Provide a method that swaps the values with the smallest and largest measure in an array of objects of a class that implements the Measurable interface. public class Measurables { /** Swaps the values with the smallest and largest measure. @param objects an array of objects of a class that implements the Measurable interface. */ public static void swapMinAndMax(Measurable[] objects) { /* code goes here */ } }
Provide a method that swaps the values with the smallest and largest measure in an array of objects of a class that implements the Measurable interface.
**
A bank account has a balance that can be changed by
deposits and withdrawals.
*/
public class BankAccount implements Measurable
{
private double balance;
/**
Constructs a bank account with a zero balance.
*/
public BankAccount()
{
balance = 0;
}
/**
Constructs a bank account with a given balance.
@param initialBalance the initial balance
*/
public BankAccount(double initialBalance)
{
balance = initialBalance;
}
/**
Deposits money into the bank account.
@param amount the amount to deposit
*/
public void deposit(double amount)
{
balance = balance + amount;
}
/**
Withdraws money from the bank account.
@param amount the amount to withdraw
*/
public void withdraw(double amount)
{
balance = balance - amount;
}
/**
Gets the current balance of the bank account.
@return the current balance
*/
public double getBalance()
{
return balance;
}
public double getMeasure()
{
return balance;
}
public String toString()
{
return "BankAccount[balance=" + balance + "]";
}
}
/**
A country with a name and area.
*/
public class Country implements Measurable
{
private String name;
private double area;
/**
Constructs a country.
@param aName the name of the country
@param anArea the area of the country
*/
public Country(String aName, double anArea)
{
name = aName;
area = anArea;
}
/**
Gets the country name.
@return the name
*/
public String toString()
{
return name;
}
/**
Gets the area of the country.
@return the area
*/
public double getArea()
{
return area;
}
public double getMeasure()
{
return area;
}
/**
Describes any class whose objects can be measured.
*/
public interface Measurable
{
/**
Computes the measure of the object.
@return the measure
*/
double getMeasure();
}
}
import java.util.Arrays;
public class Tester
{
public static void main(String[] args)
{
Measurable[] countries = new Measurable[3];
countries[0] = new Country("Uruguay", 176220);
countries[1] = new Country("Thailand", 514000);
countries[2] = new Country("Belgium", 30510);
Measurables.swapMinAndMax(countries);
System.out.println(Arrays.toString(countries));
System.out.println("Expected: [Uruguay, Belgium, Thailand]");
System.out.println();
Measurable[] objects = {
new BankAccount(1000),
new BankAccount(3000),
new BankAccount(2000),
};
Measurables.swapMinAndMax(objects);
System.out.println(Arrays.toString(objects));
System.out.println("Expected: [BankAccount[balance=3000.0], "
+ "BankAccount[balance=1000.0], BankAccount[balance=2000.0]]");
System.out.println();
Measurable[] objects2 = { new Country("Uruguay", 176220) };
Measurables.swapMinAndMax(objects2);
System.out.println(Arrays.toString(objects2));
System.out.println("Expected: [Uruguay]");
}
}
Provide a method that swaps the values with the smallest and largest measure in an array of objects of a class that implements the Measurable interface.
public class Measurables
{
/**
Swaps the values with the smallest and largest measure.
@param objects an array of objects of a class that implements the
Measurable interface.
*/
public static void swapMinAndMax(Measurable[] objects)
{
/* code goes here */
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

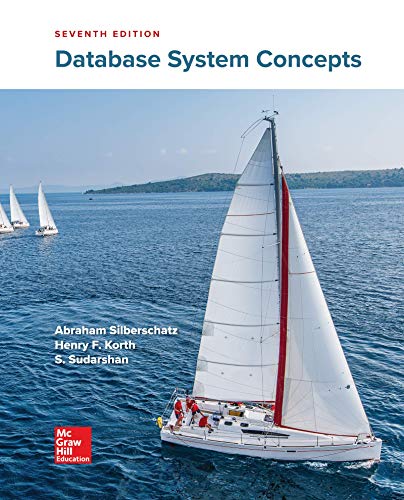
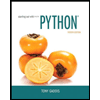
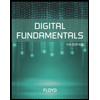
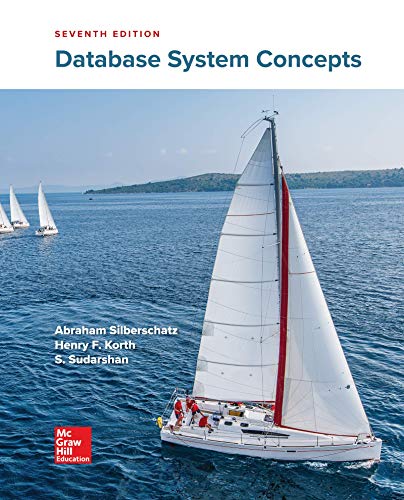
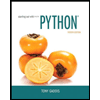
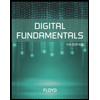
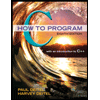
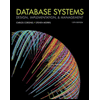
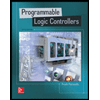