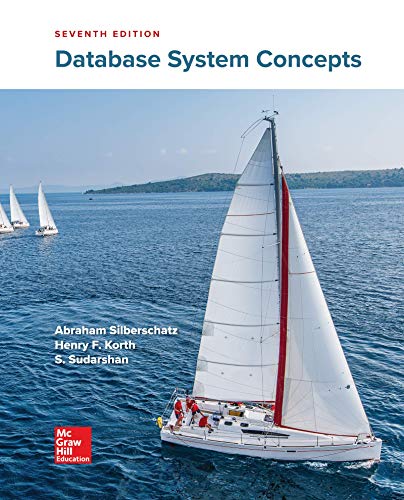
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Topic Video
Question
please fill 1 to 5 and lable it which one is map move count and all
![### Problem 17: Unsigned Integer Radix Sort
**Objective:** Write a C program for sorting 32-bit unsigned integers using radix sort with a group of 4 bits. Use the variables listed below. Assume `lst` is initialized with n numbers.
```c
#define N 1048576
#define BIN 256
#define MAXBIT 32
#define LST 1
#define BUF 0
int n, group, bin;
int flag; /* to show which one holds numbers: lst or buf */
int lst[N], buf[N];
int count[BIN], map[BIN], tmap[BIN];
int main(int argc, char **argv) {
int i;
flag = LST;
initialize(); /* initialize lst with n random floats */
for (i = 0; i < MAXBIT; i += group) radix_sort(i); /* move lst to buf or buf to lst depending on the iteration number */
correct(); /* sorted numbers must be in lst */
}
void radix_sort(int idx) {
int j, k, mask; /* initialize mask for lifting the 4 least significant bits. */
int *src_p, *dst_p; /* cast lst and buf to int pointers to treat lst/buf as int's */
/* set src_p and dst_p */
_____1_____
/* count */
_____2_____
/* map */
_____3_____
/* move */
_____4_____
}
void correct() {
_____5_____
}
```
### Explanation
1. **Definitions and Initialization:**
- `#define N 1048576`: Number of elements in the list.
- `#define BIN 256`: Number of bins used for sorting.
- `#define MAXBIT 32`: Maximum number of bits (for 32-bit integers).
- `#define LST 1`, `#define BUF 0`: Flags to indicate which array (lst or buf) currently holds the numbers.
- Variables `n`, `group`, and `bin` manage sorting and grouping operations.
- `lst` and `buf` are the arrays holding the numbers to be sorted.
- `count`, `map`, and `tmap` are auxiliary arrays for counting and mapping during the sort.
2. **Main Function:**
- Initializes the sorting process.
-](https://content.bartleby.com/qna-images/question/5bad4e48-dad8-4710-a64b-b24b80d1efcf/7576cf54-f267-45b0-9883-7d0fce3ad3a7/j59l4uq_thumbnail.png)
Transcribed Image Text:### Problem 17: Unsigned Integer Radix Sort
**Objective:** Write a C program for sorting 32-bit unsigned integers using radix sort with a group of 4 bits. Use the variables listed below. Assume `lst` is initialized with n numbers.
```c
#define N 1048576
#define BIN 256
#define MAXBIT 32
#define LST 1
#define BUF 0
int n, group, bin;
int flag; /* to show which one holds numbers: lst or buf */
int lst[N], buf[N];
int count[BIN], map[BIN], tmap[BIN];
int main(int argc, char **argv) {
int i;
flag = LST;
initialize(); /* initialize lst with n random floats */
for (i = 0; i < MAXBIT; i += group) radix_sort(i); /* move lst to buf or buf to lst depending on the iteration number */
correct(); /* sorted numbers must be in lst */
}
void radix_sort(int idx) {
int j, k, mask; /* initialize mask for lifting the 4 least significant bits. */
int *src_p, *dst_p; /* cast lst and buf to int pointers to treat lst/buf as int's */
/* set src_p and dst_p */
_____1_____
/* count */
_____2_____
/* map */
_____3_____
/* move */
_____4_____
}
void correct() {
_____5_____
}
```
### Explanation
1. **Definitions and Initialization:**
- `#define N 1048576`: Number of elements in the list.
- `#define BIN 256`: Number of bins used for sorting.
