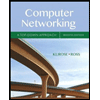
JAVA programming
I have to finish the "remove" method which is supposed to remove element e at index ind and returns it.
Ill paste the entire code below, but i only have to complete the "remove" method.
CODE:
package com.mac286.arrays;
public class ourArray {
//define an array of integers
private int[] Ar; //Ar is a reference
//declare
private int Capacity = 100;
private int size = 0;
private int Increment = 10;
//Default Constructor
public ourArray() {
Ar = new int[100];
Capacity = 100;
size = 0;
Increment = 10;
}
//Constructor that accepts the capacity (C). It creates an array of C integersm, sets capacity to C
// Increment to 10
public ourArray(int C) {
//create an array of C integers
Ar = new int[C];
size = 0;
Capacity = C;
Increment = 10;
}
//Constructor that accepts two integers (c, i) c for capacity and i for increment
public ourArray(int C, int I) {
//create an array of C integers
Ar = new int[C];
size = 0;
Capacity = C;
Increment = I;
}
//setter for Increment
public void setIncrement(int I) {
Increment = I;
}
//getter for size, capacity and increment.
public int getSize() {
return size;
}
public int getCapacity() {
return Capacity;
}
public int getIncrement() {
return Increment;
}
//- A method void add(int e); it adds e to the end of the array.
// A.add(-1); A.add(-5), A.add(-9) [-1, -5, -9]
public void add(int e) {
//add only if there is space
if(size >= Capacity) {
System.out.println("The array is full");
//get out
System.exit(1);
}
//if you are here it is not full
Ar[size] = e;
size++;
}
//isEmpty returns true if it is empty and false it it is not.
public boolean isEmpty() {
return (size == 0);
}
public String toString() {
if(this.isEmpty())
return "[]";
String st = "[";
//build your string [-1, -3, -9]
for(int i = 0; i < size-1; i++) {
//in the loop your are always dealing with element i
//v element i is Ar[i]
//append Ar[i], to the string
st += Ar[i] + ", ";
}
//close the bracket
st += Ar[size-1] + "]";
return st;
}
public void addFront(int e) {
//check there is space
if (size >= this.Capacity) {
System.out.println("Array is full");
System.exit(1);
}
//bring all values up by one
for(int i = size; i > 0; i--){
Ar[i] = Ar[i-1];
}
//add e to index 0
Ar[0] = e;
size++;
}
//remove removes the last item and returns it
public int remove() {
if(this.isEmpty()) {
System.out.println("Error: the array is empty");
return -1;
}
int save = Ar[size-1]; //save the last element to be returned
//delete by just reduing the size
size--;
//return the removed item
return save;
}
//removeFront() removes the first item and returns it
//removeFront() removes the first item and returns it
public int removeFront() {
if(this.isEmpty()) {
System.out.println("Error: the array is empty");
return -1;
}
int save = Ar[0];
//bring the rest down by one (size-1)( element down)
for(int i = 0; i < size-1; i++) {
Ar[i] = Ar[i+1];//if youy use size instead of size-1 you may get
//outofbounds exception
}
size--;
return save;
}
//This method will add element e at index ind
public void add(int ind, int e) {
if (size >= this.Capacity) {
System.out.println("Array is full");
System.exit(1);
}
if(ind == 0)
this.addFront(e);
else if (ind == size)
this.add(e);
else if(ind < 0 || ind > size) {
System.out.println("Error: cannot add at those indices");
return;
}
//bring all values up by one
for(int i = size; i > ind; i--){
Ar[i] = Ar[i-1];
}
//add e to index 0
Ar[ind] = e;
size++;
}
//int remove(intind) removes element e at index ind and returns it.
