: 345 Enter number of articles: 4 Enter Money: 65 -Safir Market Total cost is: 1380.0 Money not enough Change is: Nonearrow_forwardOne foot equals 12 inches. Write a function, using python, named feet_to_inches that accepts a number of feet as an argument and returns the number of inches in that many feet. Use the function in a programs, using python, that prompts the user to enter a number of feet then displays the number of inches in that many feet.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
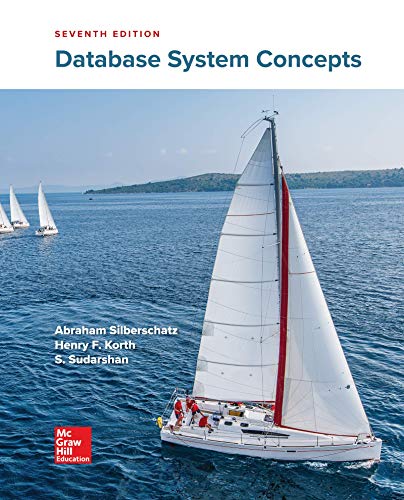
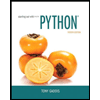
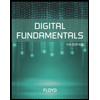
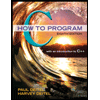
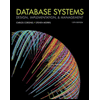
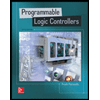