- `#define MAXBIT 32`: Maximum number of bits (for 32-bit integers).
- `#define LST 1`, `#define BUF 0`: Flags to indicate which array (lst or buf) currently holds the numbers.
- Variables `n`, `group`, and `bin` manage sorting and grouping operations.
- `lst` and `buf` are the arrays holding the numbers to be sorted.
- `count`, `map`, and `tmap` are auxiliary arrays for counting and mapping during the sort.
2. **Main Function:**
- Initializes the sorting process.
-
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a for loop to print all elements in courseGrades, following each element with a space (including the last). Print forwards, then backwards. End each loop with a newline. Ex: If courseGrades = {7, 9, 11, 10}, print:7 9 11 10 10 11 9 7 Hint: Use two for loops. Second loop starts with i = NUM_VALS - 1. Note: These activities may test code with different test values. This activity will perform two tests, both with a 4-element array (int courseGrades[4]). Also note: If the submitted code tries to access an invalid array element, such as courseGrades[9] for a 4-element array, the test may generate strange results. Or the test may crash and report "Program end never reached", in which case the system doesn't print the test case that caused the reported message. #include <iostream>using namespace std; int main() { const int NUM_VALS = 4; int courseGrades[NUM_VALS]; int i; for (i = 0; i < NUM_VALS; ++i) { cin >> courseGrades[i]; } /* Your solution goes…arrow_forwardFIX THIS CODE Using python Application CODE: import csv playersList = [] with open('Players.csv') as f: rows = csv.DictReader(f) for r in rows: playersList.append(r) teamsList = [] with open('Teams.csv') as f: rows = csv.DictReader(f) for r in rows: teamsList.append(r) plays=len(playersList) for i in range(plays): if playersList[i]['team']=='Argentina' and int(playerlist[i]['minutes played'])<200 and int(playersList[i] ['shots'])>20: print(playersList[i]['last name']) c0=0 c1=0 c2=0 for i in range(len(teamsList)): if int(teamsList[i]['redCards'])==0: c0=c0+1 if int(teamsList[i]['redCards'])==1: c1=c1+1 if int(teamsList[i]['redCards'])==2: c2=c2+1 print("Number of teams with zero redcards:",c0) print("Number of teams with zero redcards:",c1) print("Number of teams with zero redcards:",c2) ratio=0 for i in range(len(teamsList)): if int(teamsList[i]['games']>3) and if int(teamsList[i]['goalsFor'])/int(teamsList[i]['goalsAgainst'])<ratio:…arrow_forwardConsider the set A = {1, 2, 11, {121}, 22, 1212, (111, 212, 112}} Enter the value of Al. (Note: Enter a number).arrow_forward
- text file 80 1 2 3 100 100 100 1001 0 2 100 3 4 100 1002 2 0 4 4 100 5 1003 100 4 0 100 100 4 100100 3 4 100 0 3 3 3100 4 100 100 3 0 100 1100 100 5 4 3 100 0 2100 100 100 100 3 1 2 0 My code below. I am getting an error when trying to create my adjacency matrix. i dont know what i am doing wrong def readMatrix(inputfilename): ''' Returns a two-dimentional array created from the data in the given file. Pre: 'inputfilename' is the name of a text file whose first row contains the number of vertices in a graph and whose subsequent rows contain the rows of the adjacency matrix of the graph. ''' # Open the file f = open(inputfilename, 'r') # Read the number of vertices from the first line of the file n = int(f.readline().strip()) # Read the rest of the file stripping off the newline characters and splitting it into # a list of intger values rest = f.read().strip().split() # Create the adjacency matrix adjMat = []…arrow_forwardWhat does the following error message normally mean? IndexError: list index out of range Select one: а. This error normally indicates that you have tried to access an element of a list that doesn't exist. O b. This error normally means that you have used the range() function incorrectly. С. This error normally means that you have used a list where you should have used a tuple. d. This error normally means that you used too many lines of code in your program.arrow_forwardYour favorite soccer player is playing in the world cup and the match has ended in a tie. As the teams get ready for the penalty shootout, you know your favorite player will be last to shoot. A quick search tells you that your favorite player makes a goal when kicking from the penalty mark 0.76% of the time. You also know that the player before your favorite player makes a kick from the penalty mark 0.70% of the time. You also know that neither player is impacted by kicks before they take the shot. What is the probability that both players miss their kicks? Report your answer to the second decimal point, that is, 0.45 instead of 0.5.arrow_forward