public int remove(int ind) {
return 0;
}
}

![package com.mac286.arrays;
public class Tester {
public static void main(String [] args) {
//create an object ourArray
//className objectName = new className();
ourArray A = new ourArray();
+ A.getSize() +
+ A.getIncrement ());
System.out.println("size:
//display while empty
System.out.println("size:
//add -3, -1, -9
A.add(-3);
A.add (-1);
A.add(-9);
Increment:
+ A.getSize() +
+ A.toString(0);
%3D
:
//show the content
System.out.println("size:
A.addFront (-13);
System.out.println("size:
A.addFront(-25);
System.out.println("size:
System.out.println("removing:
System.out.println("size:
System.out.println("removing:
System.out.println("size:
+ A.getSize() + "
" + A.toString());
:
+ A.getSize() +
+ A.tostring(0);
:
+ A.getSize() +
+ A. remove();
+ A.getSize(0 +
+ A. removeFront());
+ A.getSize() + " :
+ A.toString(0);
:
+ A. toString 0);
+ A.toString(0);
%3D
}
}](https://content.bartleby.com/qna-images/question/33eba9e5-eb7d-44a1-93e3-ed0ee897e17f/9624f872-ad21-4d20-8cc5-89fac31f59d2/r087vcp_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- JAVA programming language i need to create an "addFront" method in the bottom of the code that adds an element at the front. [-1, -3, -5] A.add(-9) ===> [-9, -1, -3, -5]. Its all the way in the bottom, please help me. public class ourArray { //define an array of integers private int[] Ar; //Ar is a reference //declare private int capacity = 100; private int size = 0; private int increment = 10; //default constructor public ourArray() { Ar = new int[100]; capacity = 100; size = 0; increment = 10; } //Constructor that accepts the capacity (C). It creates an array of C integersm, sets capacity to C // Increment to 10 public ourArray(int C) { Ar = new int [C]; size = 0; capacity = C; increment = 10; } //Constructor that accepts two integers (c, i) c for capacity and i for increment public ourArray (int C, int I) { Ar = new int[C]; size = 0; capacity = C; increment = I; } //setter for Increment public void setincrement(int I) { increment = I; } //getter for size, capacity…arrow_forwardLanguage: Javaarrow_forwardThe files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. // Application sums and averages an array of integers public class DebugEight2 { publicstaticvoidmain(Stringargs[]) { int[] someNums = {4,17,22,8,35}; int tot =0; int x; for(x =1; x > someNums.length; ++x) tot = someNums[x]; System.out.println("Sum is " + tot); System.out.println("Average is " + tot * 1.0 / someNums.length); } }arrow_forward
- assignment is to complete the starter code:arrow_forward1). Create the class called ArrayOperations2D and include the method findRowMax below public class ArrayOperations2D { public static intl] findRowMax (int [][] anArray) int [] max = new int [anArray. length]; //for each row for (int i=0; i< anArray. length; i++) int largest = anArray [1][0]; for (int i=0; < anArray [i].langthi i++) if (anArray[i](j] > largest) largest = anArray[illjl: max [i]=largest; } return max; 2). Include the method called findRowSums below public static int[] findRowSums (int [] [] anArray) int [] rowSums = new int [anArray.length]; for (int i=0; i< anArray.length; i++) int sum = 0; for (int j=0; j<anArray(jl.length; J++) sum+=anArray [i][jl; rowSums [1] =sum; } return rowSums; 3). Add a method called findColumnMax to the class. This method should find and return the maximum value in each column of the two dimensional array 4). Add a method called findColumnSum that calculates and returns the sum of each column. 5). Add a method called…arrow_forward•• 8. Write a method that returns the sum of a given row in a two-dimensional array. Complete this code: ArrayUtil.java 1 public class ArrayUtil 2 { /** Computes the sum of a given row in a two-dimensional array. @param values the array @param the row whose sum to compute @return the sum of the given row */ public static int rowSum(int[][] values, int row) { 3 4 8 10 11 } 13 } 12 RowSumTester.java 1 public class RowSumTester 2 { public static void main(String[] args) { 4 6. int[][] counts = { 1, 0, 1 }, { 1, 1, 0 }, { 0, 0, 1 }, { 1, 0, 0 }, { 0, 1, 1 }, { 0, 1, 1 }, { 1, 1, 0 } 10 11 12 13 14 }; int sum = ArrayUtil.rowSum(counts, 5); 15 16 System.out.println(sum); System.out.println("Expected: 2"); 17 18 19 int[][] magicSquare = { { 16, 3, 2, 13 }, { 5, 10, 11, 8 }, { 9, 6, 7, 12 }, { 4, 15, 14, 1 }, }; 20 21 22 23 24 25 26 for (int row = 0; row <= 3; row++) { System.out.println(ArrayUtil. rowSum(magicSquare, row)); System.out.println("Expected: 34"); } 27 28 29 30 31 32 33 }arrow_forward
