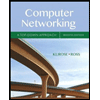
Project to create a dictionary as a binary tree using java code.
- Data structure : A word in a dictionary will keep the key (the defined word) and a definition (another string). For each node we need to keep a link to the left subtree containing the words before the current word and a link to the right subtree containing the words after the current word.
- Test and initialize : a method that will initialize a binary tree with 15 terms from the textbook. Provide correct names and definitions for the test data.
- Print a binary tree : a method that will print a binary tree in the following format.
LEFT NODE
ROOT NODE
RIGHT NODE
For instance, a valid tree is shown below, please disregard the bullets:
Need to use a recursive function that will receive the number of spaces before a node (4 spaces for each level), and a node. The function will do nothing if the node is null. If the node has a left subtree, call it recursively to print the subtree, but with more spaces. Then print the spaces and the node key. Then print recursively the right subtree with more spaces. Test the method by showing the tree after each operation performed in step 2.
- Find a word in the dictionary : Need a method that will receive a word and return the definition if the word is in dictionary or null otherwise. Test the method with two words from dictionary and two not in the dictionary.
- Insert a word : Need a method that will receive a word and its definition. If the word is in dictionary, update the definition. If not, add the word to the dictionary. Test the method with two refined definitions and two new definitions. Print the dictionary after each operation.
- Check if leaf, single leaf and two leaves : Creating three methods that will check if a word is a leaf, has a single child or has 2 children. Returns true if the property holds, otherwise false. Check the method with various words: 2 leafs, 2 single child, 2 two children and 2 not in the dictionary. Print the dictionary after each operation.
- Delete a leaf node : Need a method that will receive a word and if the word is a leaf delete the node from the dictionary. Check the method with various words: 2 leafs, 2 single child, 2 two children and 2 not in the dictionary. Print the dictionary after each operation.
One way to implement is: create a method that will receive a subtree root node and a key to delete. If the node is a leaf and has the same key remove the node from the tree and return the resulted subtree. The caller must link the subtree.
- Delete a node with a child : Need a method that will receive a word and if the word has a single child delete the node from the dictionary. Check the method with various words: 2 leafs, 2 single child, 2 two children and 2 not in the dictionary. Print the dictionary after each operation.
- Delete a node with two children : Need a method that will receive a word and if the word has two children delete the node from the dictionary. Check the method with various words: 2 leafs, 2 single child, 2 two children and 2 not in the dictionary. Print the dictionary after each operation.
- Delete any node : Combine the previous 3 delete methods to create one method to delete any node. Check the method with various words: 2 leafs, 2 single child, 2 two children and 2 not in the dictionary. Print the dictionary after each operation.
- First and last word : Need a method that will return the first and the last word from the dictionary. Check the methods.
- Preorder : Need a method that will traverse the tree in preorder and show the words in the console. Check the method.
- Inorder : Need a method that will traverse the tree in inorder and show the words in the console. Check the method.
- Postorder : Need a method that will traverse the tree in postorder and show the words in the console. Check the method.
- Height of the tree : Need a method that will compute the height tree. Check the method.
- Find the word with longest definition: Need a method that will compute the word with the longest definition. Check the method.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- PYTHON programming Binary Search in Python In the Lecture, we implemented a binary search algorithm in Java where keys were the data values. Implement a similar program in Python. Binary Search Tree Interfaceget(key) get the value key from treeset(key) insert value key into treesize() return number of non-empty nodesheight() return height of treerebalance()arrow_forwardIn Java for the RedBlackTree how would you make the InsertFixup and RemoveFixup with the following RedBlackTree and BInNode class... public class BinNode{private String data;private BinNode left;private BinNode right;private int height; //height field as with AVL tree or Red Black Treeprivate boolean color; //Needed for Red Black Treepublic BinNode(){data = "";left = null;right = null;}public int getHeight() {return height;}public void setHeight(int height) {this.height = height;}public BinNode(String d){data = d;left = null;right = null;}public void setData(String d){this.data = d;}public String getData(){return this.data;}public void setLeft(BinNode l){this.left = l;}public BinNode getLeft(){return this.left;}public void setRight(BinNode r){this.right = r;}public BinNode getRight(){return this.right;}} public class RedBlackTree {private BinNode root;private BinNode nil;public RedBlackTree() {nil = new BinNode();nil.setColor(false); // Black color for NIL nodesroot = nil;}public…arrow_forwardWrite the java code to get the 10 out of the tree.arrow_forward
- JAVA PLEASE : Assume the Tree consists of your ID digits, inserted in the fashion to minimize the height of the tree. Remove duplicates if needed. Trace the delete() method above in a similar fashion as the insert was traced, delete 3 last digits of your ID from the tree in the same order as they are present in you ID.arrow_forwardRead the tree from simple.dnd file and after coloring each branch with a different color write these two trees in tree.phy file.arrow_forwardConstruct a binary search tree from the list of the following keys using inserts: 18 2 9 6 7 4 3 20 16 17 8 12 5 4 The key 18 is the first one that will be inserted into the tree.Draw the final tree and 3 other trees to show the intermediate steps.Do an inorder traversal of the tree. What's the list you get?arrow_forward
- Write a method isBST() that takes a Node as argument and returns true if the argument node is the root of a binary search tree, false otherwise.Hint : This task is also more difficult than it might seem, because the order in which youcall the methods in the previous three exercises is importantarrow_forwardWhen presenting a menu option to the user in the main cpp file. Asking user to input in an ID, how do I code a search method implementation where it takes that ID and search it in the binary tree and either return true if found and false if not. I am trying to steer away from using item which is attached to both (ID, username). I am trying to only take the ID input and the node pointer and traversing through the binary tree to find if the ID matches any of the nodes.arrow_forwardIn java: binary tree Write a method called findTotalParents (, this method will find all the nodes that considered to be parents with any dependency and return the total number of parents. The public method shall not take any parameters, but the private method takes a BNode as a parameter, representing the current root.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
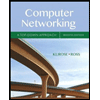
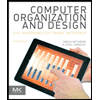
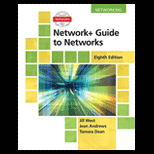
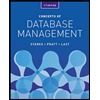
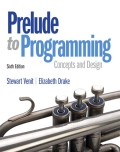
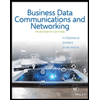