Problem 3 Write a function buckets ('a -> 'a -> bool) -> 'a list -> 'a list list. that partitions a list into equivalence classes. That is, buckets equiv 1st should return a list of lists where each sublist in the result contains equivalent elements, where two elements are considered equivalent if equiv returns true. For example: buckets () [1;2;3;4] = [[1]; [2] ; [3]; [4]] buckets (=) [1; 2; 3; 4; 2; 3; 4;3;4] = buckets (fun x y-> (=) (x mod 3) [[1]; [2; 2]; [3; 3; 3]; [4; 4;4]] (y mod 3)) [1; 2; 3; 4; 5; 6] = [[1;4]; [2;5]; [3; 6]] The order of the buckets must reflect the order in which the elements appear in the original list. For example, the output of buckets (=) [1;2;3;4] should be [[1]; [2] ; [3]; [4]] and not [[2] ; [1]; [3]; [4]] or any other permutation. The order of the elements in each bucket must reflect the order in which the elements appear in the original list. For example, the output of buckets (fun x y-> (=) (x mod 3) (y mod 3)) [1; 2; 3; 4; 5; 6] should be [[1;4]; [2;5]; [3;6]] and not [[4;1]; [5;2]; [3; 6]] or any other
Problem 3 Write a function buckets ('a -> 'a -> bool) -> 'a list -> 'a list list. that partitions a list into equivalence classes. That is, buckets equiv 1st should return a list of lists where each sublist in the result contains equivalent elements, where two elements are considered equivalent if equiv returns true. For example: buckets () [1;2;3;4] = [[1]; [2] ; [3]; [4]] buckets (=) [1; 2; 3; 4; 2; 3; 4;3;4] = buckets (fun x y-> (=) (x mod 3) [[1]; [2; 2]; [3; 3; 3]; [4; 4;4]] (y mod 3)) [1; 2; 3; 4; 5; 6] = [[1;4]; [2;5]; [3; 6]] The order of the buckets must reflect the order in which the elements appear in the original list. For example, the output of buckets (=) [1;2;3;4] should be [[1]; [2] ; [3]; [4]] and not [[2] ; [1]; [3]; [4]] or any other permutation. The order of the elements in each bucket must reflect the order in which the elements appear in the original list. For example, the output of buckets (fun x y-> (=) (x mod 3) (y mod 3)) [1; 2; 3; 4; 5; 6] should be [[1;4]; [2;5]; [3;6]] and not [[4;1]; [5;2]; [3; 6]] or any other
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 18SA
Related questions
Question
OCAML Code please, thank you!
![Problem 3
In [ ]:
Write a function
buckets : ('a -> 'a -> bool) -> 'a list -> 'a list list
that partitions a list into equivalence classes. That is, buckets equiv 1st should return a list of lists where each sublist in the result contains equivalent
elements, where two elements are considered equivalent if equiv returns true. For example:
buckets (=) [1; 2; 3; 4] = [[1]; [2] ; [3]; [4]]
buckets (=) [1; 2; 3; 4; 2; 3; 4; 3; 4]
buckets (fun x y -> (=) (x mod 3)
[[1;4]; [2;5]; [3; 6]]
The order of the buckets must reflect the order in which the elements appear in the original list. For example, the output of buckets (=) [1;2;3;4]
should be [[1] ; [2] ; [3] ; [4]] and not [[2]; [¹]; [3]; [4]] or any other permutation.
=
The order of the elements in each bucket must reflect the order in which the elements appear in the original list. For example, the output of buckets (fun x
y -> (=) (x mod 3) (y mod 3)) [1; 2; 3; 4; 5; 6] should be [[1;4]; [2;5]; [3;6]] and not [[4;1]; [5;2]; [3;6]] or any other
permutations.
Just use lists. Do not use sets or hash tables.
[[1]; [2; 2]; [3; 3; 3]; [4; 4;4]]
(y mod 3)) [1; 2; 3; 4; 5; 6]
Assume that the comparison function ('a -> 'a -> bool) is commutative, associative and idempotent.
In [] let buckets p 1 =
List append function @ may come in handy. [1;2;3] @ [4;5;6]
(* YOUR CODE HERE *)
assert (buckets (=) [1; 2; 3; 4] [[¹]; [2] ; [3]; [4]]);
assert (buckets (=) [1; 2; 3; 4; 2; 3; 4; 3; 4]
assert (buckets (fun x y -> (=) (x mod 3)
=
=
[1; 2; 3; 4; 5; 6].
[[1]; [2; 2]; [3; 3; 3]; [4; 4; 4]]);
(y mod 3)) [1; 2; 3; 4; 5; 6]
=
[[1;4]; [2;5]; [3; 6]])](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F95a918cc-648a-4ba1-8333-a4ee16085cbc%2F39c678fc-ef2d-47d5-9a63-f8356e399080%2Frot42jt_processed.png&w=3840&q=75)
Transcribed Image Text:Problem 3
In [ ]:
Write a function
buckets : ('a -> 'a -> bool) -> 'a list -> 'a list list
that partitions a list into equivalence classes. That is, buckets equiv 1st should return a list of lists where each sublist in the result contains equivalent
elements, where two elements are considered equivalent if equiv returns true. For example:
buckets (=) [1; 2; 3; 4] = [[1]; [2] ; [3]; [4]]
buckets (=) [1; 2; 3; 4; 2; 3; 4; 3; 4]
buckets (fun x y -> (=) (x mod 3)
[[1;4]; [2;5]; [3; 6]]
The order of the buckets must reflect the order in which the elements appear in the original list. For example, the output of buckets (=) [1;2;3;4]
should be [[1] ; [2] ; [3] ; [4]] and not [[2]; [¹]; [3]; [4]] or any other permutation.
=
The order of the elements in each bucket must reflect the order in which the elements appear in the original list. For example, the output of buckets (fun x
y -> (=) (x mod 3) (y mod 3)) [1; 2; 3; 4; 5; 6] should be [[1;4]; [2;5]; [3;6]] and not [[4;1]; [5;2]; [3;6]] or any other
permutations.
Just use lists. Do not use sets or hash tables.
[[1]; [2; 2]; [3; 3; 3]; [4; 4;4]]
(y mod 3)) [1; 2; 3; 4; 5; 6]
Assume that the comparison function ('a -> 'a -> bool) is commutative, associative and idempotent.
In [] let buckets p 1 =
List append function @ may come in handy. [1;2;3] @ [4;5;6]
(* YOUR CODE HERE *)
assert (buckets (=) [1; 2; 3; 4] [[¹]; [2] ; [3]; [4]]);
assert (buckets (=) [1; 2; 3; 4; 2; 3; 4; 3; 4]
assert (buckets (fun x y -> (=) (x mod 3)
=
=
[1; 2; 3; 4; 5; 6].
[[1]; [2; 2]; [3; 3; 3]; [4; 4; 4]]);
(y mod 3)) [1; 2; 3; 4; 5; 6]
=
[[1;4]; [2;5]; [3; 6]])
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
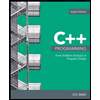
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
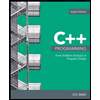
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning