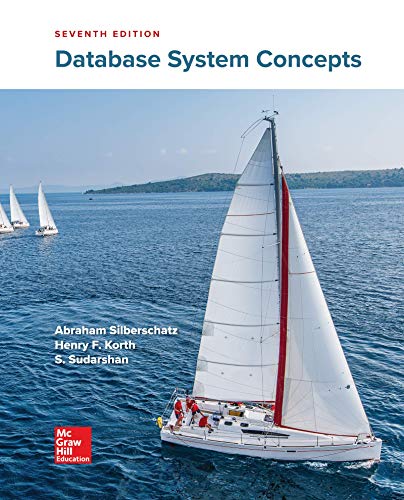
Add the method below to the parking office class.
Method
- park(Date, ParkingPermit, ParkingLot) : ParkingTransaction
Parkingoffice.java
public class ParkingOffice {
String name;
String address;
String phone;
List<Customer> customers;
List<Car> cars;
List<ParkingLot> lots;
List<ParkingCharge> charges;
public ParkingOffice(){
customers = new ArrayList<>();
cars = new ArrayList<>();
lots = new ArrayList<>();
charges = new ArrayList<>();
}
public Customer register() {
Customer cust = new Customer(name,address,phone);
customers.add(cust);
return cust;
}
public Car register(Customer c,String licence, CarType t) {
Car car = new Car(c,licence,t);
cars.add(car);
return car;
}
public Customer getCustomer(String name) {
for(Customer cust : customers)
if(cust.getName().equals(name))
return cust;
return null;
}
public double addCharge(ParkingCharge p) {
charges.add(p);
return p.amount;
}
public String[] getCustomerIds(){
String[] stringArray1 = new String[2];
for(int i=0;i<2;i++){
stringArray1[i] = customers.get(i).customerId;
}
return stringArray1;
}
public String [] getPermitID () {
String[] stringArray2 = new String[1];
for(int i=0;i<1;i++){
stringArray2[i] = charges.get(i).permitID;
}
return stringArray2;
}
public List<String> getPermitIds(Customer c){
List<String> permitid = new ArrayList<String>();
for(Car car:cars){
if(car.owner == c.customerId)
permitid.add(car.permit);
}
return permitid;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- toString() and equals() Methods This lab will demonstrate how the toString() and equals() methods work when they are not overridden. Type up the code and submit the BOTH results. CODE public class ObjectToString( public static void main(String[] args) { ClassOne co new ClassOne(); ClassTwo ct = new ClassTwo(); } } public class Classone { System.out.println(co); System.out.println(ct); System.out.println(co.equals(ct)); public void printfle() { System.out.println("I am from ClassOne"); } public class ClassTwo ( public void printMe() { } System.out.println("I am from ClassTwo"); Run the program and notice the output.arrow_forwardLook at the following class declarations: class Plant { public virtual void Message() { MessageBox.Show("I'm a plant."); } } class Tree : Plant { public override void Message() { MessageBox.Show("I'm a tree."); } } Given these class definitions, what will the following code display? Plant p = new Tree(); p.Message();arrow_forwardTask 1: Write an abstract class Method +: public -: private #: protected Underline: static # input: int # output:String Method + isHard():boolean + specificWay(): String + Method() + Method (input: int, output: String) + getInput(): int + setInput(input: int): void + getOutput(): String + setOutput(output: String): void + toString(): String Page < 38 of 41 с ZOOM + ■arrow_forward
- Shape -width: double -length: double +allmutatorMethods Shapes2D Shapes3D -height:double *calSize():double +calVolume():double 1. Define class Shape, 2DShape and 3DShape as per UML class diagram given. 2. Define all mutator methods(set and get) for all classes. 3. Define methods calArea() with formula area - width * length for 2DShapes. 4. Define methods calArea() with formula calvolume, volume = width*length*height for 3DShapes. In the main program, declare and set data in 1 object of 3DShapes. Create an array OR ArrayList of 2DShapes, set and display the info of all objects as shown in the output. 5.arrow_forward... in javaarrow_forwardTRUE OR FALSE A method that uses a generic class parameter can be static or dynamic.arrow_forward
- Take the following code: class Song { private: string name; string author; string genre; int year_released; public: Song() { /* initialize attributes */} string get_name() { return name; } void set_name(string new_name) { name = new_name; } string get_author() { return author; } void set_author(string new_author) { author = new_author; } int get_age(int curr_year) { return curr_year - year_released; } void set_year_released(int new_year) { year_released = new_year ; } }; indicate the setters of this class. Edit View Insert Format Tools Table 12pt v Paragraph v B IUAarrow_forwardThere is a class hierarchy that includes three classes: class Animal { protected int age; public int getAge() { return age; } public void setAge(int age) { this.age = age; }}class Pet extends Animal { protected String name; public String getName() { return name; } public void setName(String name) { this.name = name; }}class Cat extends Pet { protected String color; public String getColor() { return color; } public void setColor(String color) { this.color = color; }} Given the following object: Pet cat = new Cat(); Select all invalid method invocations.arrow_forwardclass box { int width; int height; int length; int volume; void volume(int height, int length, int width) { volume = width*height*length; } } class Prameterized_method { public static void main(String args[]) { box obj = new box(); obj.height = 1; obj.length = 5; obj.width = 5; obj.volume(3,2,1); System.out.println(obj.volume); } } Give result for the code Java Try to do ASAParrow_forward
- class Product: def __init__(self, product_id, name, price, quantity): self.product_id = product_id self.name = name self.price = round(price, 2) self.quantity = quantity def get_product_description(self): return (f"Product ID: {self.product_id}, " f"Name: {self.name}, " f"Price: ${self.price:.2f}, " f"Quantity Available: {self.quantity}") class ElectronicProduct(Product): def __init__(self, product_id, name, price, quantity,warranty_period): self.warranty_period = warranty_period super().__init__(product_id,name,price,quantity) def get_product_description(self): base_description = super().get_product_description() return base_description + f"\nWarranty: {self.warranty_period}" class ClothingProduct(Product): def __init__(self, product_id, name, price, quantity,size): self.size = size…arrow_forwardGiven the following class definition: class employee{public: employee(); employee(string, int, double); employee(int, double); employee(string); void setData(string, int, double); void print() const; void updatePay(double); int getNumOfServiceYears() const; double getPay() const;private: string name; int numOfServiceYears; double pay;}; Create a class parttime derived from the above class that includes private members payRate and hoursWorked of type double along with a constructor and the definition of a print function that prints out all information from the base and derived class. Provide a test program that would demonstrate the working parttime class. Note: You can assume all code is stored in a single file.arrow_forwardsolution in python languagearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
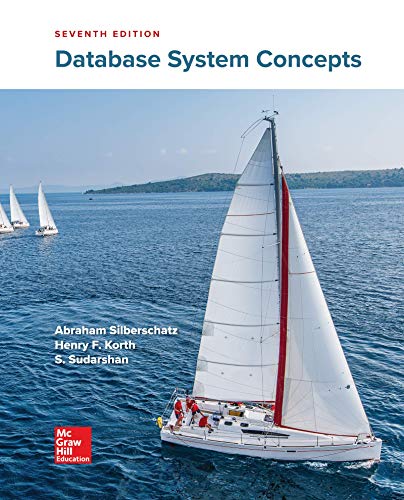
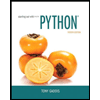
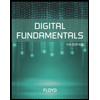
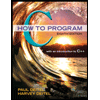
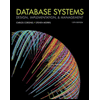
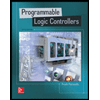