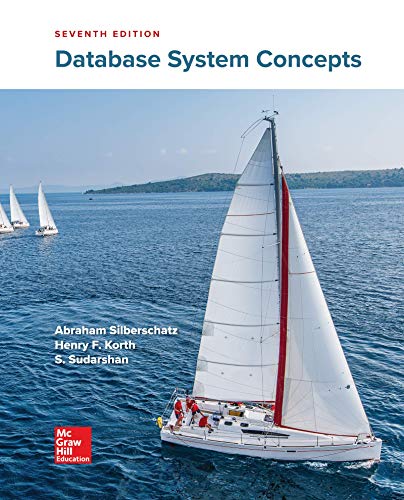
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![```java
public class PS07D
{
/**
* Write the method catch22().
*
* Given an array of ints, return
* true if the array contains a 2
* next to a 2, anywhere in the array.
*
* Examples:
* catch22({1, 2, 2}) returns true
* catch22({1, 2, 1, 2}) returns false
* catch22({2, 1, 2}) returns false
*
* @param nums the array to search.
* @return true if two adjacent 2s are in the array.
*/
// TODO - Write the catch22 method here.
public boolean catch22(int[] nums)
{
return false;
}
}
```
### Explanation
- **Class Declaration**: The code defines a public class named `PS07D`.
- **Method Description**: The method `catch22()` is described using a comment block. This method is tasked with checking an integer array for two adjacent '2' values.
- **Examples**: The comments provide examples of how the method should behave:
- `catch22({1, 2, 2})` returns `true`
- `catch22({1, 2, 1, 2})` returns `false`
- `catch22({2, 1, 2})` returns `false`
- **Parameters and Return Values**:
- **@param nums**: Describes the parameter `nums` as the array to search.
- **@return**: Specifies that the method returns a boolean value (`true` or `false`) based on the presence of adjacent '2's.
- **Method Definition**: The placeholder method `catch22` is defined as returning a `boolean` value. It accepts an integer array `nums` as a parameter. Currently, it always returns `false`, indicating that the implementation is incomplete and needs to be written.](https://content.bartleby.com/qna-images/question/07c38dc6-4a9f-400d-9784-ec1cee64c4c5/311d0bd2-e51f-4ccb-a256-34be075d9279/zq333kg_thumbnail.png)
Transcribed Image Text:```java
public class PS07D
{
/**
* Write the method catch22().
*
* Given an array of ints, return
* true if the array contains a 2
* next to a 2, anywhere in the array.
*
* Examples:
* catch22({1, 2, 2}) returns true
* catch22({1, 2, 1, 2}) returns false
* catch22({2, 1, 2}) returns false
*
* @param nums the array to search.
* @return true if two adjacent 2s are in the array.
*/
// TODO - Write the catch22 method here.
public boolean catch22(int[] nums)
{
return false;
}
}
```
### Explanation
- **Class Declaration**: The code defines a public class named `PS07D`.
- **Method Description**: The method `catch22()` is described using a comment block. This method is tasked with checking an integer array for two adjacent '2' values.
- **Examples**: The comments provide examples of how the method should behave:
- `catch22({1, 2, 2})` returns `true`
- `catch22({1, 2, 1, 2})` returns `false`
- `catch22({2, 1, 2})` returns `false`
- **Parameters and Return Values**:
- **@param nums**: Describes the parameter `nums` as the array to search.
- **@return**: Specifies that the method returns a boolean value (`true` or `false`) based on the presence of adjacent '2's.
