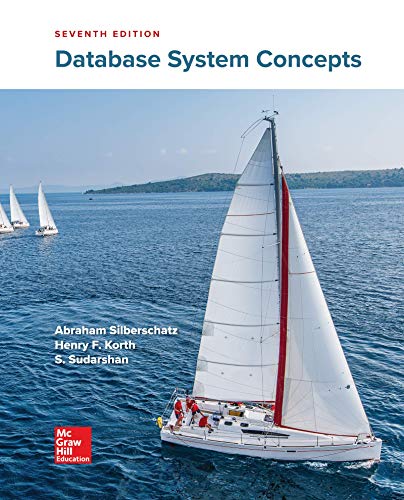
Implement a method named “randomArray” in the file named “BSTTest.java” that tests a specific sorting
- size of a randomly generated array (int): e.g., 10
This method will:
- Generate and return a random array of the length specified by the parameter
- The value range of each element should be between 0 and 1000 (inclusive)
>>> randomArray(5).
>>> [1, 2, 3, 7, 10]
Implement a method named “test” in the file named “BSTTest.java”. This method should take advantage of the implemented randomArray and print out information as such:
Testing of BST starts.
Insert 3
Insert 2
Insert 5
Insert 100
Delete 3.
The traverse gives a BST as 3, 5, 100
Testing of BST ends.
Please note that the goal is not to manually code the printings of each line. Try your best to make this method as automatic as possible. There are no limits on what parameters this method shall accept or what it returns. However, please make sure to use the method to perform testing for at least two cases in your main method of BSTTest.java.
Please use the following code as a base.
import java.util.*;
public class BSTTest{
public static void main(String[] args) {
// perform at least one test on each operation of your BST
}
private int[] randomArray(int size) {
// remove the two lines
int[] arr = new int[1];
return arr;
}
// the parameters and return are up to you to define; this method needs to be uncommented
// private test() {
//
// }
}

Step by stepSolved in 3 steps with 2 images

- write a program that reads the students.txt file and stores the name of the student and the grade information as a student object in an arraylist sorts the students names based on their first grade in decreasing order using the selection sort algorithm writes the sorted list as the students last name , middle name (if theres one), first name , and grade information into a text file output example: Robison, Lee 95 80 Green, Alex R 85 60 Waston, Zoe G 100 80 use javaarrow_forwardWrite a Java program that will allow the user to play a hangman game repeatedly until theychoose to stop. The game should randomly choose a secret word from a pre-set list of wordsfor the user to guess. Store the list of words available for play in an array so you can randomlychoose them by index during game play.During a turn, the user will guess one letter at a time. Each letter in the secret word shoulddisplay as an asterisk until the letter is guessed by the user. When a correct letter is guessed,the letter should be displayed in the correct position in place of the asterisk. The user hangshimself with the 8th wrong guess and loses the game.Make a character array to hold the characters and asterisks representing the word beingguessed. You can change the contents of this array as the letters are guessed and revealed. Inother words, this array can be used to display the current state of the secret word each round.Here is the list of words the game should choose from for the secret…arrow_forwardMethods Details: public static java.lang.StringBuffer[] getArrayStringsLongerThan(java.lang.StringBuffer[] array, int length) Returns a StringBuffer array with COPIES of StringBuffer objects in the array parameter that have a length greater than the length parameter. If no strings are found an empty array will be returned. Parameters: array - length - Returns: Throws:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forward
- A method named getUserStringsAndPopulate Array(). This method should keep prompting the user to enter as many strings as the user wants. The user should be able to stop entering strings by entering EXIT. This method should take the strings entered by the user and populate a one-dimensional array of strings with the user input. The string EXIT should NOT be added to the array because it is a special flag that will tell the method to stop prompting the user to enter more strings. This method should return the one-dimensional array of strings populated with user input and should not accept any arguments. 2.b) A method named searchArray() that would accept a one-dimensional array of strings and search the array for the string Winter. The search logic should NOT be case sensitive. If a match is found, this method should print the string in the array followed by a message like " --- Found a match!". Otherwise, the method should not print anything. This method should NOT return anything.…arrow_forwardWrite a method swapArrayEnds() that swaps the first and last elements of its array parameter. Ex: sortArray = {10, 20, 30, 40} becomes {40, 20, 30, 10}. The array's size may differ from 4. new code can only be added between existing code. as seen in image.arrow_forwardThe programming language used is Javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
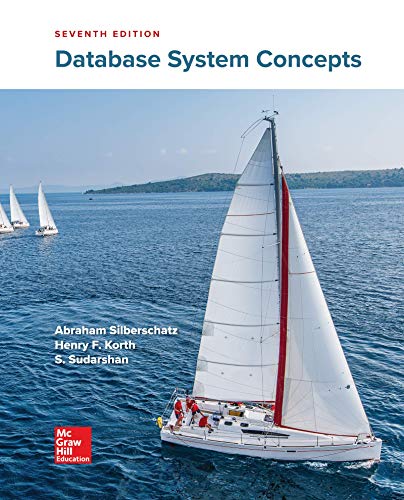
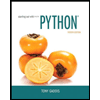
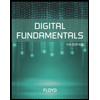
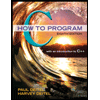
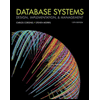
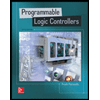