- JAVA Program Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an employee’s identification…arrow_forwardJAVA PROGRAM Homework #1. Chapter 7. PC# 2. Payroll Class (page 488-489) Write a Payroll class that uses the following arrays as fields: * employeeId. An array of seven integers to hold employee identification numbers. The array should be initialized with the following numbers: 5658845 4520125 7895122 8777541 8451277 1302850 7580489 * hours. An array of seven integers to hold the number of hours worked by each employee * payRate. An array of seven doubles to hold each employee’s hourly pay rate * wages. An array of seven doubles to hold each employee’s gross wages The class should relate the data in each array through the subscripts. For example, the number in element 0 of the hours array should be the number of hours worked by the employee whose identification number is stored in element 0 of the employeeId array. That same employee’s pay rate should be stored in element 0 of the payRate array. The class should have a method that accepts an employee’s identification…arrow_forwardPlease write it in c# program. Problem: BigNumberInteger numbers in programming limit how large numbers can be processed, so double types come tothe rescue. Sometimes we need to implement bigger values. Create a class BigNumber that uses a 40-element array of digits to store integers as large as 40 digits each. Provide methods Input, ToString, Addand Subtract. For comparing BigNumber objects, provide the following methods: IsEqualTo,IsNotEqualTo, IsGreaterThan, IsLessThan, IsGreaterThanOrEqualTo and IsLessThanOrEqualTo. Each ofthese is a method that returns true if the relationship holds between the two BigNumber objects andreturns false if the relationship does not hold. Provide method IsZero. In the Input method, use thestring method ToCharArray to convert the input string into an array of characters, then iterate throughthese characters to create your BigNumber. (Add Multiply and Divide methods For extra five points innext exam)Hint: use char –‘0’ to convert character to…arrow_forward
- Method Details: public static java.lang.String getArrayString(int[] array, char separator) Return a string where each array entry (except the last one) is followed by the specified separator. An empty string will be return if the array has no elements. Parameters: array - separator - Returns: stringThrows:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forwardHelparrow_forwardin java Integer numVals is read from input and integer array userCounts is declared with size numVals. Then, numVals integers are read from input and stored into userCounts. If the first element is less than the last element, then assign Boolean firstSmaller with true. Otherwise, assign firstSmaller with false. Ex: If the input is: 5 40 22 41 84 77 then the output is: First element is less than last element 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 import java.util.Scanner; public class UserTracker { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intnumVals; int[] userCounts; inti; booleanfirstSmaller; numVals=scnr.nextInt(); userCounts=newint[numVals]; for (i=0; i<userCounts.length; ++i) { userCounts[i] =scnr.nextInt(); } /* Your code goes here */ if (firstSmaller) { System.out.println("First element is less than last element"); } else { System.out.println("First element is not less…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
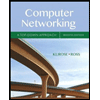
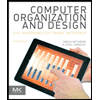
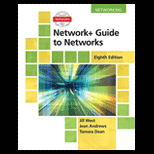
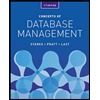
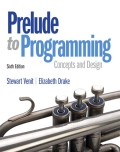
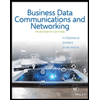