Please edit this class Authenticator. Remove breaks. Also create user array of size 100 rather than 3 different arrays. Also edit so it can be more OOP import java.util.Scanner; public class AuthenticatorApp { public static void main(String[] args) throws Exception { Scanner input=new Scanner(System.in); Authenticator obj=new Authenticator("user.data"); String Username,Password; boolean done=false; int count=0; while(count<3) { System.out.print("Username? "); Username=input.next(); System.out.print("Password? "); Password=input.next(); obj.authenticate(Username,Password); count++; } System.out.print("Too many failed attempts... please try again later"); input.close(); } } ------------------------------------------------------------------------------------------------------------ import java.io.File; import java.io.FileNotFoundException; import java.util.NoSuchElementException; import java.util.Scanner; class Authenticator { int size; String uname[]; String pwd[]; String hint[]; //constructor Authenticator(String filename) throws FileNotFoundException { uname=new String[100]; pwd=new String[100]; hint=new String[100]; File file=new File(filename); Scanner sc=new Scanner(file); int pos = 0; while(sc.hasNextLine()) { String line=sc.nextLine(); String array[]=line.split("\\s"); uname[pos]=array[0]; pwd[pos]=array[1]; hint[pos]=array[2]; pos++; } size=pos; sc.close(); } void authenticate(String username, String password) { int flagU=1,count=1; int i; for( i=0;i
Please edit this class Authenticator. Remove breaks. Also create user array of size 100 rather than 3 different arrays. Also edit so it can be more OOP
import java.util.Scanner;
public class AuthenticatorApp {
public static void main(String[] args) throws Exception {
Scanner input=new Scanner(System.in);
Authenticator obj=new Authenticator("user.data");
String Username,Password;
boolean done=false;
int count=0;
while(count<3)
{
System.out.print("Username? ");
Username=input.next();
System.out.print("Password? ");
Password=input.next();
obj.authenticate(Username,Password);
count++;
}
System.out.print("Too many failed attempts... please try again later");
input.close();
}
}
------------------------------------------------------------------------------------------------------------
import java.io.File;
import java.io.FileNotFoundException;
import java.util.NoSuchElementException;
import java.util.Scanner;
class Authenticator
{
int size;
String uname[];
String pwd[];
String hint[];
//constructor
Authenticator(String filename) throws FileNotFoundException
{
uname=new String[100];
pwd=new String[100];
hint=new String[100];
File file=new File(filename);
Scanner sc=new Scanner(file);
int pos = 0;
while(sc.hasNextLine())
{
String line=sc.nextLine();
String array[]=line.split("\\s");
uname[pos]=array[0];
pwd[pos]=array[1];
hint[pos]=array[2];
pos++;
}
size=pos;
sc.close();
}
void authenticate(String username, String password)
{
int flagU=1,count=1;
int i;
for( i=0;i<size;i++)
{
if(uname[i].equals(username))
{
flagU=0;
break;
}
}
if(flagU==1)
{
try {
throw new Exception("UserName Not Exist");
} catch (Exception e) {
System.out.println(e.getMessage());
return;
}
}
boolean f= verifyPassword(password,i);
if(f)
{
System.out.println("Welcome to the system");
System.exit(0);
}
else
{
try {
throw new Exception("*** Invalid password - hint: "+hint[i]);
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
private boolean verifyPassword(String password,int index) {
if(pwd[index].equals(password))
return true;
return false;
}
}
here are some sample excutions of the program:
username? arnow
password? java
Welcome to the system
Sample Test Run #2
Given the same users.data file as above, execution of the program should look like:
username? weiss
password? dontremember
*** Invalid password - hint: woof-woof
username? weiss
password? puppy2
Welcome to the system
Sample Test Run #3
Given the same users.data file as above, execution of the program should look like:
username? sokol
password? CUNY
*** Invalid password - hint: college
username? sokol
password? SUNY
*** Invalid password - hint: college
username? sokol
password? BC
*** Invalid password - hint: college
Too many failed attempts... please try again later
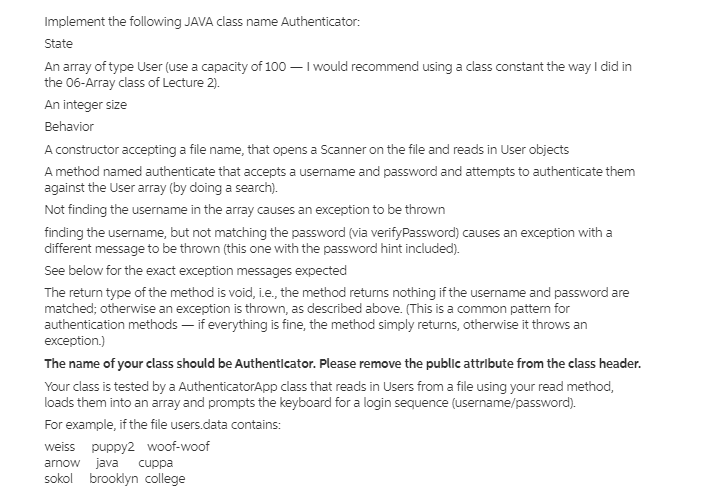

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

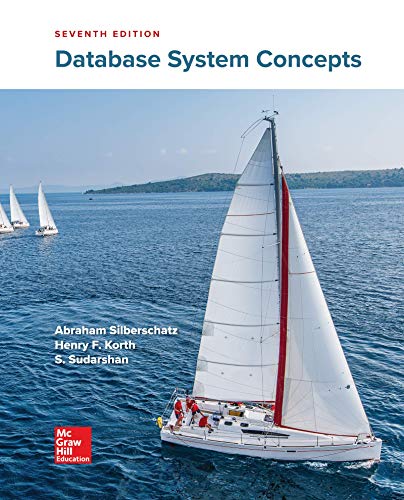
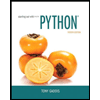
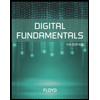
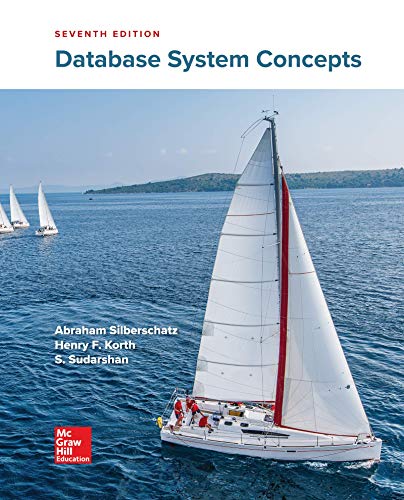
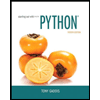
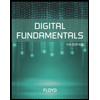
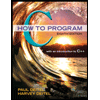
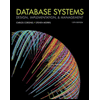
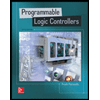