- The function count_contains_og in python takes a list of strings and returns how many strings in the list contain 'og' / 'OG' / 'oG' / 'Og' (check for 'og', ignoring case). Hint: Use the sequence membership operator in to help you check for 'og' in the individual strings. Create a lower-cased version of the string (lower), then use the in operator. For example: Test Result str_list = ['cat', 'dog', 'FROG', 'monkey'] print(count_contains_og(str_list)) 2 strlist = ["X", "x"] print(count_contains_og(strlist)) 0 list_of_one_og = ["Doggie"] print(count_contains_og(list_of_one_og)) 1arrow_forwardAs part of this assignment, the program that you will be writing will store current grades in a dictionary using course codes as keys and with values consisting of percent grades in lists. The main functions of this program are to print a student's gradebook, to drop the lowest grade in each course, print the student's gradebook again, drop the course with lowest average, and finally printing the student's gradebook again. This program requires a main function and a custom value-returning function. In the main function, code these basic steps in this sequence (intermediate steps may be missing): start with an empty dictionary that represents a gradebook and then use a while loop to allow the input of course codes from the keyboard. End the while loop when the user presses enter without entering data.within the while loop:for each course entered, use a list comprehension to generate five random integers in the range of 70 through 100. These random integers in a list represent the…arrow_forwardThis solution does not work for the question asked. The question submitted clearly shows 2 list boxes on form 1. Is there an updated code?arrow_forward
- In Kotlin, 4.Create a list of Ints from 1 to 25 Use map() with the list and your area function to create a list of the areas of circles with each of the Ints as radiusarrow_forwardComplete the method “readdata”. In this method you are to read in at least 10 sets of student data into a linked list. The data to read in is: student id number, name, major (CIS or Math) and student GPA. You will enter data of your choice. You will need a loop to continue entering data until the user wishes to stop. Complete the method “printdata”. In this method, you are to print all of the data that was entered into the linked list in the method readdata. Complete the method “printstats”. In this method, you are to search the linked list and print the following: List of student’s id and names who are CIS majors List of student’s id and names who are Math majors List of student’s names along with their gpa who are honor students (gpa 3.5 or greater) All information for the CIS student with the highest gpa (you may assume that different gpa values have been entered for all students) CODE (student_list.java) You MUST use this code: package student_list;import…arrow_forwardOverview This is a review assignment where you will use multiple tools you have picked up so far throughout the course. If you're confused about how to do something make sure you look back at earlier assignments. In this program you will start by declaring an empty dictionary named population_dict. Then you will use a while-loop to prompt a user for a series of cities and their populations, storing the input in population_dict). After this you will prompt the user to decide what is the maximum population for a city to be considers a small down. Finally, you will open a file called populationdata.txt where you will write the results. Expected Output Example of standard out (the console): What is next city? (enter q to quit) Long Beach What is the population of Long Beach? 362000 What is next city? (enter q to quit) Los Angeles What is the population of Los Angeles? 382000000 What is next city? (enter q to quit) San Diego What is the population of San Diego? 136000000 What is next city?…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
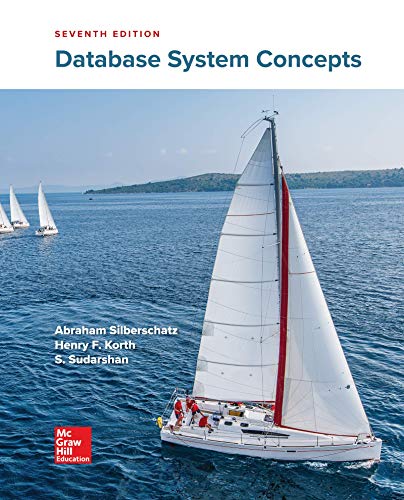
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
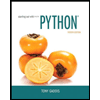
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
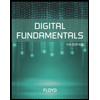
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
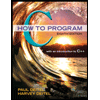
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
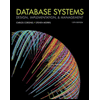
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
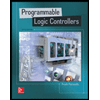
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education