Question
C++ questions
why I'm getting these errors please help
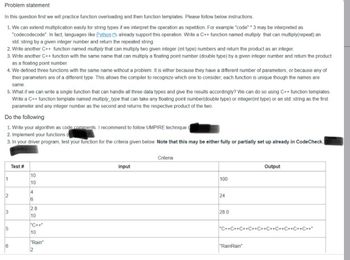
Transcribed Image Text:### Problem Statement
In this question, we will first practice function overloading and then function templates. Please follow the instructions below.
1. **String Multiplication**:
- We can extend multiplication easily for string types if we interpret the operation as repetition. For example, the expression "code" * 3 may be interpreted as "codecodecode". In fact, languages like Python already support this operation.
- **Task**: Write a C++ function named `multiply` that can multiply (repeat) an `std::string` by a given integer number and return the repeated string.
2. **Integer Multiplication**:
- Write another C++ function named `multiply` that can multiply two integer (int type) numbers and return the product as an integer.
3. **Floating-Point Multiplication**:
- Write another C++ function with the same name that can multiply a floating-point number (double type) by a given integer number and return the product as a floating-point number.
4. **Function Uniqueness**:
- We defined three functions with the same name without a problem. It is either because they have a different number of parameters, or because any of their parameters are of a different type. This allows the compiler to recognize which one to consider; each function is unique though the names are the same.
5. **Function Templates**:
- What if we want to write a single function that can handle all three data types and give the results accordingly? We can do so using C++ function templates.
- **Task**: Write a C++ function template named `multiply_type` that can take any floating point number (double type) or integer (int type) or an `std::string` as the first parameter and any integer number as the second and return the respective product of the two.
### Do the Following
1. **Algorithm Comments**:
- Write your algorithm as code comments. It is recommended to follow the UMPIRE technique.
2. **Function Implementation**:
- Implement your functions.
3. **Testing**:
- In your driver program, test your function for the criteria given below.
- **Note**: This may be either fully or partially set up already in CodeCheck (20 points).
### Test Criteria
<table>
<tr>
<th>Test #</th>
<th>Input</th>
<th>Output</
![## Introduction to Multiplication Functions in C++
In this lesson, we will explore the implementation and testing of a function that multiplies values of different types in C++. We will cover function declarations, definitions, and the use of templates for generic programming.
### Function Implementation (`multiply.cpp`)
This section contains the implementation of various multiplication functions:
```cpp
// IMPLEMENT
#include "multiply.h"
std::string multiply(std::string string1, int number) {
T return_value = T();
for (int i = 0; i < number; ++i) {
return_value += string1;
}
return return_value;
}
int multiply(int number1, int number2) {
return number1 * number2;
}
double multiply(double number1, int number2) {
return number1 * number2;
}
```
### Header File (`multiply.h`)
This header file declares the multiplication functions and provides a template for a more flexible multiply function:
```cpp
// IMPLEMENT
#ifndef MULTIPLY_H
#define MULTIPLY_H
#include <string>
std::string multiply(std::string string1, int number);
int multiply(int number1, int number2);
double multiply(double number1, int number2);
// In templates, we can't separate the declaration from the definition; We have to write it all together
template<class T>
T multiply_type(T entity, int number) {
// Write your code below
// You may need to initialize your return value like
// T return_value = T();
T return_value = T();
for (int i = 0; i < number; ++i) {
return_value += entity;
}
return return_value;
}
#endif // MULTIPLY_H
```
### Driver File (`multiply_driver.cpp`)
The driver file tests the multiplication functions:
```cpp
// REVIEW
#include <iostream>
#include "multiply.h"
// Replace ... with your code. Hit enter key to get more vertical space
int main(int argc, char *argv[]) {
// Testing multiply
std::cout << multiply(10, 10) << std::endl;
// By exactly following the pattern above, test your function below for the rest of the tests in the order they are given
std::cout << multiply(4, 6) << std::endl;
std::cout << multiply(2.8, 10) <<](https://content.bartleby.com/qna-images/question/69991f76-d6e5-4f32-9883-cbbc5a2c7e26/32dc813b-b170-493f-81e3-eb681ca9cc73/r3avs1y_thumbnail.jpeg)
Transcribed Image Text:## Introduction to Multiplication Functions in C++
In this lesson, we will explore the implementation and testing of a function that multiplies values of different types in C++. We will cover function declarations, definitions, and the use of templates for generic programming.
### Function Implementation (`multiply.cpp`)
This section contains the implementation of various multiplication functions:
```cpp
// IMPLEMENT
#include "multiply.h"
std::string multiply(std::string string1, int number) {
T return_value = T();
for (int i = 0; i < number; ++i) {
return_value += string1;
}
return return_value;
}
int multiply(int number1, int number2) {
return number1 * number2;
}
double multiply(double number1, int number2) {
return number1 * number2;
}
```
### Header File (`multiply.h`)
This header file declares the multiplication functions and provides a template for a more flexible multiply function:
```cpp
// IMPLEMENT
#ifndef MULTIPLY_H
#define MULTIPLY_H
#include <string>
std::string multiply(std::string string1, int number);
int multiply(int number1, int number2);
double multiply(double number1, int number2);
// In templates, we can't separate the declaration from the definition; We have to write it all together
template<class T>
T multiply_type(T entity, int number) {
// Write your code below
// You may need to initialize your return value like
// T return_value = T();
T return_value = T();
for (int i = 0; i < number; ++i) {
return_value += entity;
}
return return_value;
}
#endif // MULTIPLY_H
```
### Driver File (`multiply_driver.cpp`)
The driver file tests the multiplication functions:
```cpp
// REVIEW
#include <iostream>
#include "multiply.h"
// Replace ... with your code. Hit enter key to get more vertical space
int main(int argc, char *argv[]) {
// Testing multiply
std::cout << multiply(10, 10) << std::endl;
// By exactly following the pattern above, test your function below for the rest of the tests in the order they are given
std::cout << multiply(4, 6) << std::endl;
std::cout << multiply(2.8, 10) <<
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 4 images

Knowledge Booster
Similar questions
- c++ Language I need help with question #6 part c please. **WITHOUT "DP" please. I keep getting dp in the answers from you guys and it's very confusing and i can't use that please. Don't use "DP" please and thank you so much. I asked this question 3 times but i keep getting programs im not able to run. Please make it as simple as possible for me thank you so so much. I did a and b already, i just need with c using dynamic programming. Please and thanks!arrow_forwardThis is warning you, don't use any AI platform to generate answer.arrow_forwardPlease due in C++ If possible can explain what should I put in StudentInfo.h file what in StudentInfo.c Thank youarrow_forward
- C++ ONLY PLEASE, and please read carefully, i have had people submit wrong answers Assignment 7 A: Rare Collection. We can make arrays of custom objects just like we’ve done with ints and strings. While it’s possible to make both 1D and 2D arrays of objects (and more), for this assignment we’ll start you out with just one dimensional arrays. Your parents have asked you to develop a program to help them organize the collection of rare CDs they currently have sitting in their car’s glove box. To do this, you will first create an AudioCD class. It should have the following private attributes. String cdTitle String[4] artists int releaseYear String genre float condition Your class should also have the following methods: Default Constructor: Initializes the five attributes to the following default values: ◦ cdTitle = “” ◦ artists = {“”, “”, “”, “”} ◦ releaseYear = 1980 ◦ genre = “” ◦ condition = 0.0 Overloaded Constructor: Initializes the five attributes based on values passed…arrow_forwardCode in c++ pleasearrow_forwardCan I please get help with this program?arrow_forward
arrow_back_ios
arrow_forward_ios