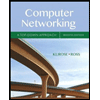
PYTHON CODING
This function takes a list of points and then returns a new list of points, which starts with the first point that was given in the list, and then followed by points closest to the start point.
Example:
points = [a, b, c, d, e, f, g]
point a is the strating point soe the new list must start with a. we then use the fistance function to calculate which points will be closest to a. lets say f is closest to a, therefore, second point in the new list will be f. Next we find which point is closest to f, lets say b is closest, therefore b is the next point in our new list, and so on.
new list = [a, f, b, ......]
-------
Here is the function that needs to be created:
def solution_path(points) :
# insert code here
return path
Please use any of the following function to develop the solution_path function
Distance function - calculates the distance between two points
def distance(p1, p2) :
distance = sqrt (((p1 [0] - p2 [0]) **2) + ((p1 [1] - p2 [1]) **2))
return (distance)
Find_closest function - calculates the closest point to the starting point
def find_closest(start_point, remaining_points):
closest_distance = 99999
for coordinate in remaining_points:
dist = distance(start_point, coordinate)
if(dist < closest_distance):
closest_distance = dist
closest_point = coordinate
return closest_point
Path_distance function - calculates the total distance between all points
def path_distance(path) :
total_distance = 0
for element in range(0, len(path) - 1):
total_distance += distance(path[element], path[element + 1])
return total_distance
Note: the function should return a new list, not modify the existing list.
Hint: use a while loop to repeat while the list of remaining points is not empty
Here is an exmaple of the input and output of the function:
points = [(5, 8), (5.5,3), (3.5,1.5), (2.5,3.5), (7.5,9), (1,3), (7,9.5), (9,2), (1,0), (6,8), (3,7.5), (8,2.5)]
path = solution_path(points)
print(path)
# the first point in the input list is (5, 8), so that appears first in our output list,
# the closest point to our start point (5, 8) is (6, 8), so (6, 8) appears second in our list,
# the closest remaining point to (6, 8) is (7.5, 9), so (7.5, 9) appears third in our list,
# and so on. So the best path should be
# [(5, 8), (6, 8), (7.5, 9), (7, 9.5), (3, 7.5), (2.5, 3.5), (1, 3), (3.5, 1.5), (5.5, 3), (8, 2.5), (9, 2), (1, 0)]

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- In python code: Write a function to convert the Roman Numeral list to Hexidecimal. DCXXXVIIDCCXXXIIICMLXXXIIICDLXXXVIICCDLXXVIIIDCLXCMXVIDCCXXXCDXCIXCDLXXIIIDCCXLIIDCCLVIICCCXXXVICXXXIXCCCXXCCLXXVIIICMXXVIICCCIXDCCXXIXCDXCLXXIVCCXXXICCXXIIDCLXXXCCCLXXVIICDLIXCCXXIICCLVDXCIVDXXXIIICCLXIIIDCCCXDCCLXXIVDCCXXIVDCXXIICDXXCCCLXVDXCCLXXIICLXXXIXDCXXIXCMIDCXCVXXVIICCCLIVDCCXLVICMXLIIDCLXIVDCCCMLXXXIVCMLXIXCDLXXVIIICCLXVCCCLXXCCCLXXIICMXXIICCVIIICLXXVIIXCIXDXXXIICDXXXVDCCCLIXCDXCIIDLVDCCCXLVIICXXXVICDLXIVDVIIICCXCVIIIDCCXIXDCCCXXXVDCCCXICDVIICCCXCIXIDLXXCCLXIXCCCXIIDCCCXXVIIIDCCCIVCCCLXVCLXXXVIIDXVDXCIICMXLIVDCCXVIDLXCDLXXIXDCCCXCIIDCXLVICLXIDXCVIIICLXXDCCLXXVDXCIIDLXXVIarrow_forwardin phython language Write a function named getOddList(). The function takes one parameter which is a list(e.g. inputlist). The function will find the odd numbers in the list and return it as a new list. Call the function with a sample list given as an argument, then print the oddlist. Sample output for a sample list [-4,6,4,7,13,78,9]: [7,13,9]arrow_forward7. Given two lists A and B, write a function to compute the difference A – B, where A – B is the list of elements that are in A but not in B. For example, if A is '(6 7 8 9) and B is (7 9) then A – B is '(6 8).arrow_forward
- 1 - Write a Python function that will do the following: 1. Ask the user to input an integer that will be appended to a list that was originally empty. This will be done 5 times, meaning that when the input is complete, the list will have five elements (all integers). a. Determine whether each element is an even number or an odd number b. Return a list of five string elements "odd" and "even" that map the indexes of the elements of the input list, according to whether these elements are even or odd numbers.For example: if the input sequence is 12, 13, 21, 51, and 30, the function will return the list ["even", "odd", "odd", "odd", "even"].arrow_forwardPlease help me, create your won code Create a function that takes a 2D list and an integer v, and returns the number of times the value v is in the list of lists. Add the Nsteps variable to count how many times the loops execute and display a message.• The main program must give a 2D list (no need to generate it, just create it manually), call the function, and display the result example it's the picturearrow_forwardin PYTHON Write a function that accepts a list as an argument and calculates the sum of each elementof the list.arrow_forward
