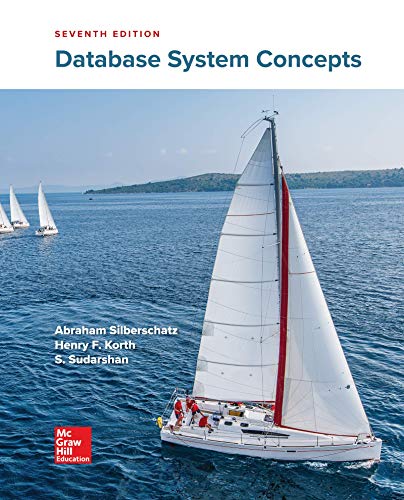
***PYTHON*** I need a
- use the list of tuples given as a starting point for data.
-
option 1 should print the full list of tuples, and the printing needs to be
formatted so that the data lines up neatly.
-
option 2 needs to work so that a user can enter the name, destination,
and mileage for a new trip, and have that data be added as a tuple to the
list.
-
options 3 need to allow filtering the list by name
- option 4 just needs to allow the program to end
-
if the user does not enter 1, 2, 3 or 4 as an option, you need to print an
error message and have them re-enter their choice.
-
there needs to be a main function that kicks things off, and you need to
write a function to print the whole list, a function that allows inputting a
new trip, and a function that filters by name and prints all the trips for
that given name. These functions are called when the user types in a
given option from the menu.
-
if you add a new trip, everything should still work
The menu options must be:
Program Options.
- Display all trips
- Input new trip
- Display trips by Name
- Exit
Each of the first 3 options should call a function to accomplish the proper task.
Tuples:
trips = [
(“alice”, ‘chicago’, 302),
(“bruce”, ‘chicago’, 309),
(“david”, ‘chicago’, 307),
(“carol”, ‘columbus’, 212),
(“alice”, ‘chicago’, 304),
(“bruce”, ‘chicago’, 301),
(“david”, ‘columbus’, 215),
(“alice”, ‘chicago’, 302),
(“carol”, ‘chicago’, 305),
(“bruce”, ‘chicago’, 304),
(“carol”, ‘columbus’, 218),
(“alice”, ‘columbus’, 217),
(“carol”, ‘chicago’, 309)
(“david”, columbus, 219)

Step-1: Start
Step-2: Define function display_trips(trips)
Step-2.1: Start loop for name, destination, mileage in trips
Step-2.1.1: Print Name, Destination and Mileage
Step-3: Define function input_new_trip(trips)
Step-3.1: Declare variable name and take input from the user
Step-3.2: Declare variable destination and take input from the user
Step-3.3: Declare variable mileage and take input from the user
Step-3.4: In trips append (name, destination, mileage)
Step-3.5: Print "Trip added successfully!"
Step-4: Define function display_trips_by_name(trips)
Step-4.1: Declare variable name_to_filter and take input from the user
Step-4.2: Declare variable filtered_trips and assign [(name, destination, mileage) for name, destination, mileage in trips if name == name_to_filter]
Step-4.3: If filtered_trips
Step-4.3.1: Print "Trips for (name_to_filter)"
Step-4.3.2: Call function display_trips and pass argument filtered_trips
Step-4.4: Else print "No trips found for (name_to_filter)."
Step-5: Define main function
Step-5.1: Declare variable trips and assign [
("alice", 'chicago', 302),
("bruce", 'chicago', 309),
("david", 'chicago', 307),
("carol", 'columbus', 212),
("alice", 'chicago', 304),
("bruce", 'chicago', 301),
("david", 'columbus', 215),
("alice", 'chicago', 302),
("carol", 'chicago', 305),
("bruce", 'chicago', 304),
("carol", 'columbus', 218),
("alice", 'columbus', 217),
("carol", 'chicago', 309),
("david", 'columbus', 219)
]
Step-5.2: Start loop while True
Step-5.2.1: Print "Program Options:"
Step-5.2.2: Print "1. Display all trips"
Step-5.2.3: Print "2. Input new trip"
Step-5.2.4: Print "3. Display trips by Name"
Step-5.2.5: Print "4. Exit"
Step-5.2.6: Declare variable choice and take input from the user
Step-5.2.7: If choice is equal to 1 then call function display_trips and pass argument trips
Step-5.2.8: Elif choice is equal to 2 then call function input_new_trip and pass argument trips
Step-5.2.9: Elif choice is equal to 3 then call function display_trips_by_name and pass argument trips
Step-5.2.10: Elif choice is equal to 4 then print "Exiting the program." and break
Step-5.2.11: Else print "Invalid choice. Please enter 1, 2, 3, or 4."
