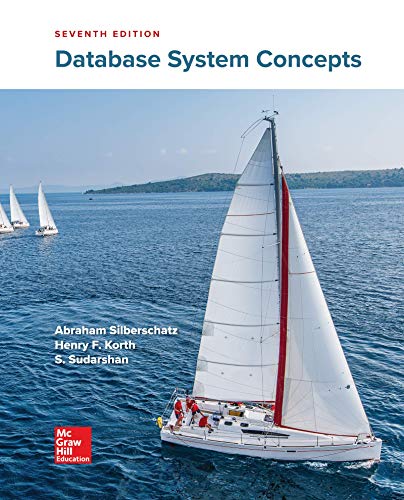
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Compute the distance between the positions of the first and last zero element in the given array. For example if the array is 3 0 1 0 4 9 5 0 6 your algorithm should yield 7 - 1 = 6. If the array contains a single zero return 0. If it doesn’t contain any zeros return a negative value
Only edit code within the /*Your code goes here*/ portion.
![### Java Code Snippet for Educational Purposes
#### Overview
This image displays a Java integrated development environment (IDE) with a code snippet from a file named `Numbers.java`. The code defines a public class `Numbers` with a static method `zeroesDistance`.
#### Code Explanation
```java
public class Numbers {
public static int zeroesDistance(double[] values) {
int firstPos = 0;
int lastPos = values.length - 1;
boolean found = false;
while (firstPos < values.length && !found) {
/* Your code goes here */
}
return lastPos - firstPos;
}
}
```
- **Class Declaration**: `Numbers` – This is the name of the class.
- **Method Declaration**: `zeroesDistance` – A static method that accepts an array of doubles and returns an integer.
- **Variables**:
- `firstPos`: Initialized to 0, likely used to track the initial position.
- `lastPos`: Initialized to the last index of the `values` array.
- `found`: A boolean set to `false`, potentially for controlling the loop.
- **While Loop**: Contains a placeholder for the code, indicated by the comment `/* Your code goes here */`. This loop appears to iterate through the `values` array until a condition is met.
- **Return Statement**: Returns the difference between `lastPos` and `firstPos`.
#### Projects and Files
The left pane shows a file explorer with several Java files listed, indicating additional projects that might relate to different coding exercises or demonstrations.
#### Console Output
At the bottom, there's a console output showing interaction with a program, likely related to the code:
- Input Series: "Enter scores, -1 to quit"
- Output Series: The scores entered are displayed, indicating a user input and echoing sequence.
- **Process Completion**: The process finished successfully with exit code 0.
This educational snippet can be used to explain basic Java methods, loops, and handling arrays within a Java class.](https://content.bartleby.com/qna-images/question/d6e87594-a0d7-48c0-af39-cad58e8108aa/004af3bf-d3a0-41cd-b232-ac8bd1e11824/yaouxbb_thumbnail.jpeg)
Transcribed Image Text:### Java Code Snippet for Educational Purposes
#### Overview
This image displays a Java integrated development environment (IDE) with a code snippet from a file named `Numbers.java`. The code defines a public class `Numbers` with a static method `zeroesDistance`.
#### Code Explanation
```java
public class Numbers {
public static int zeroesDistance(double[] values) {
int firstPos = 0;
int lastPos = values.length - 1;
boolean found = false;
while (firstPos < values.length && !found) {
/* Your code goes here */
}
return lastPos - firstPos;
}
}
```
- **Class Declaration**: `Numbers` – This is the name of the class.
- **Method Declaration**: `zeroesDistance` – A static method that accepts an array of doubles and returns an integer.
- **Variables**:
- `firstPos`: Initialized to 0, likely used to track the initial position.
- `lastPos`: Initialized to the last index of the `values` array.
- `found`: A boolean set to `false`, potentially for controlling the loop.
- **While Loop**: Contains a placeholder for the code, indicated by the comment `/* Your code goes here */`. This loop appears to iterate through the `values` array until a condition is met.
- **Return Statement**: Returns the difference between `lastPos` and `firstPos`.
#### Projects and Files
The left pane shows a file explorer with several Java files listed, indicating additional projects that might relate to different coding exercises or demonstrations.
#### Console Output
At the bottom, there's a console output showing interaction with a program, likely related to the code:
- Input Series: "Enter scores, -1 to quit"
- Output Series: The scores entered are displayed, indicating a user input and echoing sequence.
- **Process Completion**: The process finished successfully with exit code 0.
