revise and show the output import java.util.Scanner; class Person { //declaring instance variables private String name; private String contactNum; //setters and getters public void setName(String n) { this.name = n; } public String getName() { return name; } public void setContactNum(String c) { this.contactNum = c; } public String getContactNum() { return contactNum; } } class Employee extends Person { //declaring instance variables private double salary; private String department; //setters and getters public void setSalary(double s) { this.salary = s; } public double getSalary() { return salary; } public void setDepartment(String d) { this.department = d; } public String getDepartment() { return department; } } class Faculty extends Employee { //declaring instance variable private boolean status; //method implementation public boolean isRegular() { return status; } } class Student extends Person { //declaring instance variables private String program; private int yearLevel; //setters and getters public void setProgram(String p) { this.program = p; } public String getProgram() { return program; } public void setYearLevel(int y) { this.yearLevel = y; } public int getYearLevel() { return yearLevel; } } public class CollegeList { public static void main(String[] args) { Scanner sc=new Scanner(System.in); System.out.print("Press E for Employee, F for Faulty, S for Student: "); String choice=sc.nextLine(); //input choice if(choice.equalsIgnoreCase("E")) { //if choice is E/e System.out.println("Type employee's name, contact number, salary and department"); System.out.println("Press Enter after every input"); //read values from user String name=sc.nextLine(); String contactNum=sc.nextLine(); double salary=Double.parseDouble(sc.nextLine()); String department=sc.nextLine(); Employee e=new Employee(); //set values e.setName(name); e.setContactNum(contactNum); e.setSalary(salary); e.setDepartment(department); //print all values System.out.println("--------------------------"); System.out.println("Name: "+e.getName()); System.out.println("Contact Number: "+e.getContactNum()); System.out.println("Salary: "+e.getSalary()); System.out.println("Department: "+e.getDepartment()); }else if(choice.equalsIgnoreCase("F")) { //if choice is F/f System.out.println("Type faculty' name, contact number, salary and department"); System.out.println("Press Enter after every input"); //read values from user String name=sc.nextLine(); String contactNum=sc.nextLine(); double salary=Double.parseDouble(sc.nextLine()); String department=sc.nextLine(); System.out.print("Enter Y if regular or N for Tenured: "); boolean status=sc.nextLine().equals("Y"); Faculty f=new Faculty(); //set values f.setName(name); f.setContactNum(contactNum); f.setSalary(salary); f.setDepartment(department); //print all values System.out.println("--------------------------"); System.out.println("Name: "+f.getName()); System.out.println("Contact: "+f.getContactNum()); System.out.println("Salary: "+f.getSalary()); System.out.println("Department: "+f.getDepartment()); System.out.println("Status: "+status); }else if(choice.equalsIgnoreCase("S")) { //if choice is S/s System.out.println("Type student's name, contact number, program and year level"); System.out.println("Press Enter after every input"); //read input values String name=sc.nextLine(); String contactNum=sc.nextLine(); String program=sc.nextLine(); int yearLevel=sc.nextInt(); Student s=new Student(); //set all values s.setName(name); s.setContactNum(contactNum); s.setProgram(program); s.setYearLevel(yearLevel); //print all values System.out.println("--------------------------"); System.out.println("Name: "+s.getName()); System.out.println("Contact Number: "+s.getContactNum()); System.out.println("Program: "+s.getProgram()); System.out.println("Year: "+s.getYearLevel()); }else System.out.println("Invalid Choice"); } }
revise and show the output
import java.util.Scanner;
class Person {
//declaring instance variables
private String name;
private String contactNum;
//setters and getters
public void setName(String n) {
this.name = n;
