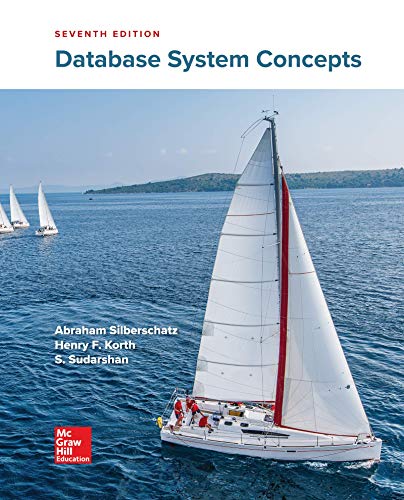
PLEASE DO THIS IN PYTHON
Part 4 - Performance of binary_search
Modify your binary_search function to count the number of comparisons (==, <, <=, >, or >=). The function will now return both the number of comparisons made and the True or False result.
The code below calls your function, and creates a simple ASCII bar chart of the number of comparisons (divided by 10, to account for small differences).
Test Code
max_elements = 100000 for length in range(1, max_elements, 10000): values = list(range(1, (2 * length) + 1, 2)) num_comparisons, found = binary_search(values, length + 1, 0, len(values) - 1) num_comparison_string = format(num_comparisons, '03d') print(num_comparison_string, '*' * (num_comparisons // 10))
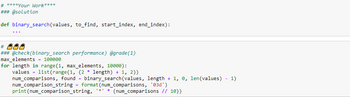

Performance of binary search
Below code modify binary_search function to count the number of comparison.
Step by stepSolved in 4 steps with 1 images

- Please use PYTHON def count_scrabble_points(user_input): """ The function ... """ tile_dict = { 'A': 1, 'B': 3, 'C': 3, 'D': 2, 'E': 1, 'F': 4, 'G': 2, 'H': 4, 'I': 1, 'J': 8, 'K': 5, 'L': 1, 'M': 3, 'N': 1, 'O': 1, 'P': 3, 'Q': 10, 'R': 1, 'S': 1, 'T': 1, 'U': 1, 'V': 4, 'W': 4, 'X': 8, 'Y': 4, 'Z': 10 } if __name__ == "__main__": ''' Type your code here. ''' Thank you!arrow_forward*Coding language is Python Write a program that opens the productsales.txt file and reads the sales into a list. The program should output the following information, in this order: The total of all sales in the list The average of all sales in the list The lowest sale in the list The highest sale in the list NOTE: Your program should include at least one user-defined function, in addition to the main function. 4147 1594 2235 8433 10000 129 5555 7030 9764 7465 1111 4444 8954 2243 2895 1436 4978 5486 1436 9846 4789 8456 2497 2280 6375arrow_forwardpython exercise Given the following list: testlist2 = (23,50,12,80,10,90,34,78,4,19,53) Use testlist2 to create Numpy array of int16 and then display the array. Display the number of dimension of testnp. Display the shape of testnp. Display the number of bytes of testnp. Display the mean, sum, max, and min of testnp.arrow_forward
- In cell C18 type a VLOOKUP function to find the corresponding letter grade (from column D) for the name in A18. The table array parameter is the same as in B18. Type FALSE for the range lookup parameter. Copy the formula in C18 to C19:C22Notice this formula works correctly for all cells. Range lookup of FALSE means do an exact match on the lookup value whether or not the table array is sorted by its first column. In cell E11, type an IF function that compares the score in B11 with the minimum score to pass in A5. If the comparison value is true, display Pass. Otherwise, display Fail. Copy the formula in E11 to E12:E15. Did you use appropriate absolute and relative references so the formula copied properly? In cell F11, type an IF function that compares the grade in D11 with the letter F (type F). If these two are not equal, display Pass. Otherwise, display Fail. Copy the formula in F11 to F12:F15arrow_forwardMust be written in the coral language. Complete the PrintArray function to iterate over each element in dataValues. Each iteration should put the element to output. Then, put "*" to output. Ex: If dataValue's elements are 2 4 7, then output is: 2*4*7*arrow_forwardPython question Application: Big-O Notation (Q8-11) For each of the time complexities in this segment give the tightest bound in terms of a simple polylogarithmic function using big-O notation. Note: use the ‘^’ symbol to indicate exponents, i.e., write O(n^2) for O(n2). Question 8 (Big-O Notation 1) T(n) = n2+ log n + n Question 9 (Big-O Notation 2) T(n) = n/3 + 4 log n + 2n log(n) Question 10 (Big-O Notation 3) T(n) = 7n5 + 2n Question 11 (Big-O Notation 4) T(n) = (n%5) + 12,000arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
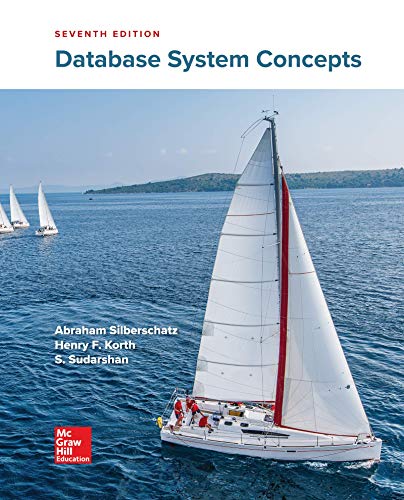
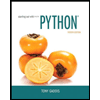
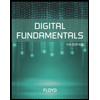
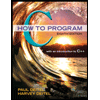
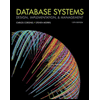
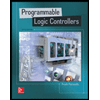