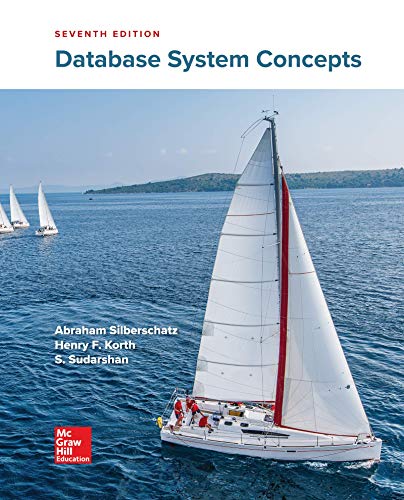
Concept explainers
- Plot the output in an Excel graph. Explain your findings. Adjust your inputs to get meaningful outputs.
//Write a program that simulates a paging system using the aging
//frames is a parameter. The sequence of page references should be read from a file.
//For a given input file, plot the number of page faults per 1000 memory references as a function of
//the number of page frames available.
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
//THE PAGE FREQUENCY PER 1000 MEMORY REFERENCE DATA IS STORED IN A SEPARATE FILE CALLED DATA.TXT.
//DATA POINTS CAN BE USED TO PLOT THE GRAPH
void AgeIncrease (int frames);
int OldestIndex (int frames);
struct PageTable {
int frame_number;
bool valid;
}*PageTable;
struct FrameTable {
int page_number;
int age;
}*FrameTable;
int main (void){
printf("Number of pages:\n");
int pages;
scanf("%d", &pages);
printf("Number of frames:\n");
int frames;
scanf("%d", &frames);
PageTable=malloc(sizeof(PageTable)*pages);
FrameTable=malloc(sizeof(FrameTable)*frames);
int i;
for (i=0;i<pages; i++){
PageTable[i].valid=false;
}
for (i=0;i<frames;i++){
FrameTable[i].age=0;
}
FILE *fp=fopen("file.txt", "r");
int request;
int frame_table_counter=0;
int page_fault_counter=0;
int request_counter=0;
int memory_ref_counter=0;
//add frame size to page faults later
while (!feof(fp)){
fscanf(fp, "%d", &request);
request_counter++;
if (PageTable[request].valid==false){
FrameTable[frame_table_counter].page_number=request;
PageTable[request].valid=true;
PageTable[request].frame_number=frame_table_counter;
frame_table_counter++;
page_fault_counter++;
memory_ref_counter++;
}
if (request_counter==1000){
FILE *fp=fopen("data.txt", "a");
fprintf (fp, "%d\n", memory_ref_counter);
memory_ref_counter=0;
request_counter=0;
fclose(fp);
}
if (frame_table_counter==frames){
break;
}
}
while (!feof(fp)){
fscanf(fp, "%d", &request);
request_counter++;
AgeIncrease(frames);
if (PageTable[request].valid==false){
int oldest_page=OldestIndex(frames);
PageTable[FrameTable[oldest_page].page_number].valid=false;
FrameTable[oldest_page].page_number=request;
FrameTable[oldest_page].age=0;
PageTable[request].frame_number=oldest_page;
PageTable[request].valid=true;
page_fault_counter++;
memory_ref_counter++;
}
if (request_counter==1000){
FILE *fp=fopen("data.txt", "a");
fprintf (fp, "%d\n", memory_ref_counter);
memory_ref_counter=0;
request_counter=0;
fclose(fp);
}
}
printf("Total page faults %d\n", page_fault_counter);
fclose(fp);
free(PageTable);
free(FrameTable);
return 0;
}
void AgeIncrease (int frames){
int i;
for (i=0;i<frames;i++){
FrameTable[i].age++;
}
}
int OldestIndex (int frames){
int oldest=-1;
int i;
int index;
for (i=0;i<frames;i++){
if (oldest<FrameTable[i].age){
oldest=FrameTable[i].age;
index=i;
}
}
return index;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 9 images

- can you please double-check this code? thank you!arrow_forwardBoth the utmp file and any directory are organized as a sequence of structs. Consider the two structs definitioned here: struct { char a; int b; char c; } one; struct { char a; char c; int b; } two; Which of the following is true? If you don't know the answer (we did discuss this in class), experiment in order to find the answer. struct one takes up more memory than struct two. struct two takes up more memory than struct one. They both take up the same amout of memory.arrow_forwardNeed another function written in python from the csv linkarrow_forward
- Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Hints: Use the find() function to find the index of a comma in each row of…arrow_forwardConsider the following algorithm to generate a sequence of numbers: Start with a random integer value n, which lies between 100 and 1000. If n is even divide it by 2, if n is odd, multiply it by 3 and add 1 to it. Repeat this process with the new value of n, terminating when n = 1. Store the sequence of numbers that you get in a mat file.arrow_forwardSketch the graph of the function h given below. Take a picture of your work, and then upload it into the problem using the button below. h:R→Z, h(n) = [3n-1]. Attach File Browse Local Files Browse Content Collection Browse Dropboxarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
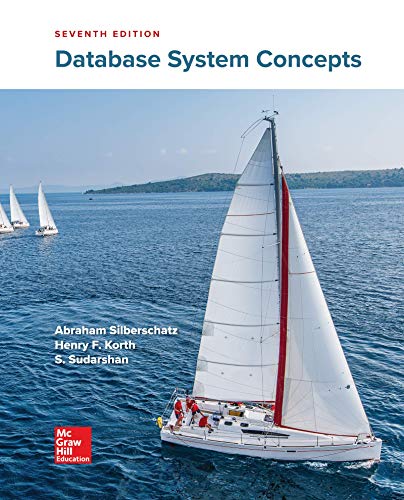
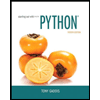
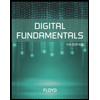
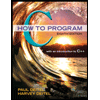
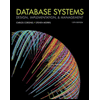
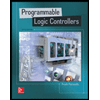