}
public String getName() {
return name;
}
public void setContactNum(String c) {
this.contactNum = c;
}
public String getContactNum() {
return contactNum;
}
}
class Employee extends Person {
//declaring instance variables
private double salary;
private String department;
//setters and getters
public void setSalary(double s) {
this.salary = s;
}
public double getSalary() {
return salary;
}
public void setDepartment(String d) {
this.department = d;
}
public String getDepartment() {
return department;
}
}
class Faculty extends Employee {
//declaring instance variable
private boolean status;
//method implementation
public boolean isRegular() {
return status;
}
}
class Student extends Person {
//declaring instance variables
private String
private int yearLevel;
//setters and getters
public void setProgram(String p) {
this.program = p;
}
public String getProgram() {
return program;
}
public void setYearLevel(int y) {
this.yearLevel = y;
}
public int getYearLevel() {
return yearLevel;
}
}
public class CollegeList {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
System.out.print("Press E for Employee, F for Faulty, S for Student: ");
String choice=sc.nextLine(); //input choice
if(choice.equalsIgnoreCase("E")) { //if choice is E/e
System.out.println("Type employee's name, contact number, salary and department");
System.out.println("Press Enter after every input");
//read values from user
String name=sc.nextLine();
String contactNum=sc.nextLine();
double salary=Double.parseDouble(sc.nextLine());
String department=sc.nextLine();
Employee e=new Employee();
//set values
e.setName(name);
e.setContactNum(contactNum);
e.setSalary(salary);
e.setDepartment(department);
//print all values
System.out.println("--------------------------");
System.out.println("Name: "+e.getName());
System.out.println("Contact Number: "+e.getContactNum());
System.out.println("Salary: "+e.getSalary());
System.out.println("Department: "+e.getDepartment());
}else if(choice.equalsIgnoreCase("F")) { //if choice is F/f
System.out.println("Type faculty' name, contact number, salary and department");
System.out.println("Press Enter after every input");
//read values from user
String name=sc.nextLine();
String contactNum=sc.nextLine();
double salary=Double.parseDouble(sc.nextLine());
String department=sc.nextLine();
System.out.print("Enter Y if regular or N for Tenured: ");
boolean status=sc.nextLine().equals("Y");
Faculty f=new Faculty();
//set values
f.setName(name);
f.setContactNum(contactNum);
f.setSalary(salary);
f.setDepartment(department);
//print all values
System.out.println("--------------------------");
System.out.println("Name: "+f.getName());
System.out.println("Contact: "+f.getContactNum());
System.out.println("Salary: "+f.getSalary());
System.out.println("Department: "+f.getDepartment());
System.out.println("Status: "+status);
}else if(choice.equalsIgnoreCase("S")) { //if choice is S/s
System.out.println("Type student's name, contact number, program and year level");
System.out.println("Press Enter after every input");
//read input values
String name=sc.nextLine();
String contactNum=sc.nextLine();
String program=sc.nextLine();
int yearLevel=sc.nextInt();
Student s=new Student();
//set all values
s.setName(name);
s.setContactNum(contactNum);
s.setProgram(program);
s.setYearLevel(yearLevel);
//print all values
System.out.println("--------------------------");
System.out.println("Name: "+s.getName());
System.out.println("Contact Number: "+s.getContactNum());
System.out.println("Program: "+s.getProgram());
System.out.println("Year: "+s.getYearLevel());
}else
System.out.println("Invalid Choice");
}
}
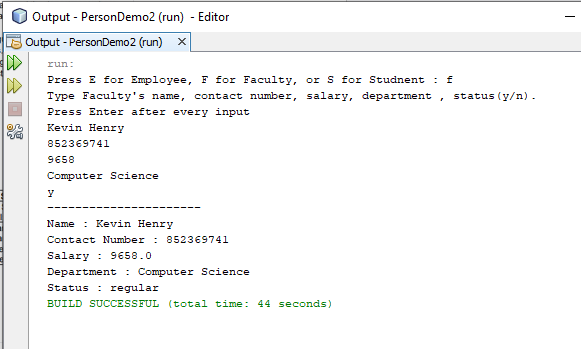

Step by step
Solved in 2 steps with 1 images

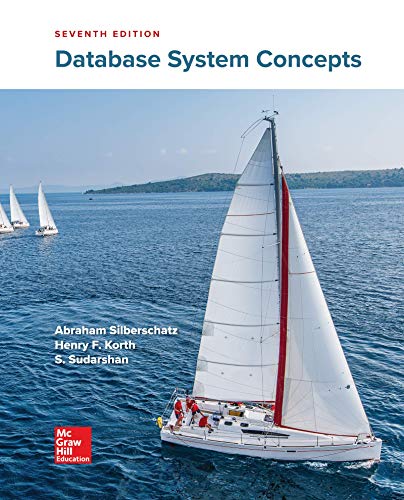
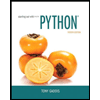
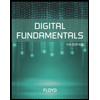
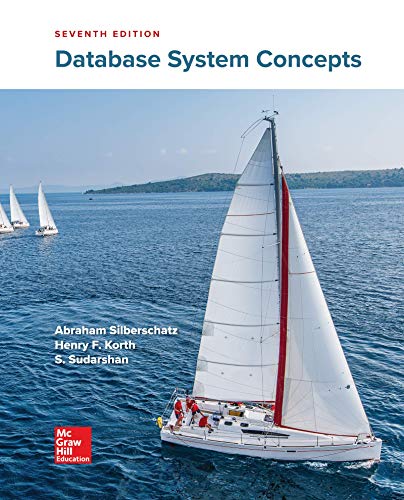
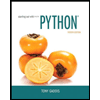
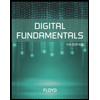
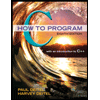
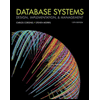
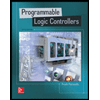