Step-6: if __name__ == "__main__":
Step-6.1: Call function main()
Step-7: Stop
Step by stepSolved in 4 steps with 5 images

- Write in JAVA When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This adjustment can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Assume that the list will always contain fewer than 20 floating-point values. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:System.out.printf("%.2f", yourValue);arrow_forwardPython programming only NEED HELParrow_forwardplease use your own code for better explanationarrow_forward
- Please do fastarrow_forward1 - Write a Python function that will do the following: 1. Ask the user to input an integer that will be appended to a list that was originally empty. This will be done 5 times, meaning that when the input is complete, the list will have five elements (all integers). a. Determine whether each element is an even number or an odd number b. Return a list of five string elements "odd" and "even" that map the indexes of the elements of the input list, according to whether these elements are even or odd numbers.For example: if the input sequence is 12, 13, 21, 51, and 30, the function will return the list ["even", "odd", "odd", "odd", "even"].arrow_forwardHello, can someone help me with this assignment? I am trying to do it on Python language: Develop a program that first reads in the name of an input file, followed by two strings representing the lower and upper bounds of a search range. The file should be read using the file.readlines() method. The input file contains a list of alphabetical, ten-letter strings, each on a separate line. Your program should output all strings from the list that are within that range (inclusive of the bounds). Ex: If the input is: input1.txt ammoniated millennium and the contents of input1.txt are: aspiration classified federation…arrow_forward
- Please send me answer of this question immediately and i will give you like sure sirarrow_forwardWhen analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This adjustment can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by dividing all values by the largest value. The input begins with an integer indicating the number of floating-point values that follow. Assume that the list will always contain positive floating-point values. Output each floating-point value with two digits after the decimal point, which can be achieved as follows:print(f'{your_value:.2f}')arrow_forwardIn python, Problem Description:You are hosting a party and do not have room to invite all of your friends. You use the following unemotional mathematical method to determine which friends to invite. Number your friends 1, 2, . . . , K and place them in a list in this order. Then perform m rounds. In each round, use a number to determine which friends to remove from the ordered list. The rounds will use numbers r1, r2, . . . , rm. In round i remove all the remaining people in positions that are multiples of ri (that is, ri, 2ri, 3ri, . . .) The beginning of the list is position 1. Output the numbers of the friends that remain after this removal process. Input Specification:The first line of input contains the integer K (1 ≤ K ≤ 100). The second line of input contains the integer m (1 ≤ m ≤ 10), which is the number of rounds of removal. The next m lines each contain one integer. The ith of these lines (1 ≤ i ≤ m) contains ri ( 2 ≤ ri ≤ 100) indicating that every person at a position…arrow_forward
- Could you show me a different way to write this code in python? I’m trying to make the code simple and easy to understandarrow_forwardprogram- python Write a function called “missing”. Ask the user for a series of integers from 1 to N. Send that list to missing and have it return the list of what numbers are missing. For example, if they enter 1 3 7 10, then you would call missing on that list which returns the list of missing numbers and then you can let them know by printing out the list that they are missing 2 4 5 6 8 9. Test it out on this and show it works. PLEASE USE SET SUBTRACTIONarrow_forwardPython programming only NEED HELP PLEASEarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
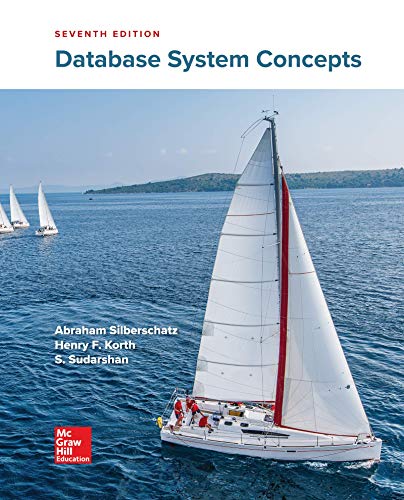
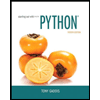
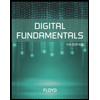
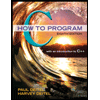
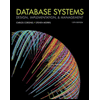
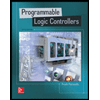