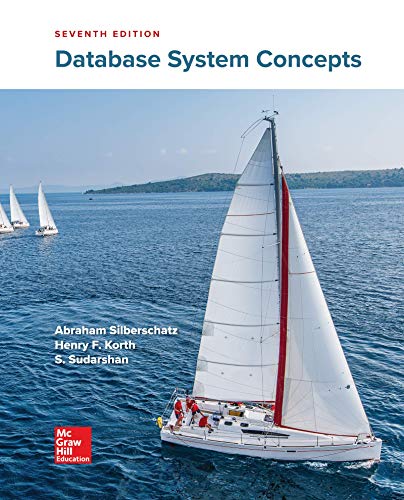
I need help with this Java problem to output as it's explained in the image below:
/**
*
* Node.java: Node class (Don't edit)
*/
public class Node implements NodeInterface<Integer>{
private Integer data;
private Node left;
private Node right;
public Node(int data){
this.data =data;
left=right=null;
}
public Integer getData(){
return data;
}
public Node getLeft(){
return left;
}
public Node getRight(){
return right;
}
public void setLeft(Node node){
this.left = node;
}
public void setRight(Node node){
this.right = node;
}
public String toString(){
return ""+data;
}
}
(NodeInterface.java) (Don't edit)
interface NodeInterface<T>{
T getData();
NodeInterface<T> getLeft();
NodeInterface<T> getRight();
}
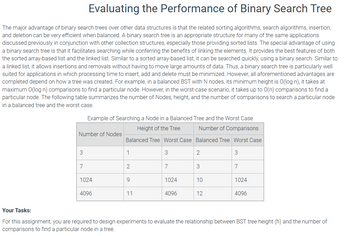
to generate a solution
a solution
- Fix the code below so that there is a function that calculate the height of the triangle. package recursion; import javax.swing.*;import java.awt.*; /** * Draw a Sierpinski Triangle of a given order on a JPanel. * * */public class SierpinskiPanel extends JPanel { private static final int WIDTH = 810; private static final int HEIGHT = 830; private int order; /** * Construct a new SierpinskiPanel. */ public SierpinskiPanel(int order) { this.order = order; this.setMinimumSize(new Dimension(WIDTH, HEIGHT)); this.setMaximumSize(new Dimension(WIDTH, HEIGHT)); this.setPreferredSize(new Dimension(WIDTH, HEIGHT)); } public static double height(double size) { double h = (size * Math.sqrt(3)) / 2.0; return h; } /** * Draw an inverted triangle at the specified location on this JPanel. * * @param x the x coordinate of the upper left corner of the triangle * @param y the y…arrow_forwardclass Queue { private static int front, rear, capacity; private static int queue[]; Queue(int c) { front = rear = 0; capacity = c; queue = new int[capacity]; } static void queueEnqueue(int data) { if (capacity == rear) { System.out.printf("\nQueue is full\n"); return; } else { queue[rear] = data; rear++; } return; } static void queueDequeue() { if (front == rear) { System.out.printf("\nQueue is empty\n"); return; } else { for (int i = 0; i < rear - 1; i++) { queue[i] = queue[i + 1]; } if (rear < capacity) queue[rear] = 0; rear--; } return; } static void queueDisplay() { int i; if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } for (i = front; i < rear; i++) { System.out.printf(" %d <-- ", queue[i]); } return; } static void queueFront() { if (front == rear) { System.out.printf("\nQueue is Empty\n"); return; } System.out.printf("\nFront Element is: %d", queue[front]);…arrow_forwardJava programming language I have to create a remove method that removes the element at an index (ind) or space in an array and returns it. Thanks! I have to write the remove method in the code below. i attached the part where i need to write it. public class ourArrayList<T>{ private Node<T> Head = null; private Node<T> Tail = null; private int size = 0; //default constructor public ourArrayList() { Head = Tail = null; size = 0; } public int size() { return size; } public boolean isEmpty() { return (size == 0); } //implement the method add, that adds to the back of the list public void add(T e) { //HW TODO and TEST //create a node and assign e to the data of that node. Node<T> N = new Node<T>();//N.mData is null and N.next is null as well N.setsData(e); //chain the new node to the list //check if the list is empty, then deal with the special case if(this.isEmpty()) { //head and tail refer to N this.Head = this.Tail = N; size++; //return we are done.…arrow_forward
