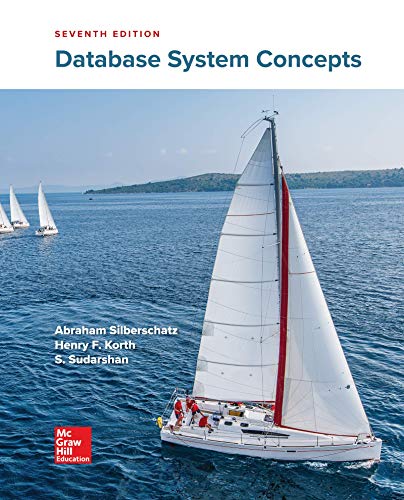
Python:
Write a program that takes an integer list as input and sorts the list into descending order using selection sort. The program should use nested loops and output the list after each iteration of the outer loop, thus outputting the list N-1 times (where N is the size of the list).
Ex: If the input is:
20 10 30 40
the output is:
[40, 10, 30, 20]
[40, 30, 10, 20]
[40, 30, 20, 10]

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 3 images

Using input " 20 10 30 40"
I get this error with your code
Traceback (most recent call last): File "main.py", line 19, in <module> N = int(input("Enter number of elements : ")) ValueError: invalid literal for int() with base 10: '20 10 30 40'
Along with this output :
Enter number of elements :
Using input " 20 10 30 40"
I get this error with your code
Traceback (most recent call last): File "main.py", line 19, in <module> N = int(input("Enter number of elements : ")) ValueError: invalid literal for int() with base 10: '20 10 30 40'
Along with this output :
Enter number of elements :
- 121: Write a Python program that prompts the user to enter 3 numbers, store these numbers in a list, and then calculate the average of the numbers stored in the list. Your average calcualtion cose should work for any number of items in the list (Hint: use the len() method)arrow_forwardPYTHON without def function randomly generate numbers by rolling one die. That is, if your program rolls a die 6000 times, you should get approximately 1000 ones, 1000 twos, etc.? Use a list to count the number of times a die is rolled, given the user wants the die rolled N times. Example Execution #1 Input the number of rolls to make:NUMBER> 6Input the seed for the random number generator:SEED> 0Your 6 rolls follow:OUTPUT 1 occurred 1 timesOUTPUT 2 occurred 0 timesOUTPUT 3 occurred 1 timesOUTPUT 4 occurred 3 timesOUTPUT 5 occurred 1 timesOUTPUT 6 occurred 0 times Example Execution #2 Input the number of rolls to make:NUMBER> 600000Input the seed for the random number generator:SEED> 123456Your 600000 rolls follow:OUTPUT 1 occurred 100340 timesOUTPUT 2 occurred 100016 timesOUTPUT 3 occurred 100186 timesOUTPUT 4 occurred 99931 timesOUTPUT 5 occurred 99858 timesOUTPUT 6 occurred 99669 timesarrow_forwardThe chapter is nested lists Create code in python using for loops Thanks!arrow_forward
- Create a program that generates an eight-digit account number. The program should generate eight random numbers, each in the range of 0 to 9, and assign each number to a list element. Then write another loop that displays the entire contents of the list without the []. use pythonarrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forwardWrite a program that uses 2 lists. Call the lists Items and Basket respectively. The first list will contain a list of at least 10 items (make up your own items) to select from. The second list will be used to add the selected items in the new list.(Basket) Your program should do the following in a Loop until the user exits the loop: Display the list (print out 1, 2, 3, 4, etc. before each item in the list (Hint: Use Index + 1) Prompt the user to select an item from the Items list. Add the selected item to the new list (Basket) (HINT: Use number entered - 1 for the Index) When the user selects 0, exit the loop and print out the new list of items (Basket) Use Microsoft Word to create your Outline and Logic sections. You can copy and paste your Python code at the end under the heading "Code:" Upload your work into the assignment when completedarrow_forward
- IN PYTHON! finish the incomplete program by writing the code that can be placed below the #write the code. use loops to calculate the sums and avg of each row of scores in the list. the following output should be displayed. dont change any part of the code. OUTPUT: Average scores for students: Student # 1: 12.0 Student # 2: 22.0 CODE: students =[ [11, 12, 13], [21, 22, 23] ] avgs = [] # Write your code here: print('Average scores for students: ") for i, avg in enumerate (avgs): print("Student #', i + 1, ':', avg)arrow_forwardPython Smallest and largest numbers in a list Write a program that reads a list of integers into a list as long as the integers are greater than zero, then outputs the smallest and largest integers in the list. Ex: If the input is: 10 5 3 21 2 -6 the output is: 2 and 21 You can assume that the list of integers will have at least 2 values.arrow_forwardin python pleasearrow_forward
- Description: Let’s suppose you are asked to develop a Python program that gets student’s quiz scores in a class, and solve the number of students took the quiz, the quiz total sum, and the quiz average. Write the Python program that performs the following: Get quiz scores and put them in a Python list called, “grades” using a while statement until -1 is entered to end the input; Compute the class average with a for loop; Print the following output: • Total number of students took the quiz; • Quiz total sum; • Quiz average.arrow_forwardDon't use ai to answer I will report your answerarrow_forwardC Programming Language Task: Deviation Write a program that prompts the user to enter N numbers and calculates which of the numbers has the largest deviation from the average of all numbers. You program should first prompt the user to enter how many numbers that will specify. The program should then scan for each number, separated by a newline. You should calculate the average value and return the number from the list which is furthest away from this average (to 2dp). Try using dynamic memory functions to store the incoming array of numbers on the heap. Code to build from: + 1 #include 2 #include 3 4 int main(void) { 5 6} 7 Output Example: deviation.c How many numbers? 5 Enter them: 1.0 2.0 6.0 3.0 4.0 Average: 3.20 Largest deviation from average: 6.00arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
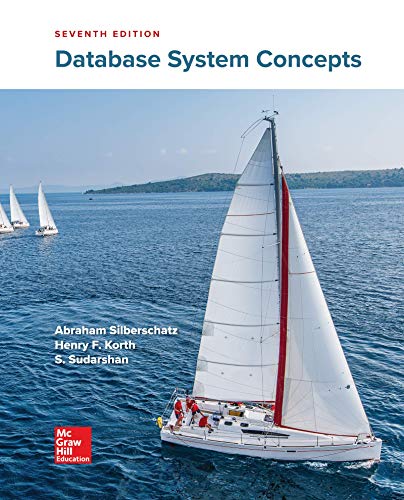
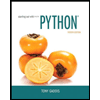
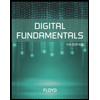
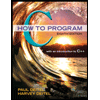
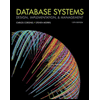
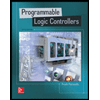