This educational snippet can be used to explain basic Java methods, loops, and handling arrays within a Java class.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Use for loops on the above 2D array to: •Find how many ZEROsand how many negative numbersare in the matrixand display results*HELP: declare a variable “count_zero= 0and count_neg=0” and increment the count each time you findzero and negativearrow_forwardThis program will create an array of 12 random integers (between 1-9) and remove all numbers less than or equal to another randomly generated integer (between 1-9)There are four steps to accomplish this.a. Create and fill an array of random integers between 1-9. The array must be size 12.b. Generate a random integer between 1-9.c. Write the code that will create a new array of the integers in the given array but with the numbers less than or equal to your random number removed. For example, if you randomly generated 6 and the original array contains 1 8 9 4 4 7 2 2 5 8 4 7 your new array will contain 8 9 7 8 7d. Print the original array, the number generated, and the new array.i.e. The original array contained:1 8 9 4 4 7 2 2 5 8 4 7All numbers less than or equal to 4 to be removed.The new array contains:8 9 7 5 8 7*Keep in mind that because we are dealing with random numbers, it is possible that the random number you generate for numbers to be removed from the array is less than…arrow_forwardThe array size declarator must be an integer expression with a value greater than or equal to zero. True Falsearrow_forward
- Finding the highest & lowest values in an array. Given the following information: Declare Integer arraySize = 10 Declare Real numberArray(arraySize = 8.54, 90.41, 8.54, 100, 3, 100.02, 2.99, 44, 100.1, 4.47 Create variables to hold the highest and lowest value, initially set them the value at the first element in the array. Don't hard code the values, use the array and the subscript. Use a loop to step through the rest of the elements At each iteration, a comparison is made to the highest and lowest variable If the element is greater than the highest value, that value is then the assigned to the highest variable. If the element is lower than the lowest value, that element is assigned to the lowest variable. Display the highest and lowest numbers found at the end of the program. If you plan to use Raptor, great, but remember two things. First, Raptor starts their arrays at 1 not 0. Second, there is no for-loop, so you must use a do until loop with an index counter. I have provided a…arrow_forwardproduce a trace table /Declare a constant for the array size. Constant Integer SIZE = 5 3 / Declare an array initialized with values. 5 Declare Integer numbers[SIZE] = 2, 4, 6, 8, 10 / Declare and initialize an accumulator variable. 8. Declare Integer total = 0 10 // Declare a counter variable for the loop. 11 Declare Integer index 12 13 // Calculate the total of the array elements. 14 For index = 0 To SIZE 15 Set total = total + numbers [index] 16 End For 17 18 /Display the sum of the array elements. 19 Display "The sum of the array elements is totalarrow_forwardCreate an application that compares the four advanced sorting algorithms discussed in this chapter. For the tests, create a 1,000-element randomly generated array. What is the algorithm's position? What happens when you expand the array size to 10,000, then 100,000 elements?arrow_forward
- Construct a row array countValues from 0 to 25, elements incremented by incrementValue. Ex: If incrementValue is 5, countValues should be [0, 5, 10, 15, 20, 25].arrow_forwardThe Fibonacci numbers are 0, 1, 1, 2, 3, 5, 8, 13, ... where each number (after the first two values) is the sum of the previous two numbers. Write a program that declares an integer array called fib_numbers with a length of 25 elements. The program then fills the array with the first 25 Fibonacci numbers using a loop. The program then prints the values to the screen using a separate loop.arrow_forwardCreate an array called sales to monitor one week's worth of sales over the course of two months. Assign sales data to the array's elements. Then, in a loop, compute the average sales for the one week of each of the two months that are recorded in the array.arrow_forward
- TicTacToeCreate the board display the board Set token to ‘x’Loop //at most 9 times, but end loop when someone wins{ Ask player where to place token display the board Checks if won Change token (if it was ‘x’ change to ‘o’ and vice versa)}Either congratulate someone on winning or say it was a draw. Create a 17x17 array of characters and put ‘ ‘ in each element.2. Write a loop to make the first horizontal line, and another to do the second horizontal line3. Write a loop to make the first vertical line, and another to create the second vertical line4. Put in the numbers (this is so the player can choose where to put the token). You will need 9 separate assignment statements.arrow_forwardArray a is an integer array with all the elements in ascending order. The element at position 15 is the 15th largest and also the 15th smallest. You can assume integers are 4 bytes long. How many bytes are there in the array? O 116 124 O None of the other answers is correct. 120arrow_forwardIn Python, Create a 2 ×4 Numpy array filled with zeros. Using a nested for loop enter int grade valuesto all elements of the array (assume the first row is Fall Semester and the second row is SpringSemester).arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
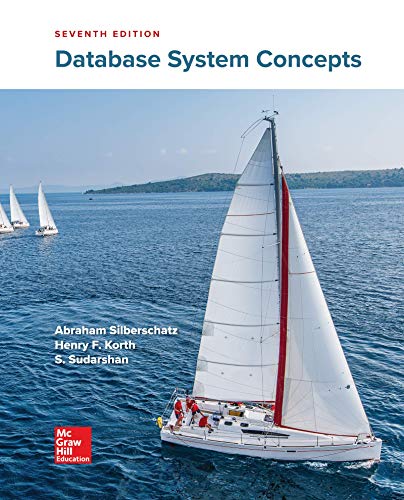
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
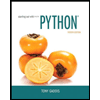
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
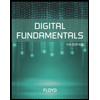
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
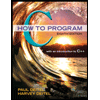
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
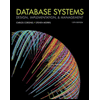
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
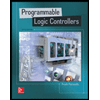
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education