- Starter code for ShoppingList.java import java.util.*;import java.util.LinkedList; public class ShoppingList{ public static void main(String[] args) { Scanner scnr=new Scanner(System.in); LinkedList<ListItem>shoppingList=new LinkedList<ListItem>();//declare LinkedList String item; int i=0,n=0;//declare variables item=scnr.nextLine();//get input from user while(item.equals("-1")!=true)//get inputuntil user not enter -1 { shoppingList.add(new ListItem(item));//add into shoppingList LinkedList n++;//increment n item=scnr.nextLine();//get item from user } for(i=0;i<n;i++) { shoppingList.get(i).printNodeData();//call printNodeData()for each object } }} class ListItem{ String item; //constructor ListItem(String item) { this.item=item; } void printNodeData() { System.out.println(item); }}arrow_forwardRun the program to tell me there is an error, BSTChecker.java:3: error: duplicate class: Node class Node { ^ BSTChecker.java:63: error: class LabProgram is public, should be declared in a file named LabProgram.java public class LabProgram { ^ 2 errors This is my program, please fix it. import java.util.*; class Node { int key; Node left, right; public Node(int key) { this.key = key; left = right = null; } } class BSTChecker { public static Node checkBSTValidity(Node root) { return checkBSTValidityHelper(root, null, null); } private static Node checkBSTValidityHelper(Node node, Integer min, Integer max) { if (node == null) { return null; } if ((min != null && node.key <= min) || (max != null && node.key >= max)) { return node; } Node leftResult = checkBSTValidityHelper(node.left, min, node.key); if (leftResult != null) { return leftResult; } Node rightResult = checkBSTValidityHelper(node.right, node.key, max); if (rightResult != null) { return rightResult; }…arrow_forwardPlease use the information in the second screenshot about the BST class and Node class to create a class BSTApp. Please make sure to use the following code as a starting point. import java.util.*; public class BSTTest{public static void main(String[] args) {// perform at least one test on each operation of your BST } private int[] randomArray(int size) {// remove the two linesint[] arr = new int[1];return arr;} // the parameters and return are up to you to define; this method needs to be uncommented// private test() {//// }} Base of class Node public class Node {int key;Node left, right, parent; public Node() {} public Node(int num) {key = num;left = null;right = null;parent = null;}} Base of class BST import java.util.*; class BST {// do not change thisprivate Node root;private ArrayList<Integer> data; // DO NOT MODIFY THIS METHODpublic BST() {root = null;data = new ArrayList<Integer>(0);} // DO NOT MODIFY THIS METHODpublic ArrayList<Integer> getData() {return…arrow_forward
- package circularlinkedlist;import java.util.Iterator; public class CircularLinkedList<E> implements Iterable<E> { // Your variablesNode<E> head;Node<E> tail;int size; // BE SURE TO KEEP TRACK OF THE SIZE // implement this constructorpublic CircularLinkedList() {} // I highly recommend using this helper method// Return Node<E> found at the specified index// be sure to handle out of bounds casesprivate Node<E> getNode(int index ) { return null;} // attach a node to the end of the listpublic boolean add(E item) {this.add(size,item);return false; } // Cases to handle// out of bounds// adding to empty list// adding to front// adding to "end"// adding anywhere else// REMEMBER TO INCREMENT THE SIZEpublic void add(int index, E item){ } // remove must handle the following cases// out of bounds// removing the only thing in the list// removing the first thing in the list (need to adjust the last thing in the list to point to the beginning)// removing the last…arrow_forwardThe language is Javaarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
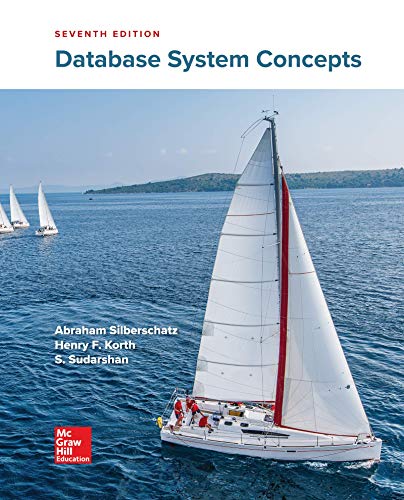
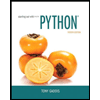
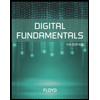
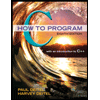
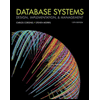
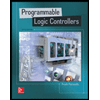