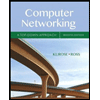
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
![**Exercise Q2.2: Java Programming Task**
**Task Description:**
Write a Java program to print all uppercase alphabets from 'B' to 'Y' using a while loop.
**Instructions:**
1. Start by initializing a character variable to 'B'.
2. Implement a while loop to iterate through the alphabet, ending at 'Y'.
3. Ensure the program correctly prints each letter followed by a space or newline for clarity.
**Code Example:**
```java
public class AlphabetPrinter {
public static void main(String[] args) {
char letter = 'B'; // Initialize starting letter
// While loop to iterate from 'B' to 'Y'
while (letter <= 'Y') {
System.out.print(letter + " "); // Print the current letter
letter++; // Move to the next letter
}
}
}
```
**Explanation:**
- The program begins with the character 'B' and increments using a while loop.
- The `<=` condition ensures that 'Y' is included in the output.
- Use of `System.out.print` for continuous letter output, with each letter followed by a space.
This exercise is an excellent way to practice control structures and the use of character data types in Java.](https://content.bartleby.com/qna-images/question/6d74f304-4963-4d38-b139-fa6445d6d6ae/57833718-e2a2-43e1-a0cf-79b9261d3404/53lumlk_thumbnail.jpeg)
Transcribed Image Text:**Exercise Q2.2: Java Programming Task**
**Task Description:**
Write a Java program to print all uppercase alphabets from 'B' to 'Y' using a while loop.
**Instructions:**
1. Start by initializing a character variable to 'B'.
2. Implement a while loop to iterate through the alphabet, ending at 'Y'.
3. Ensure the program correctly prints each letter followed by a space or newline for clarity.
**Code Example:**
```java
public class AlphabetPrinter {
public static void main(String[] args) {
char letter = 'B'; // Initialize starting letter
// While loop to iterate from 'B' to 'Y'
while (letter <= 'Y') {
System.out.print(letter + " "); // Print the current letter
letter++; // Move to the next letter
}
}
}
```
**Explanation:**
- The program begins with the character 'B' and increments using a while loop.
- The `<=` condition ensures that 'Y' is included in the output.
- Use of `System.out.print` for continuous letter output, with each letter followed by a space.
This exercise is an excellent way to practice control structures and the use of character data types in Java.
Expert Solution

arrow_forward
Requirement:
Write a JAVA program to print all uppercase alphabets from 'B' to 'Y' using a while loop.
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- PLEASE DONT COPY FROM OTHER BARTLEBY POSTS. EVERYTIME I POST YA ALWAYS COPY. DO UR OWN CODE NEED CODE FOR JAVE...please paste ur indented code... use decision statements, loop statements in ur code and keep it simplearrow_forwardIn Java Integer in is read from input. Write a while loop that iterates until in is less than or equal to 0. In each iteration: Update in with the quotient of in and 7. Output the updated in, followed by a newline. Click here for exampleEx: If the input is 2401, then the output is: 343 49 7 1 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 import java.util.Scanner; public class QuotientCalculator { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intin; in=scnr.nextInt(); /your code here/ } }arrow_forwardBb1. 3. Write an algorithm in pseudocode for the procedure to input two numbers (X, Y), and add 3 to the smaller number. Output both numbers.arrow_forward
- Q2. Write a program that reads the value of a positive integer n and prints the total number of squares in an n*n chessboard.arrow_forwardMake a program that prints the table of contents of a book where each chapter has the same number of sections, and each section has the same number of subsections. Example: Please enter the number of chapters 3Please enter the number of sections 3Please enter the number of subsections 3Chapter 1-Section 1.1--Subsection 1.1.1--Subsection 1.1.2--Subsection 1.1.3-Section 1.2--Subsection 1.2.1--Subsection 1.2.2--Subsection 1.2.3-Section 1.3--Subsection 1.3.1--Subsection 1.3.2--Subsection 1.3.3Chapter 2-Section 2.1--Subsection 2.1.1--Subsection 2.1.2--Subsection 2.1.3-Section 2.2--Subsection 2.2.1--Subsection 2.2.2--Subsection 2.2.3-Section 2.3--Subsection 2.3.1--Subsection 2.3.2--Subsection 2.3.3Chapter 3-Section 3.1--Subsection 3.1.1--Subsection 3.1.2--Subsection 3.1.3-Section 3.2--Subsection 3.2.1--Subsection 3.2.2--Subsection 3.2.3-Section 3.3--Subsection 3.3.1--Subsection 3.3.2--Subsection 3.3.3arrow_forward8. Write a JAVA program to read input a string s from the user and find the sum of all the digits in the string s.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
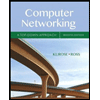
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
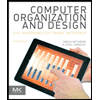
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
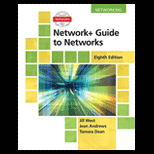
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
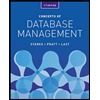
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
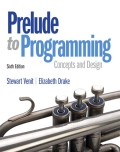
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
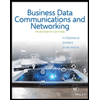
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY