Python Program: Auction House
Chapter10: Introduction To Inheritance
Section: Chapter Questions
Problem 17RQ
Related questions
Question
100%
Python Program: Auction House
![bid_id,bidder_id,auction,merchandise,device, time,country,ip,url
0,8dac2b, ewmzr,jewelry, phone0,9759243157894736,us,69.166.231.58,vasstdc27m7nks3
1,668d39, aeqok , furniture, phone1,9759243157894736,in,50.201.125.84,jmqlhflrzwuay9c
The 1st line is the “header" line, which lists all the fields. The 2nd line shows a bid with bid_id = 0.
The person who made this bid has bidder_id = '8dac2b', and the bid is for the auction 'ewmzr'.
For this subtask, you will implement a function CSV2List(csvFilename: str) -> list[Bid] which
takes as input a file name; and
• returns a list of Bid instances ordered by their bid_id, from small to large.
Keep in mind that the input file may not list the bids in the right bid_id order. You will need to reorder
them.
Hint #1: How does sorted or list.sort interact with your custom-made comparison above?
Hint #2: import csv. It's not fast but it understands CSV.
Subtask III:
In the final subtask, you will implement the following two functions to analyze the bids:
• a function mostPopularAuction(bidList: list[Bid]) -> set[Auction] takes in a list of
Bid instances (for example, as what you would get from CSV2List above) and returns a set of
identifiers (each a string) of the most popular auction(s). The most popular auction is defined as
the auction that has the most distinct number of bidders. There may be multiple auctions with
the same number of bidders.
• a function auctionWinners(bidList: list[Bid]) -> dict[str, Auction] takes in a list
of Bid instances (same as above) and returns a dictionary with the following property:
If d is the resulting dictionary and a is an auction identifier, then d[a] is an Auction
instance that reflects the state of this auction (the auction with identifier a) after going
through all the bids.
Other Things:
• We're testing your program with datasets that contain up to 1 million rows (and 20MB in size).
• On such datasets, we expect each of your functions to run within 15 seconds.
• There will be sample input/output files on the course website.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F48954d8a-c361-4523-b68b-ac12d6ced482%2F4bd197d7-a35a-4c44-875b-8c16a0ed5566%2Fr4tudbt_processed.png&w=3840&q=75)
Transcribed Image Text:bid_id,bidder_id,auction,merchandise,device, time,country,ip,url
0,8dac2b, ewmzr,jewelry, phone0,9759243157894736,us,69.166.231.58,vasstdc27m7nks3
1,668d39, aeqok , furniture, phone1,9759243157894736,in,50.201.125.84,jmqlhflrzwuay9c
The 1st line is the “header" line, which lists all the fields. The 2nd line shows a bid with bid_id = 0.
The person who made this bid has bidder_id = '8dac2b', and the bid is for the auction 'ewmzr'.
For this subtask, you will implement a function CSV2List(csvFilename: str) -> list[Bid] which
takes as input a file name; and
• returns a list of Bid instances ordered by their bid_id, from small to large.
Keep in mind that the input file may not list the bids in the right bid_id order. You will need to reorder
them.
Hint #1: How does sorted or list.sort interact with your custom-made comparison above?
Hint #2: import csv. It's not fast but it understands CSV.
Subtask III:
In the final subtask, you will implement the following two functions to analyze the bids:
• a function mostPopularAuction(bidList: list[Bid]) -> set[Auction] takes in a list of
Bid instances (for example, as what you would get from CSV2List above) and returns a set of
identifiers (each a string) of the most popular auction(s). The most popular auction is defined as
the auction that has the most distinct number of bidders. There may be multiple auctions with
the same number of bidders.
• a function auctionWinners(bidList: list[Bid]) -> dict[str, Auction] takes in a list
of Bid instances (same as above) and returns a dictionary with the following property:
If d is the resulting dictionary and a is an auction identifier, then d[a] is an Auction
instance that reflects the state of this auction (the auction with identifier a) after going
through all the bids.
Other Things:
• We're testing your program with datasets that contain up to 1 million rows (and 20MB in size).
• On such datasets, we expect each of your functions to run within 15 seconds.
• There will be sample input/output files on the course website.
