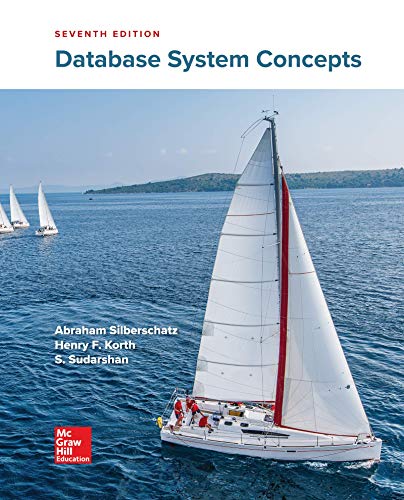
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
screen shoot shows the text arrayboundedqueue and arrayunboundedqueue
which approach does the text's use to creating an array-based queue implementation:
A. the same as its approach to the array-based stack.
B. the fixed-front approach.
C. the floating-front approach.
![```java
public class ArrayBoundedQueue<T> implements QueueInterface<T>
{
protected final int DEFCAP = 100; // default capacity
protected T[] elements; // array that holds queue elements
protected int numElements = 0; // number of elements in this queue
protected int front = 0; // index of front of queue
protected int rear; // index of rear of queue
public ArrayBoundedQueue()
{
elements = (T[]) new Object[DEFCAP];
rear = DEFCAP - 1;
}
public ArrayBoundedQueue(int maxSize)
{
elements = (T[]) new Object[maxSize];
rear = maxSize - 1;
}
public void enqueue(T element)
// Throws QueueOverflowException if this queue is full;
// otherwise, adds element to the rear of this queue.
{
if (isFull())
throw new QueueOverflowException("Enqueue attempted on a full queue.");
else
{
rear = (rear + 1) % elements.length;
elements[rear] = element;
numElements = numElements + 1;
}
}
public T dequeue()
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it.
{
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue.");
else
{
T toReturn = elements[front];
elements[front] = null;
front = (front + 1) % elements.length;
numElements = numElements - 1;
return toReturn;
}
}
public boolean isEmpty()
// Returns true if this queue is empty; otherwise, returns false.
{
return (numElements == 0);
}
public boolean isFull()
// Returns true if this queue is full; otherwise, returns false.
{
return (numElements == elements.length);
}
public int size()
// Returns the number of elements in this queue.
{
return numElements;
}
}
```
**Explanation of Key Code Sections:**
- **ArrayBoundedQueue Class:**
- This class implements a generic bounded queue using an array.
- `DEFCAP` is a](https://content.bartleby.com/qna-images/question/1b2c1e36-9974-49eb-8a0c-fe4d3c635f06/284c88fa-e731-488c-99c5-a84ea198b183/1bnapoe_thumbnail.png)
Transcribed Image Text:```java
public class ArrayBoundedQueue<T> implements QueueInterface<T>
{
protected final int DEFCAP = 100; // default capacity
protected T[] elements; // array that holds queue elements
protected int numElements = 0; // number of elements in this queue
protected int front = 0; // index of front of queue
protected int rear; // index of rear of queue
public ArrayBoundedQueue()
{
elements = (T[]) new Object[DEFCAP];
rear = DEFCAP - 1;
}
public ArrayBoundedQueue(int maxSize)
{
elements = (T[]) new Object[maxSize];
rear = maxSize - 1;
}
public void enqueue(T element)
// Throws QueueOverflowException if this queue is full;
// otherwise, adds element to the rear of this queue.
{
if (isFull())
throw new QueueOverflowException("Enqueue attempted on a full queue.");
else
{
rear = (rear + 1) % elements.length;
elements[rear] = element;
numElements = numElements + 1;
}
}
public T dequeue()
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it.
{
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue.");
else
{
T toReturn = elements[front];
elements[front] = null;
front = (front + 1) % elements.length;
numElements = numElements - 1;
return toReturn;
}
}
public boolean isEmpty()
// Returns true if this queue is empty; otherwise, returns false.
{
return (numElements == 0);
}
public boolean isFull()
// Returns true if this queue is full; otherwise, returns false.
{
return (numElements == elements.length);
}
public int size()
// Returns the number of elements in this queue.
{
return numElements;
}
}
```
**Explanation of Key Code Sections:**
- **ArrayBoundedQueue Class:**
- This class implements a generic bounded queue using an array.
- `DEFCAP` is a
![```java
public class ArrayUnboundedQueue<T> implements QueueInterface<T>
{
protected final int DEFCAP = 100; // default capacity
protected T[] elements; // array that holds queue elements
protected int origCap; // original capacity
protected int numElements = 0; // number of elements in this queue
protected int front = 0; // index of front of queue
protected int rear; // index of rear of queue
public ArrayUnboundedQueue()
{
elements = (T[]) new Object[DEFCAP];
rear = DEFCAP - 1;
origCap = DEFCAP;
}
public ArrayUnboundedQueue(int origCap)
{
elements = (T[]) new Object[origCap];
rear = origCap - 1;
this.origCap = origCap;
}
private void enlarge()
// Increments the capacity of the queue by an amount
// equal to the original capacity.
{
// create the larger array
T[] larger = (T[]) new Object[elements.length + origCap];
// copy the elements from the smaller array into the larger array
int currSmaller = front;
for (int currLarger = 0; currLarger < numElements; currLarger++)
{
larger[currLarger] = elements[currSmaller];
currSmaller = (currSmaller + 1) % elements.length;
}
// update instance variables
elements = larger;
front = 0;
rear = numElements - 1;
}
public void enqueue(T element)
// Adds element to the rear of this queue.