- **Method Definition**: The placeholder method `catch22` is defined as returning a `boolean` value. It accepts an integer array `nums` as a parameter. Currently, it always returns `false`, indicating that the implementation is incomplete and needs to be written.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- public class ArrayCommonOperations{ public static void main(String [] args) { //*** Task #1: Define and instantiate an array of integer numbers, with 10 elements //*** Task #2: Fill in the array with integer numbers from 1 to 100 //*** Task #3: define another array, named temp, and copy the initial array in it. //* This allows to preserve the original array //*** Task #4: Define the variables you need to calculate the following values, //* and initialize them with appropriate values. //*** Task #5: Print the original array //*** Task #6: Calculate the sum of all values //*** Task #7: Count the number of even values //*** Task #8: Calculate the minimum value in the array //*** Task #9: Calculate the maximum value in the array //*** Task #10: Replace the elements that are divisible by 3, with their value plus 2 //*** Task #11:…arrow_forwardin java Create two arrays with same length n: - Array A with integer numbers that user will insert- Array B with random numbers from 0 to 100.- Find sum of both arrays,- Find maximum values of both array and compare them: .if maximum of 1 st is lower then maximum of 2nd array: print sums of both arrays.if maximum of 1st is greater then maximum of 2nd array print 2nd array (use println)otherwise print first array in reverse.arrow_forwardComplete the method endsInFive that takes as arguments a 2D-array of integers and an integer value that represents a column index in the array. endsInFive returns the number of values in the column that end in 5. It is possible that the array is ragged, meaning that the number of columns in each row is different. For example, 2 is returned if the following 2D-array is passed as the first argument of endsInFive and the integer 3 as the second argument (the values 25 and 115 end in five at column index 3).arrow_forward
- /*In this program you need to implement four methods with the following signatures:printArr(int[] a)add(int[] a, int[] b)subtract(int[] a, int[] b)negate(int[] a) 1. Method printArr(a) should print out all elements of a given int array in one line. 2. Method add(a, b) should take two int arrays (assume they have the same length) and return a new array each element of which will be the sum of the corresponding elements in a and b 3. Method subtract(a, b) is similar to add(), except it returns (a - b) array 4. Method negate(a)takes an int array and returns a new array in which every element is multiplied by -1. */ public class ArrayOperations{ public static void main(String[] args){ //The main only has the testing code. //You don't have to change it. int [] a = { 1, 2, 3, 4, 5}; int [] b = {10, 1, 3, 5, 7}; System.out.print("a = "); printArr(a);//Should print each element of a (in one line) System.out.print("b = ");…arrow_forwardPlease send me answer of this question immediately and i will give you like sure sirarrow_forwardMethod Details: public static java.lang.String getArrayString(int[] array, char separator) Return a string where each array entry (except the last one) is followed by the specified separator. An empty string will be return if the array has no elements. Parameters: array - separator - Returns: stringThrows:java.lang.IllegalArgumentException - When a null array parameter is providedarrow_forward
- Method Details getCountInRange public static int getCountInRange(int[] array, int lower, int upper) Returns the number of values in the range defined by lower (inclusive) and upper (inclusive) Parameters: array - integer array lower - lower limit upper - upper limit Returns: Number of values found Throws: java.lang.IllegalArgumentException - if array is null or lower is greater than upper Any error message is fine (e.g., "Invalid parameters(s)")arrow_forwardPlease help with this java problemarrow_forwardParagraph Styles Editing 5. Given the following series of n integers:1+2+3++n-2+n-1+nWrite a Java method that returns the sum of the series. 6. Given the following function:f(x)-2xFor, determine 7. Consider an array that stores the following sequence of integers:10, 15, 20, 25, 30Write a Java method that returns the sum of the integers stored in the array. The array must be passed to the method as an argument. I OFocus Earrow_forward
- In javacode: Use ArrayList to create an array called myAL of type integer. --Fill the array with the values 5, 10, 15, 22, 33. --Print the array (use enhanced for loop). --Insert the value 25 between 10 and 15 and print the array.--Remove 2 elements on index 1 and 3 and then print the array.--Print if the array contains the value 123 or not.--Print the index of the element 22.--Print the size of the array.arrow_forwardfor the class ArrayAverage write a code that calculates the average of the listed numbers with a double result for the class ArrayAverageTester write a for each loop and print out the resultarrow_forward/*** creates an array of 1000 integers where each element matches its index* * @return the array that is created*/public static int[] makeNumberArray() {arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
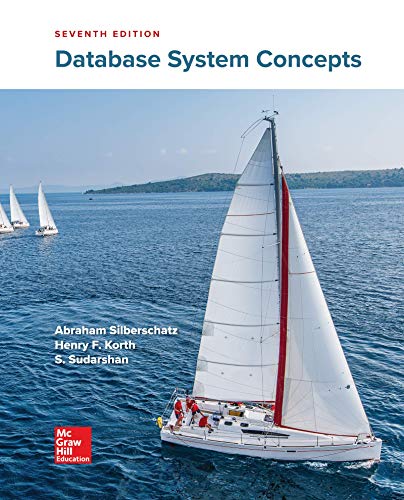
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
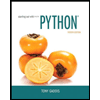
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
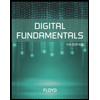
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
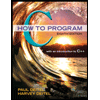
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
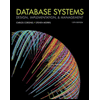
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
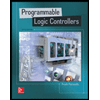
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education