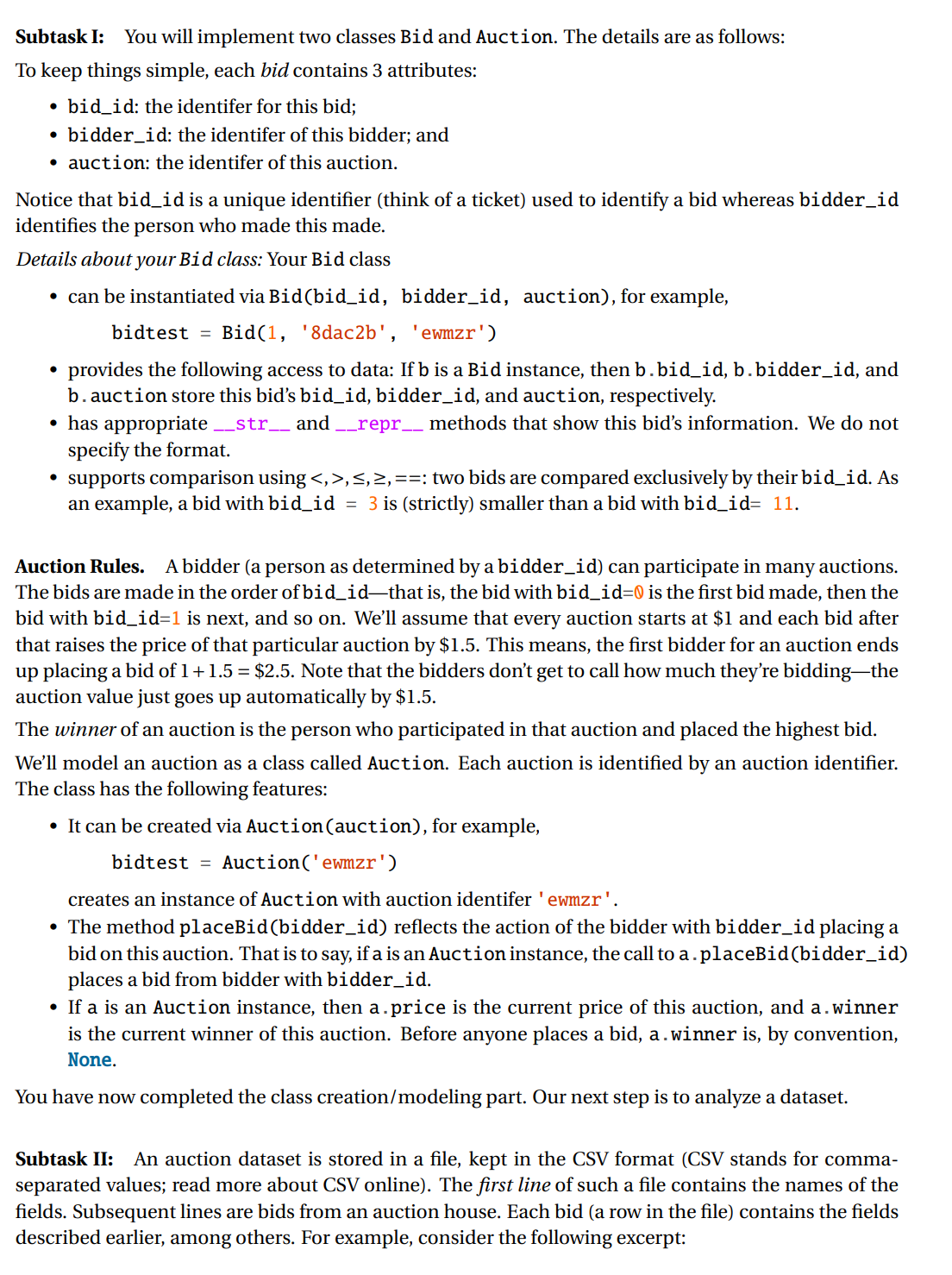
Transcribed Image Text:Subtask I: You will implement two classes Bid and Auction. The details are as follows:
To keep things simple, each bid contains 3 attributes:
bid_id: the identifer for this bid;
• bidder_id: the identifer of this bidder; and
• auction: the identifer of this auction.
Notice that bid_id is a unique identifier (think of a ticket) used to identify a bid whereas bidder_id
identifies the person who made this made.
Details about your Bid class: Your Bid class
• can be instantiated via Bid(bid_id, bidder_id, auction), for example,
bidtest =
Bid(1, '8dac2b',
ewmzr')
• provides the following access to data: If b is a Bid instance, then b.bid_id, b.bidder_id, and
b.auction store this bid's bid_id, bidder_id, and auction, respectively.
has appropriate _str__ and _repr__ methods that show this bid's information. We do not
specify the format.
supports comparison using <,>, s,2,==: two bids are compared exclusively by their bid_id. As
an example, a bid with bid_id = 3 is (strictly) smaller than a bid with bid_id= 11.
Auction Rules. A bidder (a person as determined by a bidder_id) can participate in many auctions.
The bids are made in the order of bid_id–that is, the bid with bid_id=0 is the first bid made, then the
bid with bid_id=1 is next, and so on. We'll assume that every auction starts at $1 and each bid after
that raises the price of that particular auction by $1.5. This means, the first bidder for an auction ends
up placing a bid of 1+1.5 = $2.5. Note that the bidders don't get to call how much they're bidding-the
auction value just goes up automatically by $1.5.
The winner of an auction is the person who participated in that auction and placed the highest bid.
We'll model an auction as a class called Auction. Each auction is identified by an auction identifier.
The class has the following features:
• It can be created via Auction(auction), for example,
bidtest = Auction('ewmzr')
creates an instance of Auction with auction identifer 'ewmzr'.
• The method placeBid(bidder_id) reflects the action of the bidder with bidder_id placing a
bid on this auction. That is to say, if a is an Auction instance, the call to a.placeBid(bidder_id)
places a bid from bidder with bidder_id.
• If a is an Auction instance, then a.price is the current price of this auction, and a.winner
is the current winner of this auction. Before anyone places a bid, a.winner is, by convention,
None.
You have now completed the class creation/modeling part. Our next step is to analyze a dataset.
Subtask II:
An auction dataset is stored
a file, kept in the CSV format (CSV stands for comma-
separated values; read more about CSV online). The first line of such a file contains the names of the
fields. Subsequent lines are bids from an auction house. Each bid (a row in the file) contains the fields
described earlier, among others. For example, consider the following excerpt:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
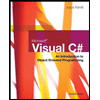
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
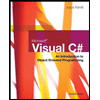
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,