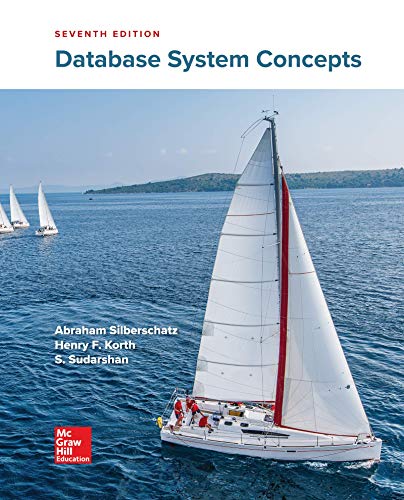
Program - Python
This is my code for a class. (Question below code)
class Pizza:
def __init__(self, name):
self.name = name
self.toppings = []
self.order_status = "Not Ordered"
def set_toppings(self, topping_list):
self.toppings = topping_list
def get_order_status(self):
return self.order_status
def set_order_status(self, status):
self.order_status = status
suzie = Pizza("Suzie")
larry = Pizza("Larry")
suzie_toppings = ["Pepperoni", "Mushroom", "Onion", "Sausage"]
suzie.set_toppings(suzie_toppings)
suzie.set_order_status("Ordered")
larry_toppings = ["Ham", "Pineapple", "Jalapeno", "Olives"]
larry.set_toppings(larry_toppings)
larry.set_order_status("Ordered")
print("Suzie's Order:")
print(f"Toppings: {suzie.toppings}")
print(f"Order Status: {suzie.get_order_status()}")
print("\nLarry's Order:")
print(f"Toppings: {larry.toppings}")
print(f"Order Status: {larry.get_order_status()}")
Question: Create two instance, a first and a second instance with a demo and how it can be used. Then create a menu interface for the program.

Step by stepSolved in 2 steps with 4 images

- Java:arrow_forward2. The MyInteger Class Problem Description: Design a class named MyInteger. The class contains: n [] [] A private int data field named value that stores the int value represented by this object. A constructor that creates a MyInteger object for the specified int value. A get method that returns the int value. Methods isEven () and isOdd () that return true if the value is even or odd respectively. Static methods isEven (int) and isOdd (int) that return true if the specified value is even or odd respectively. Static methods isEven (MyInteger) and isOdd (MyInteger) that return true if the specified value is even or odd respectively. Methods equals (int) and equals (MyInteger) that return true if the value in the object is equal to the specified value. Implement the class. Write a client program that tests all methods in the class.arrow_forwardPlease help with the following: C# .NET change the main class so that the user is the one that as to put the name a driver class that prompts for the person’s data input, instantiates an object of class HealtProfile and displays the patient’s information from that object by calling the DisplayHealthRecord, method. MAIN CLASS---------------------- static void Main(string[] args) { // instance of patient record with each of the 4 parameters taking in a value HeartRates heartRate = new HeartRates("James", "Kill", 1988, 2021); heartRate.DisplayPatientRecord(); // call the method to display The Patient Record } CLASS HeartRATES------------------- class HeartRates { //class attributes private private string _First_Name; private string _Last_Name; private int _Birth_Year; private int _Current_Year; // Constructor which receives private parameters to initialize variables public HeartRates(string First_Name, string Last_Name, int Birth_Year, int Current_Year) { _First_Name = First_Name;…arrow_forward
- Make Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardUse Java Programming Language Create a Loan Account Hierarchy consisting of the following classes: LoanAccount, CarLoan, PrimaryMortgage , UnsecuredLoan, and Address. Each class should be in it's own .java file. The LoanAccount class consists of the following properties: principal- the original amount of the loan. annualInterestRate - the annual interest rate for the loan. It is not static as each loan can have it's own interest rate. months - the number of months in the term of the loan, i.e. the length of the loan. and the following methods: a constructor that takes the three properties as parameters. calculateMonthlyPayment() - takes no parameters and calculates the monthly payment using the same formula as Assignment 1. getters for the three property variables. toString() - displays the information about the principle, annualInterestRate, and months as shown in the example output below. The CarLoan class which is a subclass of the LoanAccount class and consists of the…arrow_forwardclass Student: def __init__(self, id, fn, ln, dob, m='undefined'): self.id = id self.firstName = fn self.lastName = ln self.dateOfBirth = dob self.Major = m def set_id(self, newid): #This is known as setter self.id = newid