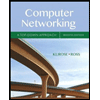
Programming Assignment:
Here is a loop that starts with an initial estimate of the square root of a, x, and improves it until it
stops changing:
while True:
y = (x + a / x) / 2
if abs(y - x) < epsilon:
break
x = y
where epsilon has a value like 0.0000001 that determines how close is close enough.
Encapsulate this loop in a function called square_root that takes a as a parameter, chooses a
reasonable value of x, and returns an estimate of the square root of a.
To test the square root
function named test_square_root that prints a table like this:
1.0 1.0 1.0 0.0
2.0 1.41421356237 1.41421356237 2.22044604925e-16
3.0 1.73205080757 1.73205080757 0.0
4.0 2.0 2.0 0.0
5.0 2.2360679775 2.2360679775 0.0
6.0 2.44948974278 2.44948974278 0.0
7.0 2.64575131106 2.64575131106 0.0
8.0 2.82842712475 2.82842712475 4.4408920985e-16
9.0 3.0 3.0 0.0
The first column is a number, a; the second column is the square root of a computed with the
[your function]; the third column is the square root computed by math.sqrt; the fourth column
is the absolute value of the difference between the two estimates.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

- 60 points Write a function which takes one parameter int num, and prints out a countdown timer with minutes and seconds separated by a colon (:). It should print out one line for each second elapsed and then pause one second before printing out the next line. After it has done all of this, it should call a special function ringAlarm () to sound an alarm. A few things to note: - You can assume that calling the function sleepytime () makes the program pause for one second - You can assume that calling the function ringAlarm () makes the program sound an alarm - It should count down from num minutes:zero seconds to zero minutes:zero seconds - Your function must have the following signature: void timer (int num) You do not need to demonstrate calling this function from main(). You don't need to format the output nicely; the below is acceptable output for calling this function when num is 10: 10:0 9:59 9:58 //...and so on, all the way down to: 9:1 9:0 8:59 8:58 //..and so on, all the way…arrow_forwardThe function below does not work as expected: If sales is a negative number is returns 3 instead of returning 0. Please help me correct it. /*This function determines the commision percent based on the following table:Sales Commission$0 - 1000 3%1001 - 5000 4.5%5001 - 10,000 5.25%over 10,000 6%*/double Sales::detCommissionPercent() const{double commission = 0;if ( amountSold > 0){if(amountSold<= 1000) // if amountSold is between [0, 1000] commission = 3;else if(amountSold<=5000)// if amountSold is between [1001, 5000] commission = 4.5;else if(amountSold<=10000) // if amountSold is between [5001, 10000] commission = 5.25;else commission = 6;}return commission;}arrow_forwardExample code that will for example count the number of people visting a park each day of the week, and determine the day on which the most vists occured using loops. No functions can be used.arrow_forward
- ] ] has_perfect You are to write a function has "perfect (graph)" that takes in a BIPARTITE graph as its input, and then determintes whether or not the graph has a perfect matching. In other words, it will return the Boolean value True if it has one, and False if it does not. After compiling the above cell, you should be able to compile the following cell and obtain the desired outputs. print (has perfect({"A" : ["B", "C"], "B" : ["A", "D"], "C" : ["A", "D"], "D" : ["B", "C"]}), has perfect ( {"A" : ["B"], "B" : ["A", "D", "E"], "C" : ["E"], "D":["B"], "E": ["B","C","F"], "F":["E"]})) This should return True False Python Pythonarrow_forwardin C++ Write an inductive function, called IndFn, for adding 12+10+8+6+4 Write a function with a procedural loop, called ProFun, for adding 14+12+10+8+6+4;arrow_forwardin the C++ version please suppose to have a score corresponding with probabilities at the end and do not use the count[] function. Please explain the detail when coding. DO NOT USE ARRAY. The game of Pig The game of Pig is a dice game with simple rules: Two players race to reach 100 points. Each turn, a player repeatedly rolls a die until either a 1 ("pig") is rolled or the player holds and scores the sum of the rolls (i.e. the turn total). At any time during a player's turn, the player is faced with two decisions: roll - if the player rolls 1: the player scores nothing and it becomes the opponents turn. 2 - 6: the number is added to the player's turn total and the player's turn continues. hold - The turn total is added to the player's score and it becomes the opponent's turn. This game is a game of probability. Players can use their knowledge of probabilities to make an educated game decision. Assignment specifications Hold-at-20 means that the player will choose to roll…arrow_forward
- A Circle and Two Squares Imagine a circle and two squares: a smaller and a bigger one. For the smaller one, the circle is a circumcircle and for the bigger one, an incircle. Create a function, that takes an integer (radius of the circle) and returns the difference of the areas of the two squares. Examples square_areas_difference (5) → 50arrow_forward5. use c code to Write a stringSearch function that gets two strings one called needle and the other called haystack. It then searches in the Haystack for the needle. If it finds it, it returns the index of where the needle starts in the haystack. If the needle cannot be found, it should return -1 Prototype: int stringSearch(char needle[], char haystack) Example1: Haystack: “This is just an example” Needle: “just” Result: 8 Example2: Haystack: “This is just an example” Needle: “This” Result: 0 Example3: Haystack: “This is just an example” Needle: “this” Result: -1 Hint: all the answer need to include an output and use c code to answerarrow_forwardThe parameters passed to function are .called formal parameters True O Fales Oarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
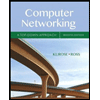
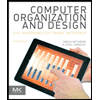
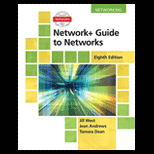
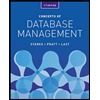
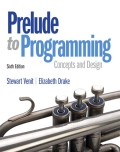
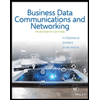