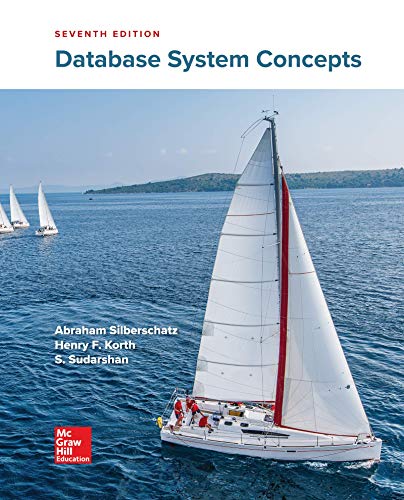
Please code in python
Declare a function named contains_char. Its purpose is when given two strings, the first of any length, the second a single character, it will return True if the single character of the second string is found at any index of the first string, and return False otherwise. More specifically, declare your contains_char function such that:
- It has two parameters (name them descriptively)
- A string that is being searched through for the second parameter
- A string that is expected to be a single character in length and is the character being searched for
- A boolean return type
- A docstring describing the purpose of the function in your own words
Since the caller of this function can be expected to provide the correct arguments, specifically a second argument whose length is one, we will “assert” this assumption in our code such that an error is raised if it is not found to be true. As the first line of code in your function’s body, add the following assert statement and fill in the blank (_____) with your second parameter’s name:
assert len(_______) == 1

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 3 images

- Problem DNA: Subsequence markingA common task in dealing with DNA sequences is searching for particular substrings within longer DNA sequences. Write a function mark_dna that takes as parameters a DNA sequence to search, and a shorter target sequence to find within the longer sequence. Have this function return a new altered sequence that is the original sequence with all non-overlapping occurrences of the target surrounded with the characters >> and <<. Hints: ● String slicing is useful for looking at multiple characters at once. ● Remember that you cannot modify the original string directly, you’ll need to build a copy. Start with an empty string and concatenate onto it as you loop. Constraints: ● Don't use the built-in replace string method. All other string methods are permitted. >>> mark_dna('atgcgctagcatg', 'gcg') 'at>>gcg<<ctagcatg' >>> mark_dna('atgcgctagcatg', 'gc')…arrow_forwardScrabble Scrabble is a game where players get points by spelling words. Words are scored by adding together the point values of each individual letter. Define a function scrabble_score (word:str) -> int that takes a string word as input and returns the equivalent scrabble score for that word. score = {"a": 1, "c": 3, "f": 4, "i": 1, "1": 1, "o": 1, "r": 1, "u": 1, "t": 1, "x": 8, "z": 10} For example, "b": 3, "h": 4, "n": 1, Your Answer: == 20 assert scrabble_score("quick") assert scrabble_score("code") == 7 1 # Put your answer here 2 Submit "e": 1, "d": 2, "g": 2, "k": 5, "j": 8, "m": 3, "q": 10, "p": 3, "s": 1, "w": 4, "v": 4, "y": 4,arrow_forwardUsing C++ Language In this program you will write a function of void return type named compare that accepts an integer parameter named guess. This function will compare the value of guess with a seeded randomly generated integer between 1 to 100, inclusive, and let the user know what the random number was as well as whether the guess was larger than, smaller than or equal to the random number. NOTE THAT: You will NOT generate a random number inside the compare function. Rather, you will write another function named getRandom of int return type to do it. You will need to call getRandom from compare, no parameters are necessary. Inside your main function, get the guess from user and pass it to the compare function. Code: #include <iostream>using namespace std; int getRandom(){ //generate seeded random num. //in between 1-100 inclusive //return random number } void compare(int guess){ //call getRandom //compare guess with random number //inform user what…arrow_forward
- Alert: Don't submit AI generated answer and please submit a step by step solution and detail explanation for each steps. Write a python program using functionsarrow_forward5. use c code to Write a stringSearch function that gets two strings one called needle and the other called haystack. It then searches in the Haystack for the needle. If it finds it, it returns the index of where the needle starts in the haystack. If the needle cannot be found, it should return -1 Prototype: int stringSearch(char needle[], char haystack) Example1: Haystack: “This is just an example” Needle: “just” Result: 8 Example2: Haystack: “This is just an example” Needle: “This” Result: 0 Example3: Haystack: “This is just an example” Needle: “this” Result: -1 Hint: all the answer need to include an output and use c code to answerarrow_forward4. Complete the function show_upper. This function takes one parameter - a string (s). It should return a string made up of all the upper-case characters in s. For example, if s is “aBdDEfgHijK” then show_upper should return “BDEHK”. It should return the upper-case string - not print it. Do not change anything outside show_upper.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
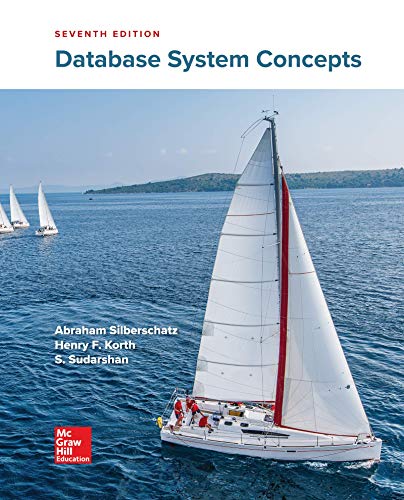
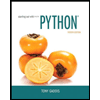
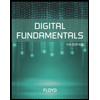
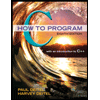
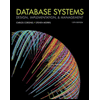
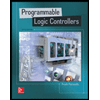