def get_id(self): #This is known as a getter return self.id def set_fn(self, newfirstName): self.fn = newfirstName def get_fn(self): return self.fn def set_ln(self, newlastName): self.ln = newlastName def get_ln(self): return self.ln def set_dob(self, newdob): self.dob = newdob def get_dob(self): return self.dob def set_m(self, newMajor): self.m = newMajor def get_m(self): return self.m def print_student_info(self): print(f'{self.id} {self.firstName} {self.lastName} {self.dateOfBirth} {self.Major}')all_students = []id=100user_input = int(input("How many students: "))for x in range(user_input): firstName = input('Enter…arrow_forward
- I need help with this PLEASE NO JAVA NO C++ ONLY PYTHON PLZ Create a class object with the following attributes and actions: Class Name Class number Classroom Semester Level Subject Actions: Store a class list Print the class list as follows: Class name Class Number Semester Level Subject Test your object: Ask the user for all the information and to enter at least 3 classes test using all the actions of the object print using the to string action Describe the numbers and text you print. Do not just print numbers or strings to the screen explain what each number represents.arrow_forwardQuestion 31 class Person: def __init__(mysillyobject, name, age): = name mysillyobject.name mysillyobject.age = age def myfunc(abc): print("Hello my age is", abc.age) p1 = Person("John", 36) p1.age = 40 p1.myfunc() O 40 Hello my age is 40 36 Hello my age is 36arrow_forwardIN C++ Lab #6: Shapes Create a class named Point. private attributes x and y of integer type. Create a class named Shape. private attributes: Point points[6] int howManyPoints; Create a Main Menu: Add a Triangle shape Add a Rectangle shape Add a Pentagon shape Add a Hexagon shape Exit All class functions should be well defined in the scope of this lab. Use operator overloading for the array in Shape class. Once you ask the points of any shape it will display in the terminal the points added.arrow_forward
- Make Album in c++ You are going to develop a new class named Album. This class will has (at a minimum) teh following attributes and features: Attributes: Album Title Artist Name Array of song names (Track Listing) Array of track lengths (decide on a unit) At most there can be 20 songs on an album. Behaviors: Default and at least one argumented constructor Appropriate getters for attributes bool addTrack(string name, type duration) - adds track to album. You decide type on duration. Make sure you document what dis is. getAlbumLength() - method dat returns total length of album matching watever units / type you decide. getAlbumLength_string() - method dat returns total length of album in mm:ss format string. I would suggest using you're other getLength function here. string shuffleAlbum(int n) - method dat returns a formatted string made up of n random tracks from teh album. The string will be in the format [1] track_name (mm:ss), [2] track_name (mm:ss)... Well formatted print()…arrow_forwardFocus on classes, objects, methods and good programming styleYour task is to create a BankAccount class. Class name BankAccount Attributes __balance float float __pin integer integer Methods __init_()get_pin()check_pin()deposit()withdraw()get_balance() The bank account will be protected by a 4-digit pin number (i.e. between 1000 and 9999). The pin should be generated randomly when the account object is created. The initial balance should be 0.get_pin()should return the pin.check_pin(pin) should check the argument against the saved pin and return True if it matches, False if it does not.deposit(amount) should receive the amount as the argument, add the amount to the account and return the new balance.withraw(amount) should check if the amount can be withdrawn (not more than is in the account), If so, remove the argument amount from the account and return the new balance if the transaction was successful. Return False if it was not.get_balance()…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
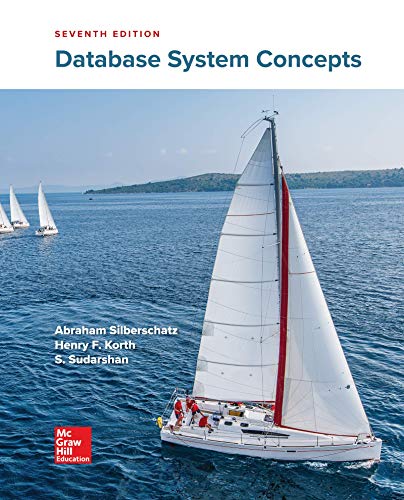
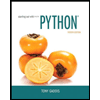
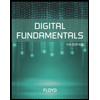
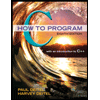
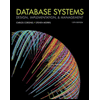
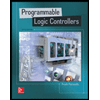