- The function interleave_lists in python takes two parameters, L1 and L2, both lists. Notice that the lists may have different lengths. The function accumulates a new list by appending alternating items from L1 and L2 until one list has been exhausted. The remaining items from the other list are then appended to the end of the new list, and the new list is returned. For example, if L1 = ["hop", "skip", "jump", "rest"] and L2 = ["up", "down"], then the function would return the list: ["hop", "up", "skip", "down", "jump", "rest"]. HINT: Python has a built-in function min() which is helpful here. Initialize accumulator variable newlist to be an empty list Set min_length = min(len(L1), len(L2)), the smaller of the two list lengths Use a for loop to iterate k over range(min_length) to do the first part of this function's work. On each iteration, append to newlist the item from index k in L1, and then append the item from index k in L2 (two appends on each iteration). AFTER the loop…arrow_forwardPlease help. This is Python I feel so lostarrow_forwardComputer Science Write a program in F#, listOfPrime, to construct a list of all prime numbers within a given range of integers. Example: listOfPrime 5 17 val it : int list = [7; 11; 13] (Note: Do not read input, instead use the arguments to the function. Do not print the output, instead, return values as specified. No loops, while, for,, if etc.)arrow_forward
- python help, please show all steps and include comments Write a function largerLst() that takes a list of numbers (i.e., int or float values) as a parameter and returns a new list constructed out of it. The list returned has all the values in the parameter list that are larger than the element immediately to their right, that is, larger than their right neighbor. If the parameter list is empty or there are no values larger than their right neighbor, then the function returns an empty list. Note that the last element in the list will never be included in the list returned since it does not have a right neighbor. The function should not change the parameter list in any way. Note that a correct solution to this problem must use an indexed loop That is, one that makes a call to the range() function to generate indices into the list which are used to solve the problem (Review lecture notes 8-1). A solution that does not use an indexed loop will not be accepted. Note in particular that you…arrow_forwardIn python, Problem Description:You are hosting a party and do not have room to invite all of your friends. You use the following unemotional mathematical method to determine which friends to invite. Number your friends 1, 2, . . . , K and place them in a list in this order. Then perform m rounds. In each round, use a number to determine which friends to remove from the ordered list. The rounds will use numbers r1, r2, . . . , rm. In round i remove all the remaining people in positions that are multiples of ri (that is, ri, 2ri, 3ri, . . .) The beginning of the list is position 1. Output the numbers of the friends that remain after this removal process. Input Specification:The first line of input contains the integer K (1 ≤ K ≤ 100). The second line of input contains the integer m (1 ≤ m ≤ 10), which is the number of rounds of removal. The next m lines each contain one integer. The ith of these lines (1 ≤ i ≤ m) contains ri ( 2 ≤ ri ≤ 100) indicating that every person at a position…arrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
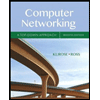
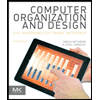
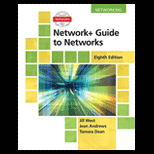
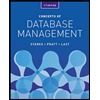
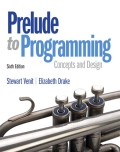
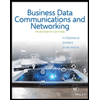