{
if (numElements == elements.length)
enlarge();
rear = (rear + 1) % elements.length;
elements[rear] = element;
numElements = numElements + 1;
}
public T dequeue()
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it.
{
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue.");
else
{
T toReturn = elements[front];
elements[front] = null;
front = (front + 1) % elements.length](https://content.bartleby.com/qna-images/question/1b2c1e36-9974-49eb-8a0c-fe4d3c635f06/284c88fa-e731-488c-99c5-a84ea198b183/50weo3_thumbnail.png)
Transcribed Image Text:```java
public class ArrayUnboundedQueue<T> implements QueueInterface<T>
{
protected final int DEFCAP = 100; // default capacity
protected T[] elements; // array that holds queue elements
protected int origCap; // original capacity
protected int numElements = 0; // number of elements in this queue
protected int front = 0; // index of front of queue
protected int rear; // index of rear of queue
public ArrayUnboundedQueue()
{
elements = (T[]) new Object[DEFCAP];
rear = DEFCAP - 1;
origCap = DEFCAP;
}
public ArrayUnboundedQueue(int origCap)
{
elements = (T[]) new Object[origCap];
rear = origCap - 1;
this.origCap = origCap;
}
private void enlarge()
// Increments the capacity of the queue by an amount
// equal to the original capacity.
{
// create the larger array
T[] larger = (T[]) new Object[elements.length + origCap];
// copy the elements from the smaller array into the larger array
int currSmaller = front;
for (int currLarger = 0; currLarger < numElements; currLarger++)
{
larger[currLarger] = elements[currSmaller];
currSmaller = (currSmaller + 1) % elements.length;
}
// update instance variables
elements = larger;
front = 0;
rear = numElements - 1;
}
public void enqueue(T element)
// Adds element to the rear of this queue.
{
if (numElements == elements.length)
enlarge();
rear = (rear + 1) % elements.length;
elements[rear] = element;
numElements = numElements + 1;
}
public T dequeue()
// Throws QueueUnderflowException if this queue is empty;
// otherwise, removes front element from this queue and returns it.
{
if (isEmpty())
throw new QueueUnderflowException("Dequeue attempted on empty queue.");
else
{
T toReturn = elements[front];
elements[front] = null;
front = (front + 1) % elements.length
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Implement the complete stack functionality by using ArrayList which will containInteger values. C++arrow_forwardFor an array Based Implementation of Lists with Dynamic Allocation, why we should define a destructor by ourselves and what the destructor will do?arrow_forwardWhich list implementations would work well for implementing bags, stacks, and queues?arrow_forward
- Research Java’s standard libraries (the APIs that are provided as part of the language,not your own custom code). Demonstrate how to add five elements of type UACar toan reference-based list implementation. Obtain the first element in the list and sendit to the println() statement. Finally, remove the first and last element in the list.arrow_forwardCan you list some drawbacks of implementing Queues using arrays?arrow_forwardCreate a class called ResizingArrayQueueOfStrings that uses the queue abstraction using a fixed-size array. Then, as an extension, use array resizing in your implementation to get rid of the size restriction.arrow_forward
- The interface provided by a Queue ADT is a binding agreement between the implementer and the programmer. what do you think, true or falsearrow_forwardWrite a C++ program to implement the Queue Class. Write implementations for the methods of the Queue class. Write the main program to test all the methods implemented. The Queue class can be implemented to store integer values.arrow_forwardCompare the operations of ArrayLists and Linked Lists? What are the similarities and differences of both forms?arrow_forward
- Java Design and draw a method called check() to check if characters in a linked list is a palindrome or not e.g "mom" or "radar" or "racecar. spaces are ignored, we can call the spaces the “separator”. The method should receive the separator as a variable which should be equal to “null” when no separator is used.arrow_forwardYour crazy boss has assigned you to write a linear array-based implementation of the IQueue interface for use in all the company's software. You've tried explaining why that's a bad idea with logic and math, but to no avail. So - suppress your inner turmoil and complete the add() and remove() methods of the AQueue class and identify the runtime order of each of your methods. /** A minimal queue interface */ public interface IQueue { /** Add element to the end of the queue */ public void add(E element); /** Remove and return element from the front of the queue * @returns fırst element * @throws NoSuchElementException if queue is empty */ public E remove(); /** Not an ideal Queue */ public class AQueue implements IQueue{ private El) array: private int rear; public AQueue(){ array = (E[)(new Object[10]): rear = 0: private void expandCapacity) { if (rear == array.length) { array = Arrays.copyOf(array, array.length 2): @Override public void add(E element) { / TODO @Override public E…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
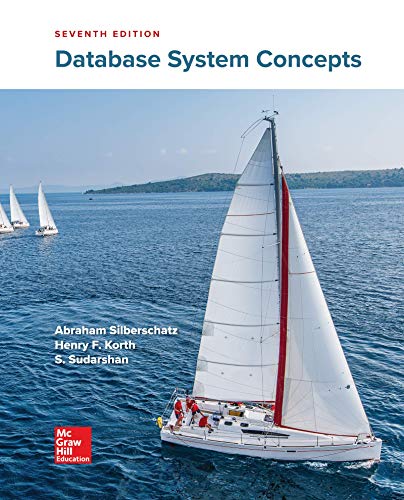
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
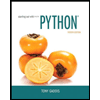
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
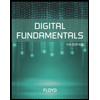
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
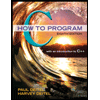
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
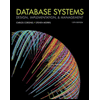
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
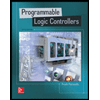
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education